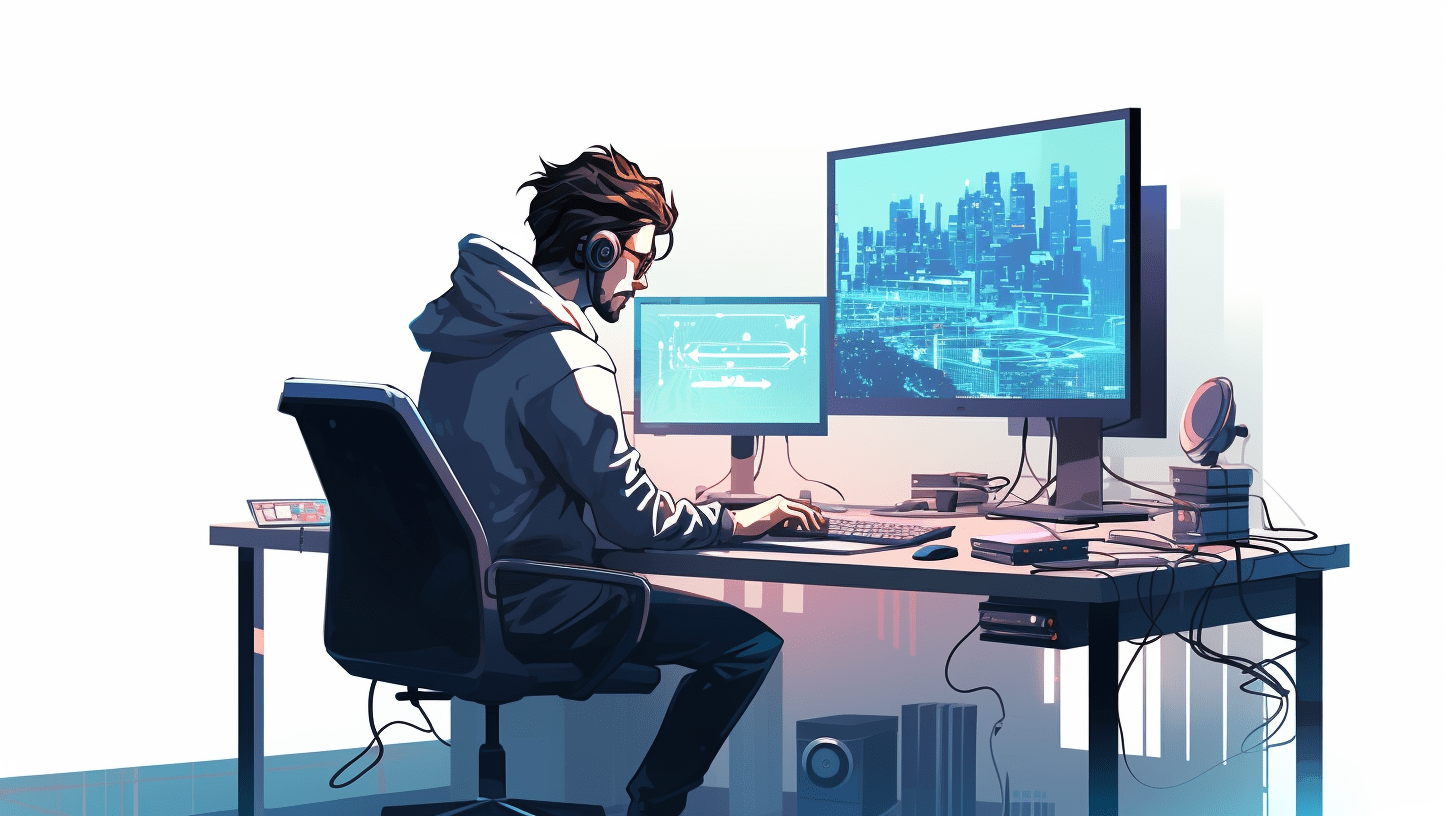
Python is a powerful programming language that is widely used for handling large datasets in the world of big data. With its simplicity and extensive libraries, Python provides various tools and techniques to manipulate and analyze massive amounts of data efficiently.
When it comes to handling big data, two popular frameworks often come to mind: Hadoop and Spark. Hadoop provides a distributed file system called Hadoop Distributed File System (HDFS) and a programming model called MapReduce, while Spark offers a fast and general-purpose cluster computing system that supports advanced analytics and real-time processing.
In this tutorial, we will explore how to handle large datasets using Python in the context of Hadoop and Spark. We will cover different aspects such as reading, processing, and analyzing big data using Python with specific focus on Hadoop and Spark.
1. Handling Large Datasets with Hadoop
To handle large datasets in Hadoop using Python, we need the help of a Python library called Pydoop. Pydoop provides a Python API for Hadoop, allowing us to interact with HDFS and perform MapReduce jobs using Python.
First, we need to ensure that Pydoop is installed in our Python environment. We can install it using pip:
pip install pydoop
Once Pydoop is installed, we can start handling large datasets in Hadoop using Python. Here’s an example of how to read a file from HDFS:
import pydoop.hdfs as hdfs # Open the file in HDFS with hdfs.open('/path/to/file.txt') as file: # Read the contents of the file contents = file.read() # Process the contents of the file # ...
In this example, we use the pydoop.hdfs
module to interact with HDFS. We open the file using the hdfs.open()
method and read its contents using the read()
method. We can then process the contents of the file as needed.
2. Handling Large Datasets with Spark
When it comes to handling large datasets with Spark using Python, we have two main options: PySpark API and DataFrames API.
2.1. PySpark API:
The PySpark API allows us to write Spark applications using Python. To handle large datasets with Spark using Python, we need to first install Spark and PySpark in our Python environment. We can install them using pip:
pip install pyspark
Once Spark and PySpark are installed, we can start handling large datasets with Spark using Python. Here’s an example of how to read a file and perform a simple transformation using PySpark:
from pyspark.sql import SparkSession # Create a SparkSession spark = SparkSession.builder.appName("LargeDatasets").getOrCreate() # Read a file as a DataFrame df = spark.read.text("/path/to/file.txt") # Perform transformations on the DataFrame df_transformed = df.filter(df.value.contains("example")) # Show the transformed DataFrame df_transformed.show()
In this example, we first create a SparkSession using the SparkSession.builder
API. We then use the read.text()
method to read a file and load it as a DataFrame. We can then apply various transformations on the DataFrame, such as filtering rows based on a condition. Finally, we use the show()
method to display the transformed DataFrame.
2.2. DataFrames API:
The DataFrames API is an abstraction on top of the PySpark API that provides a more structured and high-level interface for working with large datasets. DataFrames are similar to tables in a relational database, allowing us to perform operations like filtering, joining, and aggregating data.
To handle large datasets with Spark using the DataFrames API, we first need to install the necessary dependencies:
pip install pyspark pandas
Once the dependencies are installed, we can start handling large datasets with Spark using the DataFrames API. Here’s an example:
from pyspark.sql import SparkSession # Create a SparkSession spark = SparkSession.builder.appName("LargeDatasets").getOrCreate() # Read a file as a DataFrame df = spark.read.text("/path/to/file.txt") # Convert the DataFrame to a Pandas DataFrame df_pandas = df.toPandas() # Perform data manipulation using Pandas df_transformed = df_pandas[df_pandas["value"].str.contains("example")] # Convert the transformed Pandas DataFrame back to a Spark DataFrame df_transformed_spark = spark.createDataFrame(df_transformed) # Show the transformed Spark DataFrame df_transformed_spark.show()
In this example, we again create a SparkSession using the SparkSession.builder
API. We read a file and load it as a DataFrame using the read.text()
method. We then convert the DataFrame to a Pandas DataFrame using the toPandas()
method. We can perform data manipulation using Pandas, and then convert the transformed Pandas DataFrame back to a Spark DataFrame using the createDataFrame()
method. Finally, we display the transformed Spark DataFrame using the show()
method.
In this tutorial, we explored how to handle large datasets using Python in the context of Hadoop and Spark. We learned how to use Pydoop to interact with HDFS and perform MapReduce jobs in Hadoop using Python. We also learned how to handle large datasets in Spark using the PySpark API and the DataFrames API. With these tools and techniques, we can efficiently manipulate and analyze big data using Python.
Source: https://www.plcourses.com/python-and-big-data-handling-large-datasets/