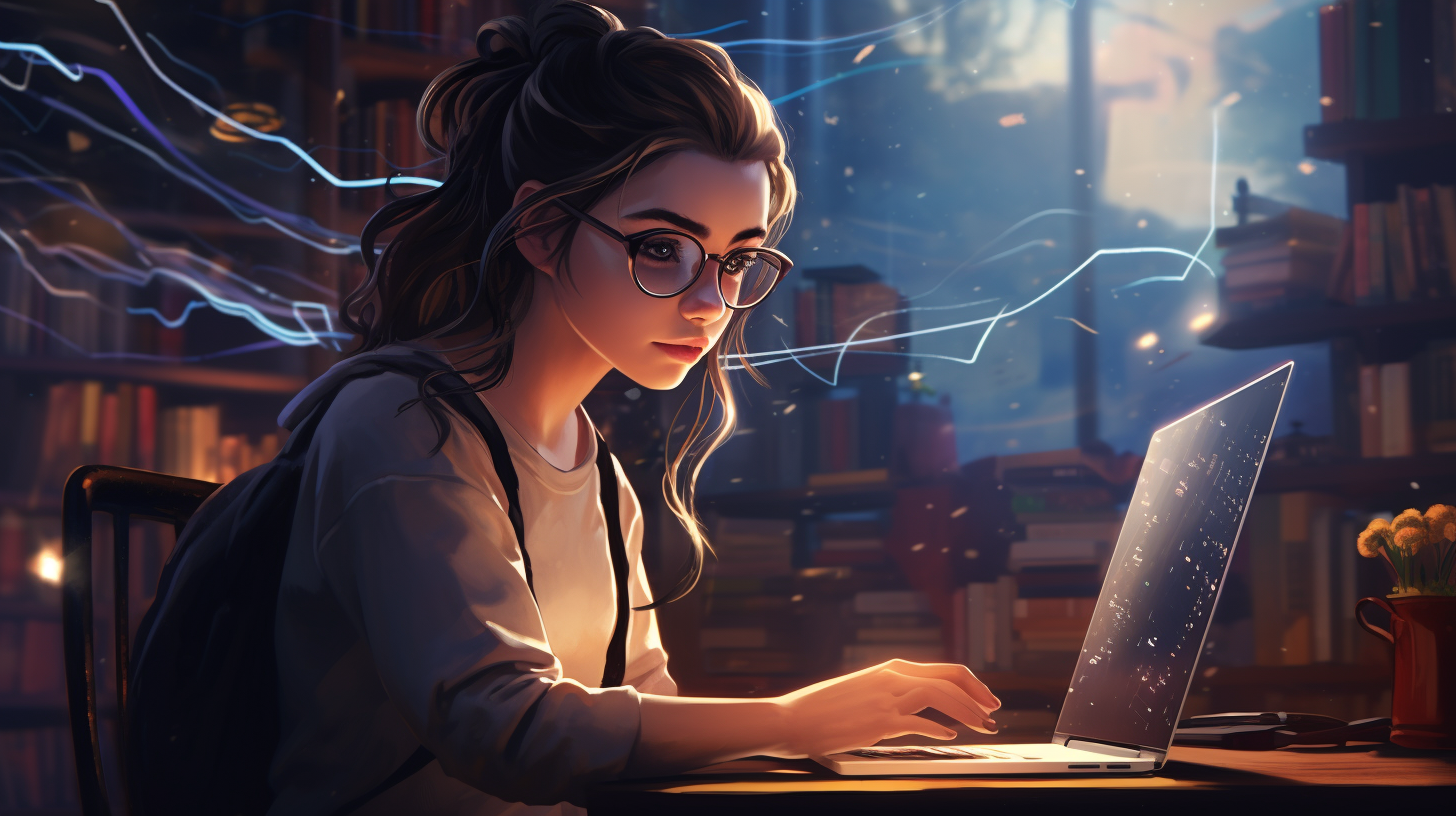
When dealing with sensitive data in Bash scripts, understanding the fundamentals of encryption is essential. Encryption is the process of transforming information to make it unreadable to unauthorized users. Essentially, it converts plaintext into ciphertext through the use of algorithms and keys. To begin grasping encryption, it’s crucial to comprehend a few key concepts: plaintext, ciphertext, keys, algorithms, and the types of encryption.
Plaintext is the original data that you want to protect, whereas ciphertext refers to the scrambled version of the data produced after encryption. The transformation from plaintext to ciphertext is executed using an encryption algorithm, which is a systematic method that dictates how the data should be altered.
Another pivotal component is the key. This is a piece of information that determines the output of the encryption algorithm. Without the key, decrypting the ciphertext back to plaintext becomes practically impossible. This leads us to the two main types of encryption:
Symmetric encryption employs the same key for both encryption and decryption. It is generally faster and more efficient for encrypting large amounts of data. However, it requires secure key management, as anyone with the key can decrypt the data. Common symmetric algorithms include AES (Advanced Encryption Standard) and DES (Data Encryption Standard).
Asymmetric encryption, on the other hand, uses a pair of keys: a public key for encryption and a private key for decryption. This method is considerably more secure since the private key does not need to be shared. RSA (Rivest-Shamir-Adleman) is a well-known asymmetric encryption algorithm.
Now that you have a foundational understanding of encryption, let’s look at how you can implement these concepts in your Bash scripts.
# Example of using OpenSSL for symmetric encryption # Encrypting a file openssl enc -aes-256-cbc -salt -in myfile.txt -out myfile.txt.enc -k mypassword # Decrypting the file openssl enc -d -aes-256-cbc -in myfile.txt.enc -out myfile.txt -k mypassword
In the example above, we use the OpenSSL command-line tool to perform symmetric encryption using the AES-256-CBC algorithm. Notice how the same password is used for both encryption and decryption, showcasing the core principle of symmetric cryptography.
Understanding these basic elements of encryption not only helps you secure data effectively but also lays the groundwork for more complex data protection strategies in your scripts.
Choosing the Right Encryption Tools
Choosing the right encryption tools is critical to ensuring the security of your data in Bash scripts. The landscape of encryption tools is vast, with a variety of options available, each with its strengths and weaknesses. When selecting an encryption tool, you should consider factors such as ease of use, compatibility, performance, and your specific encryption needs.
One of the most widely used tools for encryption is OpenSSL. This robust library and command-line utility supports a variety of cryptographic algorithms and protocols. It is highly versatile, so that you can perform symmetric and asymmetric encryption, hashing, and secure communication over networks. Here’s a quick example of how to use OpenSSL to securely encrypt files:
# Encrypting a file openssl enc -aes-256-cbc -salt -in myfile.txt -out myfile.txt.enc -k mypassword # Decrypting the file openssl enc -d -aes-256-cbc -in myfile.txt.enc -out myfile.txt -k mypassword
In the example above, we specify the AES-256-CBC algorithm for encryption, using a salt for added security. The -k
option allows you to set the password directly in the command. However, be cautious: hardcoding passwords in scripts is generally discouraged due to security risks.
Another tool worth mentioning is GnuPG (GPG), which provides a robust framework for public-key cryptography. GPG supports both symmetric and asymmetric encryption, allowing for secure file encryption and signing. It’s particularly useful when you need to share encrypted data with others without the need to exchange keys directly. Here’s how you can use GPG to encrypt and decrypt files:
# Encrypt a file with a symmetric key gpg -c myfile.txt # Decrypt the file gpg myfile.txt.gpg
Using the -c
flag prompts GPG to use symmetric encryption, where you will be asked to enter a passphrase. This approach is more secure than using a simple command-line password since GPG hashes the passphrase.
When choosing an encryption tool, ponder the performance implications as well. Some algorithms may be faster than others, especially with larger datasets. Symmetric encryption algorithms like AES are typically faster than their asymmetric counterparts like RSA. However, asymmetric encryption provides benefits in terms of key management and security, especially when sharing encrypted information.
Ultimately, the choice of encryption tool depends on your specific use case. For simple, simpler file encryption, OpenSSL or GPG may suffice. For more complex requirements involving secure key exchanges, you might want to ponder integrating a complete library like those provided by GnuPG or APIs that wrap these functionalities for easier use within your scripts.
Always remember that the security of your keys and the encryption process is as strong as the weakest link in your system. Ensure that key storage and management are handled with the utmost care to maintain the integrity of your encrypted data.
Implementing Encryption in Bash Scripts
Once you’ve selected the right tools, the next step is to implement encryption within your Bash scripts effectively. This process involves integrating the encryption commands into your scripts while ensuring that they are executed securely and reliably. Below are practical methods for implementing both symmetric and asymmetric encryption in your Bash scripts.
Implementing Symmetric Encryption
Using OpenSSL for symmetric encryption is a common choice due to its flexibility and robust features. Here’s a basic implementation that demonstrates how to encrypt and decrypt a file within a Bash script:
#!/bin/bash # Define variables PASSWORD="mypassword" INPUT_FILE="myfile.txt" ENCRYPTED_FILE="myfile.txt.enc" DECRYPTED_FILE="myfile_decrypted.txt" # Encrypt the file openssl enc -aes-256-cbc -salt -in "$INPUT_FILE" -out "$ENCRYPTED_FILE" -k "$PASSWORD" # Notify the user echo "File encrypted: $ENCRYPTED_FILE" # Decrypt the file openssl enc -d -aes-256-cbc -in "$ENCRYPTED_FILE" -out "$DECRYPTED_FILE" -k "$PASSWORD" # Notify the user echo "File decrypted: $DECRYPTED_FILE"
In the above script, we define the password and filenames as variables for ease of modification. OpenSSL encrypts the file with AES-256-CBC, storing the output in a specified encrypted file. The script also includes commands to decrypt the file, showcasing the dual functionality of the script.
Implementing Asymmetric Encryption
For situations where secure key management is required, asymmetric encryption using GnuPG (GPG) is an excellent option. Below is a script that demonstrates how to create a key pair, encrypt a file using the public key, and then decrypt it using the private key:
#!/bin/bash # Generate GPG key pair (run this once) # gpg --full-generate-key # Define variables INPUT_FILE="myfile.txt" ENCRYPTED_FILE="myfile.txt.gpg" DECRYPTED_FILE="myfile_decrypted.txt" # Encrypt the file gpg --output "$ENCRYPTED_FILE" --encrypt --recipient "[email protected]" "$INPUT_FILE" # Notify the user echo "File encrypted with GPG: $ENCRYPTED_FILE" # Decrypt the file gpg --output "$DECRYPTED_FILE" --decrypt "$ENCRYPTED_FILE" # Notify the user echo "File decrypted: $DECRYPTED_FILE"
This script showcases the process of encrypting a file with GPG using a public key associated with the specified recipient. The decryption process then leverages the private key, ensuring that only the intended recipient can access the plaintext data. This method enhances security, particularly when sharing sensitive information over insecure channels.
Handling Errors and Secure Practices
When implementing encryption in your Bash scripts, it’s vital to handle potential errors gracefully. Ensure your scripts check for the success of each command, especially during file operations. Additionally, consider the following practices:
- Use environment variables for sensitive information instead of hardcoding passwords or keys directly in the script.
- Implement logging to keep track of encryption and decryption operations for auditing purposes.
- Regularly update your encryption libraries to protect against vulnerabilities.
- Consider using a secure temporary directory for working with sensitive files to minimize exposure.
Integrating these encryption methods and practices in your Bash scripts will significantly enhance the security of your data. Remember, the focus should always be on maintaining the integrity and confidentiality of the information you’re handling.
Best Practices for Secure Data Handling
When it comes to secure data handling within your Bash scripts, a disciplined approach especially important. The notion of security transcends mere encryption; it encompasses all facets of data management, from how you store your keys to how you handle sensitive data in transit. Below are essential practices to follow that will fortify your scripts against potential vulnerabilities.
1. Secure Key Management
Proper management of encryption keys is one of the cornerstones of data security. Hardcoding sensitive keys and passwords into your scripts is a risky practice that exposes you to a high number of threats. Instead, consider using environment variables or external secure storage solutions, such as HashiCorp Vault or AWS Secrets Manager, to retrieve your keys dynamically at runtime. This method obfuscates your sensitive information and mitigates the risk of accidental exposure.
# Retrieve the encryption key from an environment variable PASSWORD=${ENCRYPTION_PASSWORD}
2. Use Temporary Files Judiciously
When working with sensitive data, always use temporary files with care. If a script generates temporary files during encryption and decryption, ensure they’re stored securely and deleted after use. Use the mktemp
command to create a unique temporary file, which helps prevent race conditions and ensures that your scripts do not inadvertently use an existing file.
# Create a secure temporary file for the decrypted content TEMP_FILE=$(mktemp) /usr/bin/openssl enc -d -aes-256-cbc -in "$ENCRYPTED_FILE" -out "$TEMP_FILE" -k "$PASSWORD" # Process the temporary file and delete it afterward rm "$TEMP_FILE"
3. Logging and Auditing
Implementing logging is an often-overlooked component of secure data handling. For every encryption or decryption operation performed in your scripts, log the necessary details (without exposing sensitive data, of course). This practice allows you to audit actions taken on sensitive files and can be invaluable for tracing back any issues that arise.
# Logging example echo "$(date): Encrypted $INPUT_FILE to $ENCRYPTED_FILE" >> /var/log/encryption.log echo "$(date): Decrypted $ENCRYPTED_FILE to $DECRYPTED_FILE" >> /var/log/decryption.log
4. Regularly Update Encryption Libraries
Like any software, encryption libraries are subject to vulnerabilities. Regularly updating OpenSSL, GnuPG, and any other cryptographic libraries you use is essential to protect against known exploits. Automate the update process if possible, and monitor announcements for critical patches.
5. Validate Input and Output
Data validation especially important when dealing with inputs for encryption processes. Ensure that the files you intend to encrypt meet your expected criteria to avoid injecting harmful data into your scripts. Similarly, validate the output after decryption to confirm that it matches the original plaintext.
# Check if the input file exists before processing if [[ ! -f "$INPUT_FILE" ]]; then echo "Error: Input file $INPUT_FILE does not exist." >&2 exit 1 fi
By adhering to these best practices, you ensure that your Bash scripts are not only effective in encrypting data but also resilient against various security threats. Taking proactive steps in secure data handling will significantly fortify the integrity and confidentiality of the information you manage.
Source: https://www.plcourses.com/encrypting-data-in-bash-scripts/