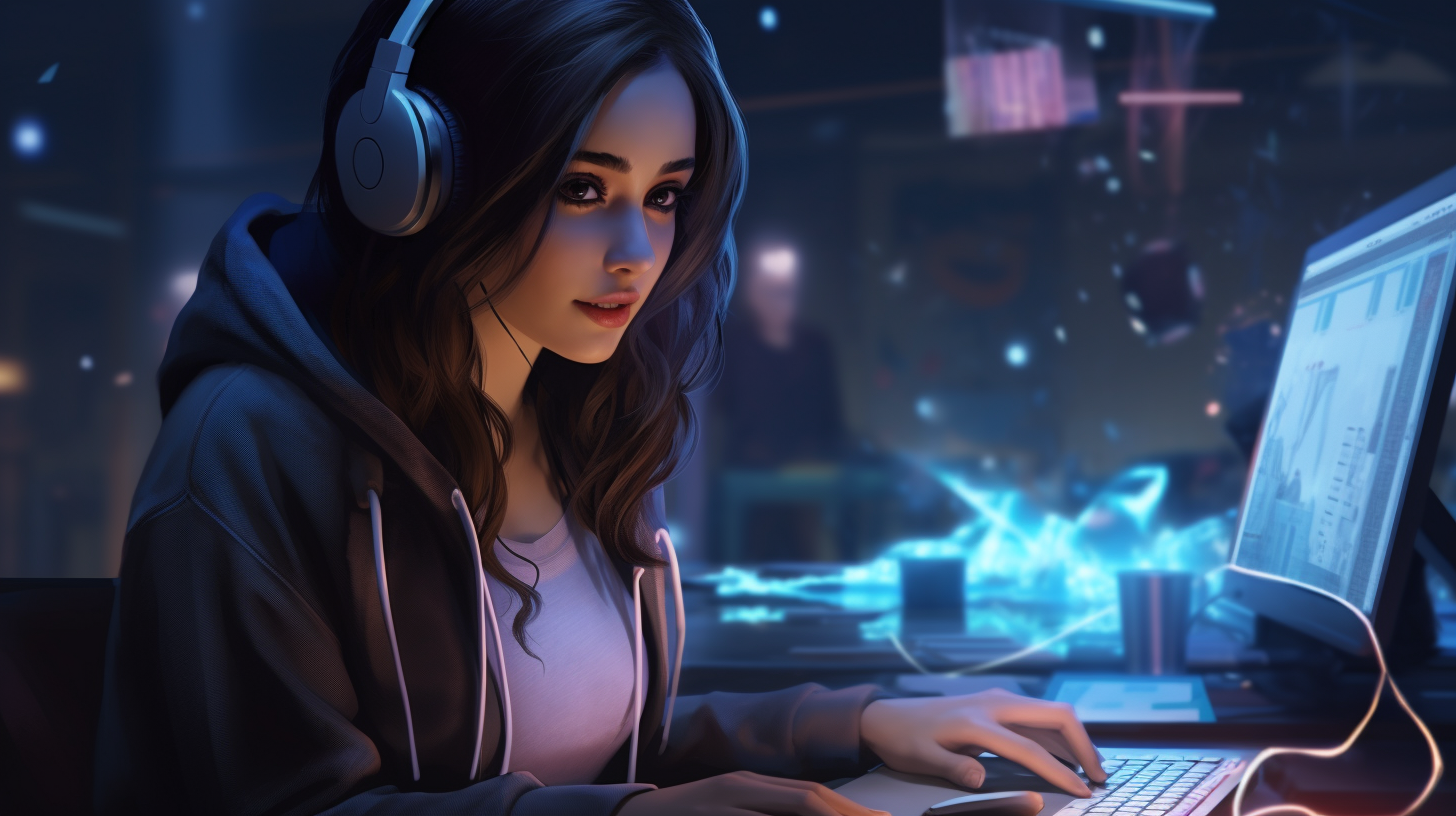
When it comes to analyzing data, understanding the relationships between data sets is critical. SQL, or Structured Query Language, is a powerful tool used to manage and manipulate relational databases. In this article, we’ll explore how to use SQL for data relationship analysis.
Data Relationships
Before diving into the SQL code, it’s important to understand the types of relationships that can exist between data sets:
- One-to-One: Each record in one table corresponds to one record in another table.
- One-to-Many: A single record in one table can relate to one or more records in another table.
- Many-to-Many: Records in one table can relate to multiple records in another table, and vice versa.
These relationships are established through the use of keys – primary keys that uniquely identify a record in a table, and foreign keys that reference the primary key in another table.
SQL Joins
Joins are a key concept in SQL when analyzing data relationships. Joins allow you to combine rows from two or more tables based on related columns. There are several types of joins:
- INNER JOIN: Returns records with matching values in both tables.
- LEFT (OUTER) JOIN: Returns all records from the left table, and the matched records from the right table.
- RIGHT (OUTER) JOIN: Returns all records from the right table, and the matched records from the left table.
- FULL (OUTER) JOIN: Returns all records when there is a match in either left or right table.
Code Example: INNER JOIN
SELECT employees.name, departments.name FROM employees INNER JOIN departments ON employees.department_id = departments.id;
This code will retrieve a list of employee names along with their department names by joining the ’employees’ table with the ‘departments’ table using the department_id as the common key.
Code Example: LEFT JOIN
SELECT employees.name, departments.name FROM employees LEFT JOIN departments ON employees.department_id = departments.id;
This code will retrieve all employees’ names and their department names if available. If an employee doesn’t belong to any department, the department name will be NULL.
Aggregate Functions for Data Analysis
When analyzing data relationships, aggregate functions can be used to compute summary statistics. Some common aggregate functions include COUNT(), SUM(), AVG(), MAX(), and MIN().
Code Example: COUNT()
SELECT departments.name, COUNT(employees.id) as number_of_employees FROM departments LEFT JOIN employees ON departments.id = employees.department_id GROUP BY departments.name;
This code will return the number of employees in each department by grouping the results based on the department name and counting the employee IDs.
Subqueries for Complex Analysis
Sometimes, you may need to perform complex analyses that require subqueries. A subquery is a SQL query nested inside a larger query.
Code Example: Subquery
SELECT name FROM employees WHERE department_id IN (SELECT id FROM departments WHERE name = 'Engineering');
This code will list all employees who are in the ‘Engineering’ department, by using a subquery that first selects all department IDs with the name ‘Engineering’.
To wrap it up, SQL provides a high number of methods for analyzing data relationships, from simple joins to intricate subqueries. Understanding these concepts and applying them effectively allows for powerful and meaningful insights from your data sets.
Source: https://www.plcourses.com/sql-for-data-relationship-analysis/