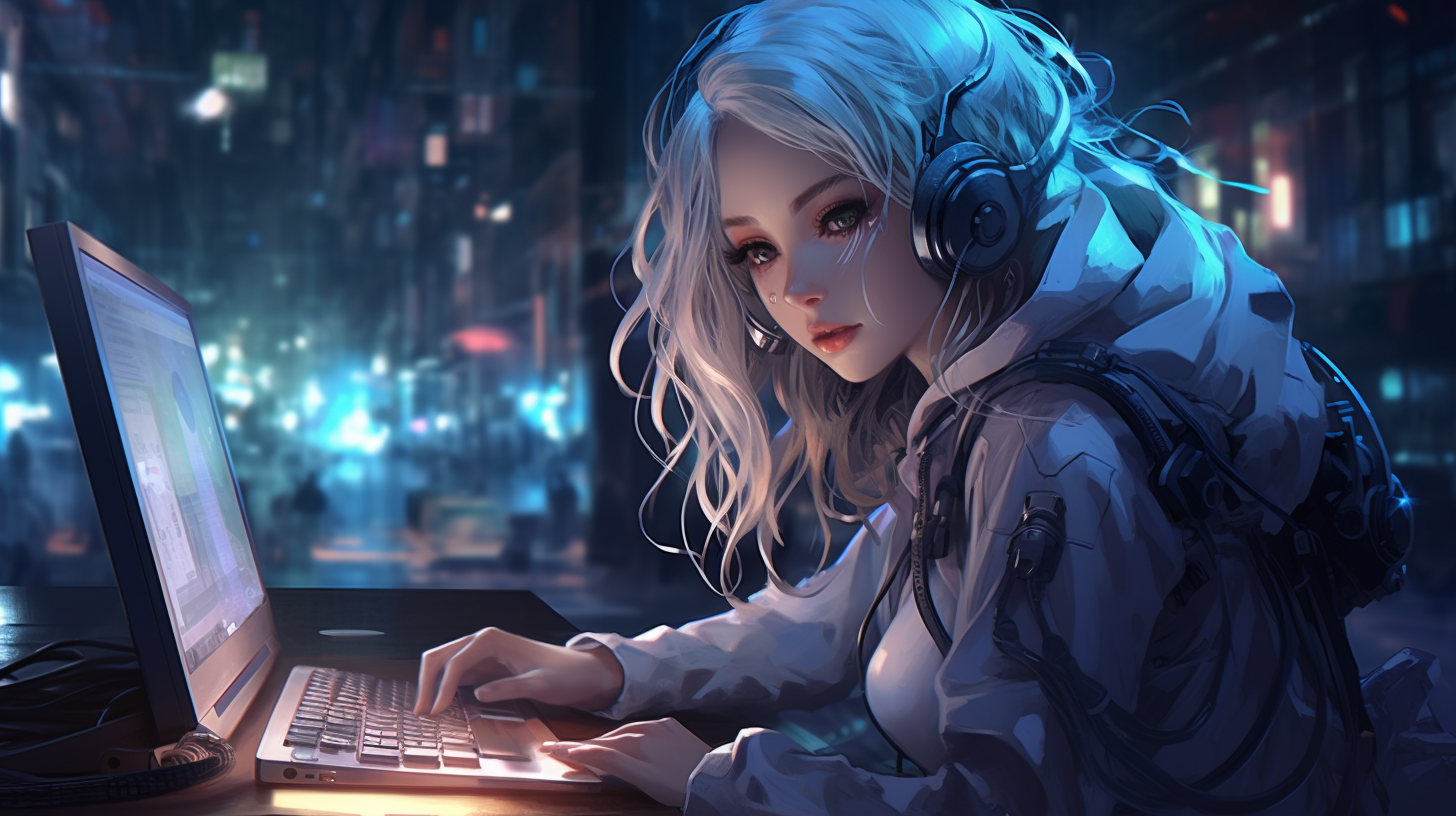
In recent years, there has been a growing interest in electric vehicles (EVs) as a sustainable and eco-friendly mode of transportation. As EV adoption continues to increase, there is a need for data analysis and simulation techniques to optimize the performance and efficiency of these vehicles.
Python, with its rich ecosystem of libraries and tools, offers a powerful platform for analyzing and simulating data related to electric vehicles. In this tutorial, we will explore how to use Python for data analysis and simulation in the context of electric vehicles.
Data Analysis with Pandas
Pandas is a popular Python library for data manipulation and analysis. It provides powerful data structures and data analysis tools, making it an ideal choice for working with electric vehicle data.
To get started, we first need to install the Pandas library. Open your terminal or command prompt and run the following command:
pip install pandas
Once Pandas is installed, we can import it into our Python script and start using its functionalities:
import pandas as pd # Create a dataframe from a CSV file df = pd.read_csv('electric_vehicle_data.csv') # Display the first few rows of the dataframe print(df.head())
In the above example, we import Pandas as ‘pd’ for convenience. We then use the ‘read_csv()’ function to create a dataframe from a CSV file containing electric vehicle data. We can then use the ‘head()’ function to display the first few rows of the dataframe.
Simulation with SimPy
SimPy is a discrete-event simulation library for Python. It allows us to model and simulate complex systems, such as electric vehicle charging stations, in a flexible and efficient manner.
To install SimPy, run the following command:
pip install simpy
Let’s create a simple simulation of an electric vehicle charging station using SimPy:
import simpy def charging_station(env): while True: print(f"Charging at time {env.now}") yield env.timeout(1) # Charging takes 1 unit of time env = simpy.Environment() env.process(charging_station(env)) # Run the simulation for 5 units of time env.run(until=5)
In the above example, we define a function called ‘charging_station’ that simulates the behavior of an electric vehicle charging station. The function runs indefinitely and prints the current time when a vehicle is charging. We then create a SimPy environment, add the ‘charging_station’ process to the environment, and run the simulation for 5 units of time.
This is just a basic example, but SimPy allows for much more complex simulations, including modeling different charging strategies, vehicle arrivals, and resource constraints.
Python provides powerful tools for data analysis and simulation in the context of electric vehicles. In this tutorial, we explored how to use Pandas for data analysis and SimPy for simulation. With these tools, you can analyze and optimize the performance of electric vehicles, as well as simulate different scenarios to improve charging infrastructure and efficiency.
Whether you are a researcher, engineer, or enthusiast in the field of electric vehicles, Python can be a valuable asset in your data analysis and simulation tasks. Start exploring the possibilities today!
Source: https://www.plcourses.com/python-for-electric-vehicles-data-analysis-and-simulation/