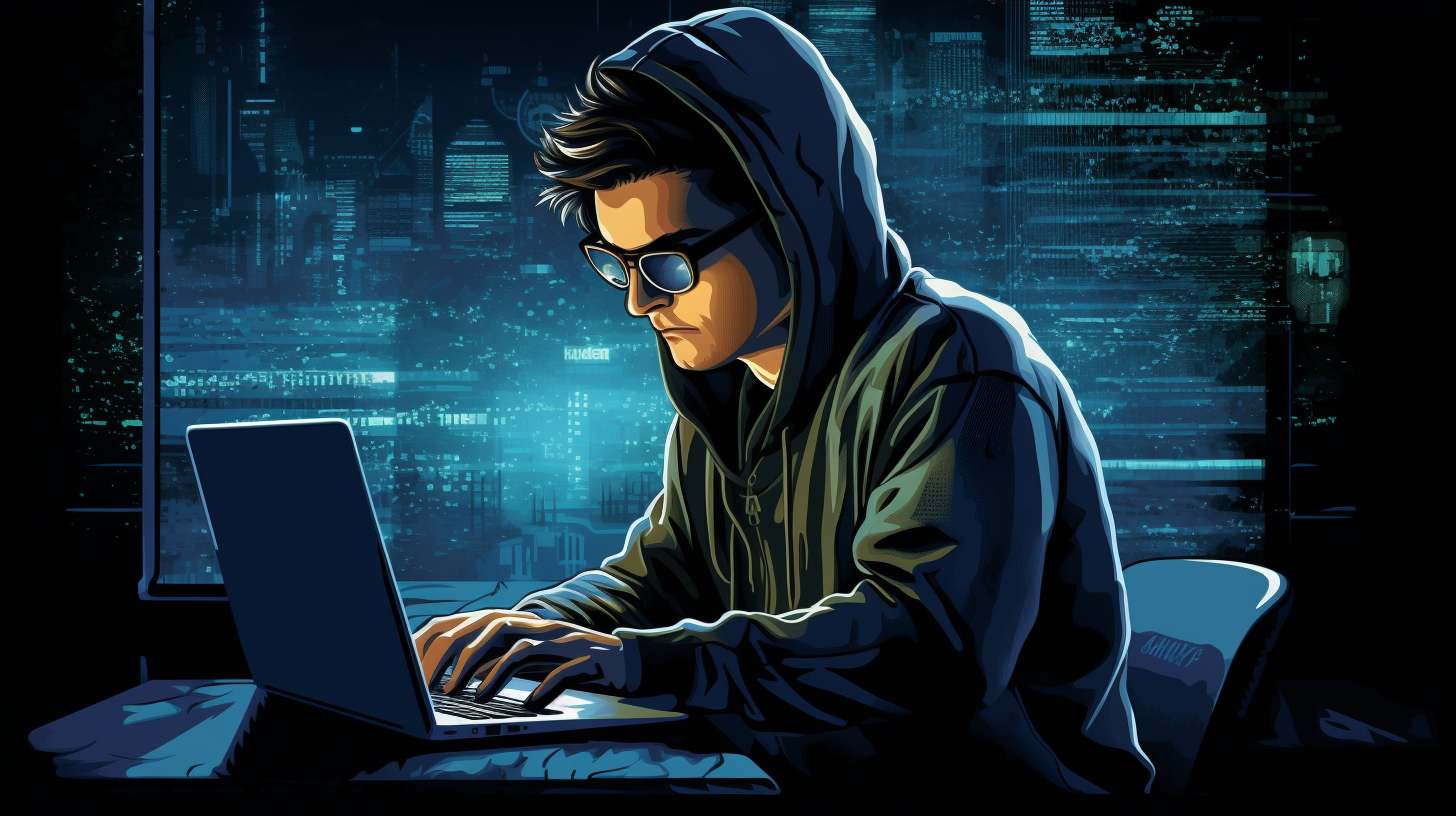
Core Image is a powerful framework provided by Apple that allows for sophisticated image processing and analysis. It serves as the backbone for manipulating images in various ways, making it a vital component for developers working with graphics in Swift. The framework harnesses the GPU (Graphics Processing Unit) for real-time image processing, which results in smooth performance and high-quality output.
When using Core Image, developers interface with a series of classes and methods designed to handle image filtering, transformation, and analysis. Core Image operates on the concept of a CIImage object, which represents image data in a format that the framework can manipulate. This allows for streamlined operations on images, ranging from simple adjustments like brightness and contrast to more complex filters that can apply artistic effects.
One of the key advantages of using Core Image is its non-destructive editing capabilities. By applying filters to a CIImage, developers can preview changes in real time without altering the original image data. This feature is critical for applications that require iterative adjustments or user-driven modifications.
To create a CIImage
, developers typically initialize it from a UIImage
or directly from a file URL. Here’s a simple example of how to create a CIImage
from a UIImage
:
let uiImage = UIImage(named: "exampleImage")! let ciImage = CIImage(image: uiImage)
Once you have a CIImage, you can apply various filters to it using CIFilter. This filter class provides a plethora of built-in filters that can be used to alter the appearance of the image. Each filter can be configured with various parameters to achieve the desired effect.
For instance, if you wanted to apply a Gaussian blur to the image, you could use the following code snippet:
let blurFilter = CIFilter(name: "CIGaussianBlur") blurFilter?.setValue(ciImage, forKey: kCIInputImageKey) blurFilter?.setValue(10.0, forKey: kCIInputRadiusKey) if let outputImage = blurFilter?.outputImage { // Process outputImage as needed }
As you dig deeper into Core Image, you’ll find that it supports a wide range of functionality, including the ability to chain multiple filters and create custom filters if more specialized effects are needed. This flexibility makes Core Image an essential tool for anyone looking to implement high-performance image processing in their Swift applications.
Setting Up Your Swift Environment for CoreImage
Before diving into the intricacies of Core Image, it is crucial to ensure that your Swift environment is properly set up to leverage this powerful framework. Core Image is part of the larger iOS and macOS SDKs, and integrating it into your projects is quite simpler.
First, you need to make sure that you are using Xcode, Apple’s integrated development environment (IDE) for macOS. Xcode provides all the necessary tools to develop, build, and test your Swift applications. Ensure you have the latest version of Xcode installed, as updates frequently include enhancements and new features for frameworks like Core Image.
Once Xcode is ready, you can start a new project or open an existing one. To use Core Image, you need to import the framework at the beginning of your Swift files. That is done using a simple import statement:
import CoreImage
After importing the framework, you can begin working with Core Image classes such as CIImage, CIFilter, and CIContext. Create your image processing pipeline by ensuring that you understand how to initialize a CIImage from different sources, such as UIImage, URLs, or raw pixel data.
As you work on the image processing tasks, ensure you also have the necessary permissions to access the photo library or camera if your application requires it. That’s important for apps that need to manipulate images taken by users. You can configure these permissions in the project’s Info.plist file, where you specify the usage description keys like NSPhotoLibraryUsageDescription and NSCameraUsageDescription.
For a practical example, let’s say you want to load an image from the app’s bundle and process it with Core Image. Here’s how you might set that up:
if let imagePath = Bundle.main.path(forResource: "exampleImage", ofType: "jpg") { let imageURL = URL(fileURLWithPath: imagePath) let ciImage = CIImage(contentsOf: imageURL) // Now you can apply filters to ciImage }
After loading the image, you can use various filters to manipulate it as needed. It is also beneficial to explore the Core Image documentation provided by Apple to understand the vast array of filters and their parameters. This will help you broaden the scope of what you can achieve with Core Image in your applications.
Essential CoreImage Classes and Their Functions
Core Image provides an extensive collection of classes and functions that streamline image processing tasks. Understanding these essential classes is important for using the full potential of the framework. The primary classes you will interact with include CIImage, CIFilter, and CIContext, each serving a distinct role in the image processing pipeline.
The CIImage class is the foundation of all image operations in Core Image. This class represents an image in a format that Core Image can manipulate, encapsulating the image data you wish to process. You can create a CIImage from various sources: a UIImage, a file URL, or even raw pixel data. This versatility allows developers to work with images from diverse origins effectively.
let uiImage = UIImage(named: "exampleImage")! let ciImage = CIImage(image: uiImage)
Once you have a CIImage, you can implement image transformations using the CIFilter class. This class serves as a bridge to a wide array of built-in filters that can be applied to the CIImage. Each filter can be adjusted through input parameters to achieve a desired visual effect. Filters are very flexible and allow for chaining multiple effects together to create complex visual transformations.
For example, to apply a sepia tone effect to an image, the following code snippet utilizes the CIFilter class:
let sepiaFilter = CIFilter(name: "CISepiaTone")! sepiaFilter.setValue(ciImage, forKey: kCIInputImageKey) sepiaFilter.setValue(0.8, forKey: kCIInputIntensityKey) if let outputImage = sepiaFilter.outputImage { // Use outputImage as needed }
Another critical class is CIContext. This class manages the rendering of CIImages into other formats, such as CGImage or Bitmap data. It serves as an interface between Core Image and the underlying graphics system. When you have applied filters to your CIImage and are ready to display or save it, you would use a CIContext to render the output.
Here’s how you can create a CIContext and render an output image:
let context = CIContext() if let outputImage = sepiaFilter.outputImage, let cgImage = context.createCGImage(outputImage, from: outputImage.extent) { let processedImage = UIImage(cgImage: cgImage) // Use processedImage for display or further processing }
In addition to these core classes, Core Image also offers a range of utility classes for handling specific tasks, such as CIImageAccumulator for accumulating image data over multiple frames and CIImageOptions for configuring various rendering options. By using these classes, developers can create sophisticated image processing workflows that are both efficient and effective.
Image Filters: Transforming Visuals with CoreImage
Image filters are at the heart of Core Image, providing developers with a powerful way to alter and enhance visuals with minimal effort. With over a hundred built-in filters available, Core Image allows you to apply a wide range of effects, from standard adjustments like brightness and contrast to more artistic transformations such as sepia tones and vintage effects. This section delves into the mechanics of applying these filters, the parameters involved, and how to tailor them to suit your specific application needs.
To apply an image filter using Core Image, you start by creating a CIFilter instance for the desired effect. Each filter comes with its own set of input parameters that allow you to control the effect’s intensity, radius, or other characteristics. Once the filter is configured, you can retrieve the output image which represents the result of applying that filter to your original CIImage.
Here’s an example that demonstrates how to apply a vibrance filter to enhance the colors of an image:
let vibranceFilter = CIFilter(name: "CIVibrance")! vibranceFilter.setValue(ciImage, forKey: kCIInputImageKey) vibranceFilter.setValue(1.0, forKey: kCIInputAmountKey) if let outputImage = vibranceFilter.outputImage { // This outputImage now has enhanced vibrance }
In this example, we create a vibrance filter, set the input image, and specify the amount of vibrance to apply. The filter enhances the saturation of the less saturated colors while leaving the already vibrant colors untouched, resulting in a more balanced and appealing image.
Chaining multiple filters is another powerful feature of Core Image. You can take the output of one filter and use it as the input for another, allowing for complex transformations. For instance, you can combine a Gaussian blur with a color adjustment to create a dreamy effect:
let blurFilter = CIFilter(name: "CIGaussianBlur")! blurFilter.setValue(ciImage, forKey: kCIInputImageKey) blurFilter.setValue(5.0, forKey: kCIInputRadiusKey) if let blurredImage = blurFilter.outputImage { let colorAdjustmentFilter = CIFilter(name: "CIColorControls")! colorAdjustmentFilter.setValue(blurredImage, forKey: kCIInputImageKey) colorAdjustmentFilter.setValue(1.2, forKey: kCIInputSaturationKey) if let finalImage = colorAdjustmentFilter.outputImage { // Work with finalImage as needed } }
This example first applies a Gaussian blur to soften the image, and then a color control filter adjusts the saturation of the blurred image, creating a unique artistic output.
For more advanced use cases, you may want to create custom filters. Core Image allows developers to implement their own CIFilter subclasses, enabling bespoke effects that aren’t achievable with the built-in filters. This level of customization opens up a world of creative possibilities, so that you can define new behaviors and effects for your images.
Performance Optimization Techniques for CoreImage in Swift
When implementing Core Image in your Swift applications, performance optimization becomes paramount, especially when dealing with high-resolution images or complex filter chains. Understanding how to effectively manage resources, leverage GPU acceleration, and minimize latency can significantly enhance the responsiveness of your app. Here are several techniques to ensure that your image processing tasks run smoothly and efficiently.
1. Use CIContext Wisely
Creating a CIContext can be resource-intensive. It is beneficial to instantiate a single CIContext and reuse it throughout your application, rather than creating a new one for every image processing task. This approach reduces overhead and maximizes performance. Here’s an example of how to create and reuse a CIContext:
let sharedContext = CIContext() // Create once for reuse
By reusing the same context, you can avoid the repetitive costs of context initialization and help your app maintain a smoother performance.
2. Use Asynchronous Processing
Core Image supports asynchronous processing, which can help avoid blocking the main thread. By using the performAsync
method, you can offload image processing tasks, allowing the UI to remain responsive while the image filters are applied. Here’s how you can implement this:
let queue = DispatchQueue.global(qos: .userInitiated) queue.async { let outputImage = blurFilter.outputImage // Process image on a background thread DispatchQueue.main.async { // Update UI with outputImage on the main thread } }
This technique significantly enhances user experience by ensuring that image processing does not interfere with UI interactions.
3. Optimize Image Size and Format
Working with very large images can lead to unnecessary memory usage and slowdowns. Before applying filters, consider resizing your images to a more manageable resolution. Additionally, using the appropriate format can also save processing time. For instance, converting your images to a more efficient format like JPEG or PNG before processing can reduce the data size:
if let ciImage = CIImage(image: uiImage.resized(to: CGSize(width: 1000, height: 1000))) { // Apply filters here }
Implementing a resizing function can be done easily, ensuring that you’re always working with images that are optimal for your use case.
4. Leverage Filter Caching
Core Image has built-in mechanisms for caching filter outputs. If you are applying the same filters to the same images repeatedly, ponder caching the output images. This can save time and processing power:
var cachedImages: [String: CIImage] = [:] // Cache dictionary if let cachedImage = cachedImages["exampleKey"] { // Use cachedImage instead of processing again } else { let outputImage = blurFilter.outputImage cachedImages["exampleKey"] = outputImage // Cache for future use }
This caching strategy is especially useful in scenarios where users might apply the same filters multiple times, such as in photo editing applications.
5. Profile and Analyze Performance
Finally, always profile your image processing code to identify bottlenecks. Use the Instruments tool provided by Xcode to analyze performance while tracking memory usage, CPU load, and rendering times. This data can guide you in making informed decisions about where optimizations are needed.
Real-World Applications: Using CoreImage for Stunning Effects
Core Image opens the door to a myriad of real-world applications that can enhance user experiences through stunning visual effects. From photo editing applications to augmented reality experiences, Core Image’s powerful capabilities allow developers to create engaging graphics that captivate users. Below are some practical implementations of Core Image that showcase its versatility and effectiveness in delivering complex visual manipulations.
One of the most popular applications of Core Image is in photo editing apps. Users often seek to improve their images with filters that improve aesthetic allure, convey emotions, or simply create a more artistic representation. By using built-in filters, developers can provide users with a suite of options for transforming their photos conveniently. For example, a simple photo editor might include filters for adjusting brightness, contrast, and applying artistic effects like vignette or monochrome.
let contrastFilter = CIFilter(name: "CIContrast")! contrastFilter.setValue(ciImage, forKey: kCIInputImageKey) contrastFilter.setValue(1.5, forKey: kCIInputContrastKey) // Increase contrast if let contrastImage = contrastFilter.outputImage { // Display or process contrastImage }
Another compelling use case for Core Image is in real-time photo effects, particularly for applications involving live camera feeds, such as social media or video conferencing apps. By applying filters directly to the camera feed, developers can allow users to see the effects of their selections in real-time. This interaction especially important for enhancing user engagement and satisfaction.
let cameraFilter = CIFilter(name: "CIPhotoEffectNoir")! // Noir effect for dramatic effect cameraFilter.setValue(ciImage, forKey: kCIInputImageKey) if let effectImage = cameraFilter.outputImage { // Render effectImage onto the camera feed }
Furthermore, Core Image can be harnessed to implement dynamic UI effects that respond to users’ interactions. For instance, when a user taps a button or swipes across the screen, the images can morph or change according to the applied filters, creating a sense of fluidity and responsiveness. Such enhancements can significantly elevate the user experience, especially in gaming or creative applications.
let zoomFilter = CIFilter(name: "CIBumpDistortion")! zoomFilter.setValue(ciImage, forKey: kCIInputImageKey) zoomFilter.setValue(CIVector(x: 150, y: 150), forKey: kCIInputCenterKey) // Center of distortion zoomFilter.setValue(150, forKey: kCIInputRadiusKey) // Radius of the effect if let zoomedImage = zoomFilter.outputImage { // Use zoomedImage as a dynamic visual effect }
Another innovative application of Core Image is in the sphere of augmented reality (AR). Developers can use Core Image to manipulate images captured by the camera, integrating seamlessly with ARKit to overlay effects or transformations that enhance the user’s environment. For example, adding artistic filters to virtual objects or ensuring that images blend into the real world with the right lighting and shadow effects can create a more immersive experience.
let filter = CIFilter(name: "CIEdges")! // Edge detection filter filter.setValue(ciImage, forKey: kCIInputImageKey) if let edgeImage = filter.outputImage { // Integrate edgeImage into AR experience }
Source: https://www.plcourses.com/swift-and-coreimage/