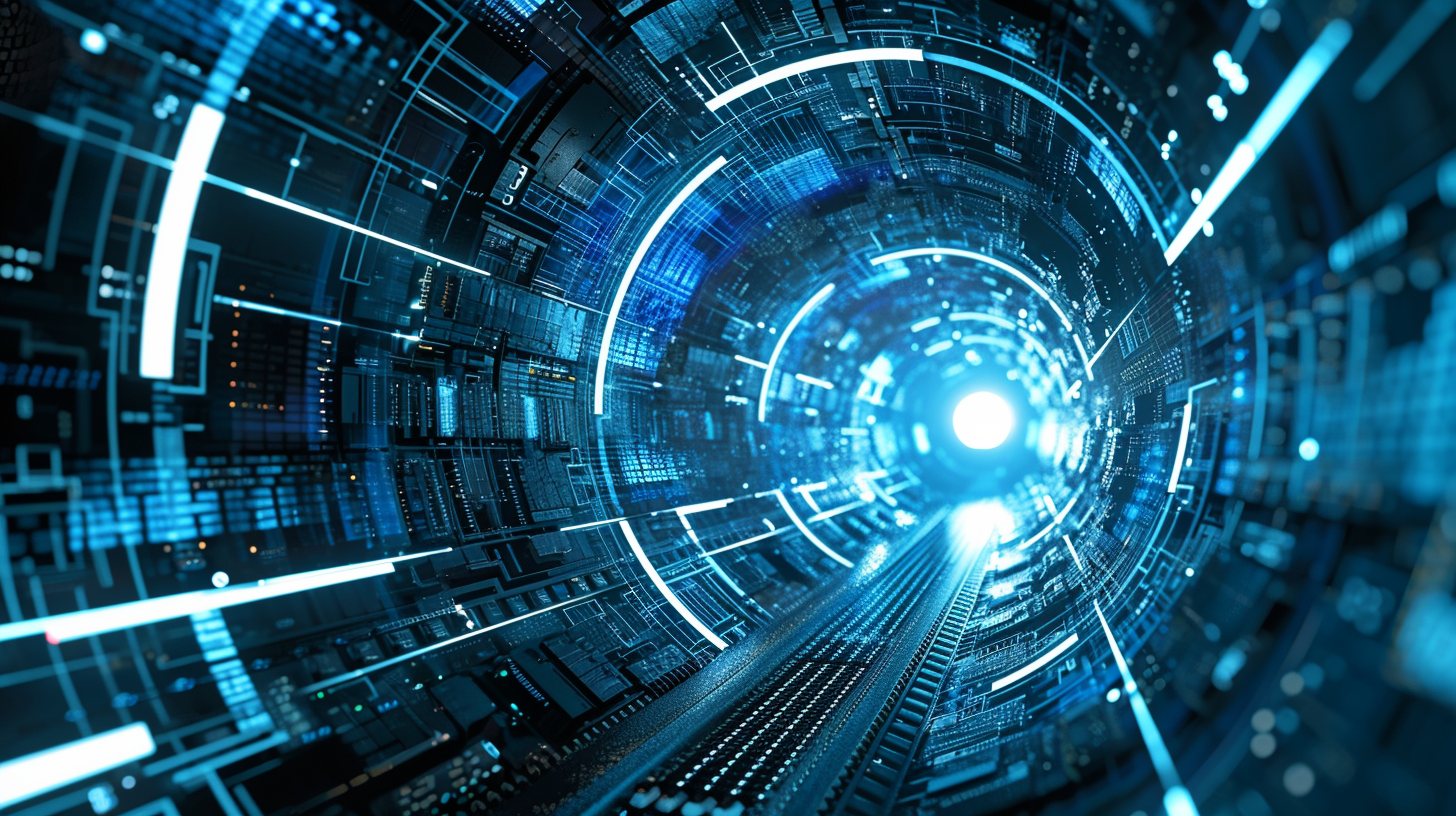
In the world of deep learning, training neural networks can be a computationally intensive and time-consuming process. Fortunately, Keras provides a powerful mechanism called callbacks that allows you to monitor and control the training process in various ways. Callbacks are functions that are called at specific stages during the training process, allowing you to perform actions such as logging metrics, saving model checkpoints, and adjusting learning rates.
The keras.callbacks
module in Keras offers a wide range of built-in callbacks that you can utilize to enhance your model training. Additionally, you can create custom callbacks tailored to your specific requirements. By using callbacks, you can gain insights into the training process, optimize model performance, and implement advanced techniques like early stopping and learning rate scheduling.
from keras.callbacks import Callback class CustomCallback(Callback): def on_epoch_end(self, epoch, logs=None): # Implement custom logic here pass
The Callback
class serves as the base class for all callbacks in Keras. It provides a set of methods that you can override to define your custom behavior. The on_epoch_end
method is called at the end of each training epoch, so that you can perform actions based on the current epoch and the recorded metrics stored in the logs
dictionary.
Keras callbacks offer a powerful and flexible way to monitor, control, and optimize the training process of your deep learning models. By using the built-in callbacks and creating custom ones, you can unlock advanced techniques and gain deeper insights into your model’s performance.
Monitoring Model Performance
One of the primary use cases for callbacks in Keras is monitoring the performance of your model during training. Keras provides several built-in callbacks that enable you to track various metrics, such as loss, accuracy, and other custom metrics you may have defined.
The keras.callbacks.CSVLogger
callback is a simple yet effective tool for logging epoch-level metrics to a CSV file. This can be particularly useful for later analysis or visualization of the training progress. Here’s an example of how to use it:
from keras.callbacks import CSVLogger csv_logger = CSVLogger('training.log') model.fit(X_train, y_train, epochs=100, batch_size=32, validation_data=(X_val, y_val), callbacks=[csv_logger])
In this example, the CSVLogger
callback will create a file named ‘training.log’ and log the epoch number, loss, and any other metrics you’ve specified during model compilation.
Another useful callback for monitoring training progress is keras.callbacks.ProgbarLogger
. This callback displays a progress bar in the terminal, showing the current epoch, loss, and other metrics. It is particularly handy when training models interactively or when you want a quick visual representation of the training progress.
from keras.callbacks import ProgbarLogger progress_bar = ProgbarLogger() model.fit(X_train, y_train, epochs=100, batch_size=32, validation_data=(X_val, y_val), callbacks=[progress_bar])
Additionally, Keras provides the keras.callbacks.TensorBoard
callback, which integrates with TensorFlow’s visualization toolkit, TensorBoard. This callback allows you to track and visualize various metrics, model graphs, and even histograms of weight and bias distributions during training. TensorBoard provides a comprehensive suite of visualization tools, making it easier to understand and debug your models.
from keras.callbacks import TensorBoard tensorboard = TensorBoard(log_dir='./logs') model.fit(X_train, y_train, epochs=100, batch_size=32, validation_data=(X_val, y_val), callbacks=[tensorboard])
By monitoring your model’s performance during training, you can gain valuable insights into its behavior, identify potential issues, and make informed decisions about adjustments or optimizations that may be required.
Early Stopping
Early stopping is a powerful technique in deep learning that helps prevent overfitting and saves computational resources by stopping the training process when the model’s performance on a validation set stops improving. The keras.callbacks.EarlyStopping callback allows you to implement this technique in your Keras models.
Here’s an example of how to use the EarlyStopping callback:
from keras.callbacks import EarlyStopping early_stop = EarlyStopping(monitor='val_loss', patience=5) model.fit(X_train, y_train, epochs=100, batch_size=32, validation_data=(X_val, y_val), callbacks=[early_stop])
In this example, the EarlyStopping callback is configured to monitor the validation loss (‘val_loss’). If the validation loss does not improve for 5 consecutive epochs (patience=5), the training process will be stopped automatically.
You can customize the behavior of the EarlyStopping callback by adjusting the following parameters:
- The metric to monitor for early stopping. You can specify any metric that you’ve defined during model compilation.
- The minimum change in the monitored metric to qualify as an improvement. This helps prevent early stopping due to small, insignificant fluctuations.
- The number of epochs to wait before stopping the training if the monitored metric does not improve.
- One of ‘auto’, ‘min’, or ‘max’. In ‘min’ mode, the training will stop when the monitored metric stops decreasing, and in ‘max’ mode, it will stop when the metric stops increasing.
- Whether to restore the model weights from the epoch with the best value of the monitored metric.
Early stopping is particularly useful when you have a finite computational budget or when you want to avoid overfitting your model to the training data. By stopping the training process when the model’s performance on the validation set plateaus, you can save time and resources while ensuring that your model generalizes well to unseen data.
Note: It is important to have a separate validation set, distinct from the training and test sets, to effectively utilize early stopping. The validation set serves as an unbiased estimate of the model’s performance during training, helping to determine when to stop the training process.
Model Checkpointing
Another powerful feature provided by Keras callbacks is model checkpointing. Model checkpointing allows you to save your model’s weights at specific intervals during training, which can be useful for several reasons:
- Saving the best model weights based on a monitored metric
- Resuming training from a previously saved state
- Maintaining a history of model weights during training
The keras.callbacks.ModelCheckpoint
callback allows you to automatically save your model’s weights during training. Here’s an example of how to use it:
from keras.callbacks import ModelCheckpoint checkpoint = ModelCheckpoint('model_weights.h5', monitor='val_loss', save_best_only=True) model.fit(X_train, y_train, epochs=100, batch_size=32, validation_data=(X_val, y_val), callbacks=[checkpoint])
In this example, the ModelCheckpoint
callback is configured to save the model’s weights to the file ‘model_weights.h5’ whenever the validation loss (‘val_loss’) improves. By setting save_best_only=True
, only the weights from the best epoch (based on the monitored metric) will be saved.
You can customize the behavior of the ModelCheckpoint
callback by adjusting the following parameters:
- The path to save the model weights file. You can include placeholders like ‘{epoch:02d}’ to include the epoch number in the file name.
- The metric to monitor for saving the best model weights.
- Whether to save only the best model weights based on the monitored metric, or save the weights after every epoch.
- One of ‘auto’, ‘min’, or ‘max’. Specifies whether to monitor for the minimum or maximum value of the monitored metric.
- Whether to save only the model’s weights or the entire model architecture and weights.
- The frequency (in epochs) at which to save the model weights.
Model checkpointing is especially useful when training large models or when you want to ensure that you don’t lose progress during long training sessions. By saving the best model weights, you can easily load and use the best-performing model for inference or further fine-tuning.
Additionally, you can combine model checkpointing with early stopping to save the best model weights before the training process is terminated due to a lack of improvement on the validation set.
from keras.callbacks import EarlyStopping, ModelCheckpoint early_stop = EarlyStopping(monitor='val_loss', patience=5) checkpoint = ModelCheckpoint('best_model.h5', monitor='val_loss', save_best_only=True) callbacks = [early_stop, checkpoint] model.fit(X_train, y_train, epochs=100, batch_size=32, validation_data=(X_val, y_val), callbacks=callbacks)
In this example, the EarlyStopping
callback will terminate the training process if the validation loss doesn’t improve for 5 consecutive epochs, while the ModelCheckpoint
callback will save the best model weights based on the validation loss. This combination ensures that you not only save computational resources by stopping training early but also retain the best-performing model weights for future use.
Learning Rate Scheduling
Learning rate scheduling is a powerful technique used in deep learning to dynamically adjust the learning rate during the training process. The learning rate is an important hyperparameter that determines the step size at which the model’s weights are updated during optimization. A well-tuned learning rate can significantly impact the convergence speed and the final performance of your model.
Keras provides several built-in callbacks that enable you to implement learning rate scheduling strategies. One of the most commonly used callbacks for this purpose is the keras.callbacks.LearningRateScheduler
. This callback allows you to define a custom learning rate schedule as a function of the current epoch or iteration.
from keras.callbacks import LearningRateScheduler # Define a learning rate schedule function def exponential_decay(epoch, lr=0.001): return lr * 0.9 ** epoch # Create the learning rate scheduler callback lr_scheduler = LearningRateScheduler(exponential_decay) model.fit(X_train, y_train, epochs=100, batch_size=32, validation_data=(X_val, y_val), callbacks=[lr_scheduler])
In the example above, we define a custom function exponential_decay
that implements an exponential learning rate decay schedule. This function takes the current epoch and an initial learning rate as input and returns the updated learning rate for that epoch. The LearningRateScheduler
callback is then initialized with this function, and it will update the learning rate of the optimizer during training according to the specified schedule.
Keras also provides a few predefined learning rate schedules through the keras.optimizers.schedules
module. For example, you can use the ExponentialDecay
schedule as follows:
from keras.optimizers.schedules import ExponentialDecay initial_learning_rate = 0.01 decay_rate = 0.9 decay_steps = 1000 lr_scheduler = ExponentialDecay( initial_learning_rate=initial_learning_rate, decay_rate=decay_rate, decay_steps=decay_steps ) optimizer = tf.keras.optimizers.Adam(learning_rate=lr_scheduler) model.compile(optimizer=optimizer, ...)
In this example, we create an ExponentialDecay
schedule and pass it directly to the optimizer’s learning_rate
parameter. The optimizer will automatically update the learning rate according to the specified schedule during training.
Learning rate scheduling can be particularly beneficial when training deep neural networks, as it can help overcome saddle points and local minima, leading to better convergence and improved model performance. Some popular learning rate schedules include:
- The learning rate is reduced by a fixed factor at specific intervals or steps.
- The learning rate decays exponentially over time or epochs.
- The learning rate follows a cosine annealing schedule, gradually decreasing towards the end of training.
- The learning rate oscillates between predefined boundary values, providing a mechanism for exploring different regions of the loss landscape.
By using the LearningRateScheduler
callback or directly using the predefined schedules in Keras, you can easily integrate learning rate scheduling strategies into your model training process, potentially leading to faster convergence and better overall performance.
Custom Callbacks
While Keras provides a wide range of built-in callbacks, there may be scenarios where you need to implement custom behavior during the training process. Fortunately, Keras allows you to define your own custom callbacks by extending the keras.callbacks.Callback
class.
To create a custom callback, you need to define a new class that inherits from Callback
and override one or more of its methods. These methods are called at specific points during the training process, which will allow you to execute your custom logic.
Here’s an example of a custom callback that prints the current learning rate after each epoch:
from keras.callbacks import Callback class LearningRateLogger(Callback): def on_epoch_end(self, epoch, logs=None): lr = self.model.optimizer.lr print(f"Epoch {epoch+1}: Learning rate = {lr:.6f}") # Usage lr_logger = LearningRateLogger() model.fit(X_train, y_train, callbacks=[lr_logger], ...)
In this example, we define a new class LearningRateLogger
that inherits from Callback
. We override the on_epoch_end
method, which is called at the end of each training epoch. Inside this method, we access the current learning rate from the model’s optimizer and print it to the console.
The Callback
class provides several methods that you can override to define custom behavior at different stages of the training process. Here are some commonly used methods:
- Called at the start of an epoch.
- Called at the end of an epoch.
- Called at the start of a batch.
- Called at the end of a batch.
- Called at the start of the training process.
- Called at the end of the training process.
These methods provide hooks into different stages of the training process, allowing you to implement custom logic, such as logging, modifying model parameters, or performing additional computations.
Custom callbacks can be particularly useful for implementing advanced techniques, such as cyclical learning rates, gradient clipping, or adaptive learning rate scheduling based on custom criteria. They also enable you to integrate external systems or services into your training process, such as logging metrics to a remote server or triggering notifications based on specific conditions.
By creating custom callbacks, you can extend the functionality of the Keras training process and tailor it to your specific requirements, enabling you to implement complex and sophisticated training strategies.
Summary and Best Practices
Keras callbacks offer a powerful and flexible mechanism for monitoring, controlling, and optimizing the training process of your deep learning models. While Keras provides a wide range of built-in callbacks, it is essential to understand best practices and considerations when working with callbacks to ensure efficient and effective model training.
Best Practices:
- While callbacks can significantly enhance your training process, using too many callbacks can introduce overhead and potentially slow down your training. Choose the callbacks that are most relevant to your specific requirements.
- Keras offers a comprehensive set of built-in callbacks that cover common use cases, such as logging, early stopping, and model checkpointing. Utilize these built-in callbacks before considering custom implementations, as they’re well-tested and optimized.
- Many callbacks can be used in combination to achieve more complex behavior. For example, you can combine early stopping with model checkpointing to save the best model weights before terminating the training process.
- When using callbacks that rely on validation data, such as early stopping and model checkpointing, it is crucial to have a separate validation set distinct from the training and test sets. This ensures an unbiased estimate of the model’s performance during training.
- Leverage callbacks like CSVLogger, ProgbarLogger, and TensorBoard to monitor and visualize the training progress. This will help you identify potential issues and make informed decisions about adjustments or optimizations.
- Implementing learning rate scheduling strategies using callbacks like LearningRateScheduler can significantly improve the convergence speed and final performance of your models.
- When creating custom callbacks, thoroughly test and validate them to ensure they behave as expected and do not introduce unintended side effects or performance issues.
By following these best practices and using the power of Keras callbacks, you can significantly enhance the training process of your deep learning models, leading to improved performance, faster convergence, and more efficient resource utilization.
from keras.callbacks import EarlyStopping, ModelCheckpoint, CSVLogger, TensorBoard early_stop = EarlyStopping(monitor='val_loss', patience=5) checkpoint = ModelCheckpoint('best_model.h5', monitor='val_loss', save_best_only=True) csv_logger = CSVLogger('training.log') tensorboard = TensorBoard(log_dir='./logs') callbacks = [early_stop, checkpoint, csv_logger, tensorboard] model.fit(X_train, y_train, epochs=100, batch_size=32, validation_data=(X_val, y_val), callbacks=callbacks)
This example demonstrates the combination of several built-in callbacks, including early stopping, model checkpointing, CSV logging, and TensorBoard integration. By carefully selecting and combining these callbacks, you can monitor the training progress, save the best model weights, log metrics to a file, and visualize the training process using TensorBoard.
Source: https://www.pythonlore.com/using-keras-callbacks-for-monitoring-and-performance-tuning/