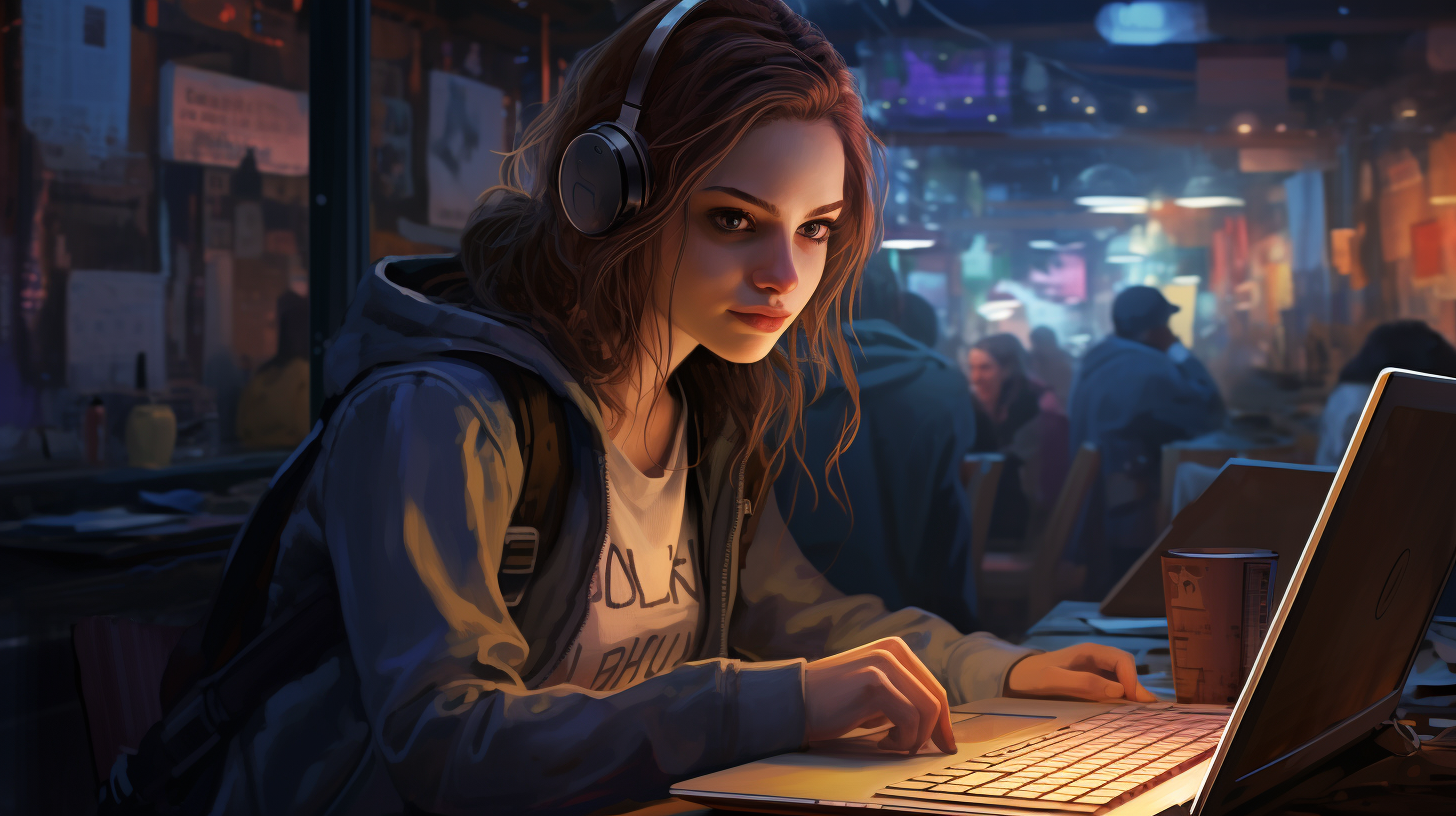
Bash, short for Bourne Again SHell, is a powerful scripting language commonly used in the Unix operating system. While it is primarily known for its use in automating system administration tasks, Bash can also be utilized in web development, specifically for making HTTP requests and interacting with APIs.
In this tutorial, we will explore the various ways you can leverage Bash for web development. We will cover topics such as sending HTTP requests, parsing JSON responses, handling authentication, and interacting with RESTful APIs.
Sending HTTP Requests
One of the fundamental tasks in web development is sending HTTP requests. With Bash, you can easily make GET, POST, PUT, DELETE, and other types of requests using the curl
command-line tool.
# Sending a GET request curl http://example.com/api/users # Sending a POST request with JSON payload curl -X POST -H "Content-Type: application/json" -d '{"username":"john","password":"secret"}' http://example.com/api/login
Here, we use the curl
command followed by the target URL to send a GET request. For POST requests, we specify the method using -X POST
and include the JSON payload using -d
.
Parsing JSON Responses
When interacting with APIs, responses are often delivered in JSON format. Extracting data from JSON responses is a common task in web development. In Bash, you can utilize tools like jq
to parse and manipulate JSON data.
curl http://example.com/api/users | jq '.[0].username'
In this example, we make a GET request to retrieve a list of users from an API. We then pipe the response to jq
and extract the username of the first user using the JSONPath-like syntax '.[0].username'
.
Handling Authentication
Many web APIs require authentication to access protected resources. Bash provides various methods to handle authentication, including basic authentication and OAuth.
For basic authentication, you can include the credentials in the request using the -u
flag with curl
:
curl -u username:password http://example.com/api/protected-resource
If the API uses OAuth, you can obtain an access token using Bash and pass it as a header:
# Request an access token response=$(curl -X POST -d "client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&grant_type=client_credentials" https://api.example.com/oauth/token) access_token=$(echo $response | jq -r '.access_token') # Make a request with the access token curl -H "Authorization: Bearer $access_token" http://example.com/api/protected-resource
In this example, we first obtain an access token by making a POST request to the OAuth server. We then extract the token from the response using jq
. Finally, we include the access token in the Authorization header of subsequent requests via -H "Authorization: Bearer $access_token"
.
Interacting with RESTful APIs
RESTful APIs are widely used for web development. With Bash, you can easily interact with RESTful APIs by constructing HTTP requests and handling responses. Let’s look at an example of creating a new resource.
# Creating a new user curl -X POST -H "Content-Type: application/json" -d '{"username":"jane","password":"secretpassword"}' http://example.com/api/users
In this example, we make a POST request to the API endpoint for creating users. We include the user details in the JSON payload and set the Content-Type
header to application/json
.
Bash is not typically associated with web development, but it can be a handy tool for making HTTP requests, interacting with APIs, and handling JSON responses. By leveraging tools like curl
and jq
, you can perform various web development tasks directly from the command line.
Whether you need to retrieve data from an API, create new resources, or handle authentication, Bash has you covered. So next time you find yourself needing to perform web development tasks, give Bash a try!
Source: https://www.plcourses.com/bash-for-web-development/