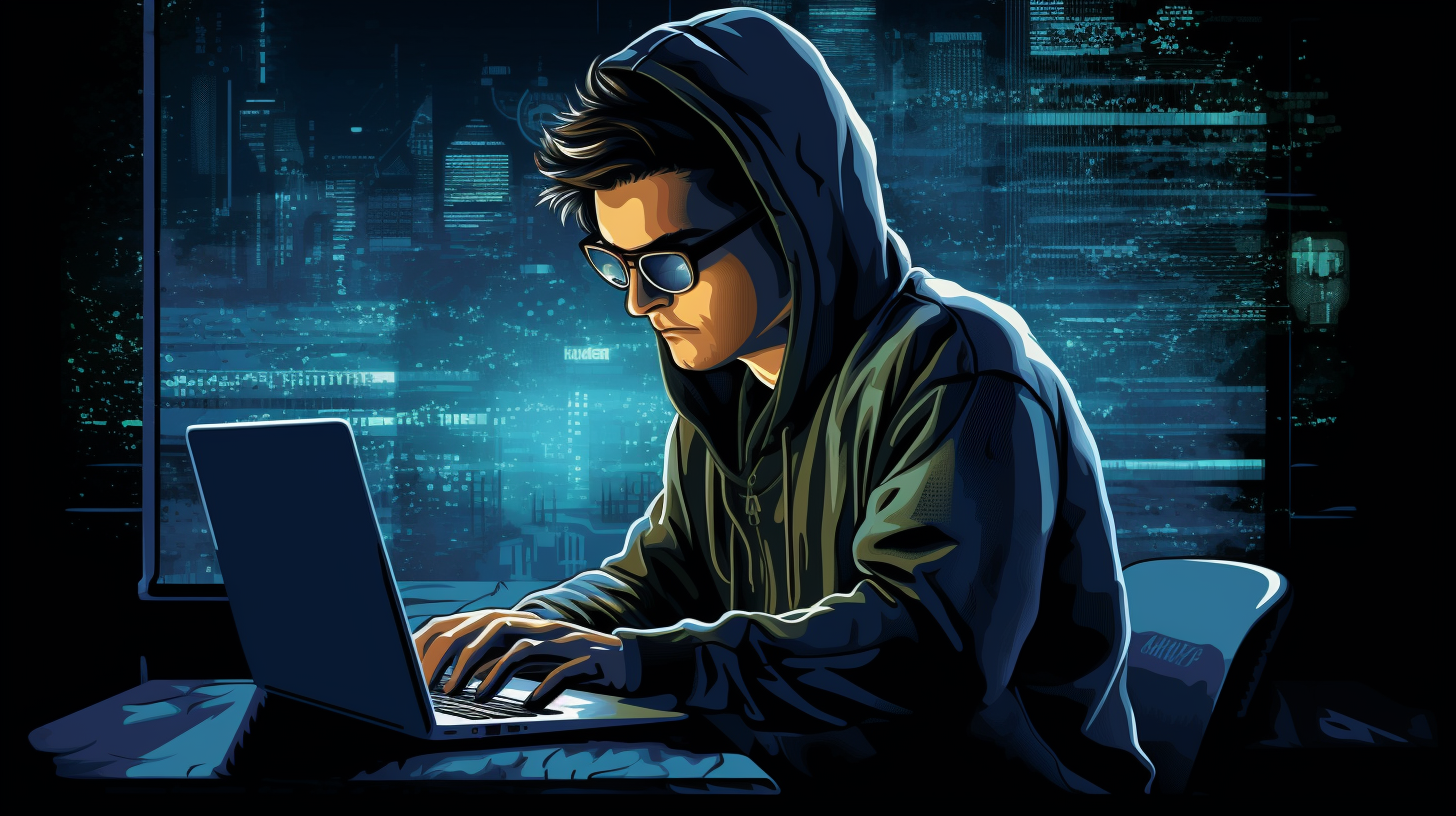
Gradient Boosting Machines (GBM) are a powerful machine learning technique that have proven successful across a wide range of predictive modeling tasks. They are part of the ensemble methods, which combine the predictions from multiple models to improve accuracy and robustness over a single model. GBM specifically is an iterative algorithm that builds an ensemble of weak prediction models, typically decision trees, to create a strong overall model.
The core idea behind Gradient Boosting is to sequentially add predictors to an ensemble, each one correcting its predecessor. That’s achieved by fitting the new predictor to the residual errors made by the previous predictor. Instead of minimizing the loss function by adjusting the model parameters, as is done in classical methods like linear regression, Gradient Boosting focuses on minimizing the loss function by sequentially adding models that correct the errors of the ensemble.
One of the key advantages of Gradient Boosting Machines is their ability to work with heterogeneous features (numerical and categorical data), handle missing data, and robustness to outliers in output space (via robust loss functions). They’re also flexible enough to optimize different loss functions, which can be tailored to the problem at hand, be it binary classification, multi-class classification, or regression tasks.
However, GBMs can be computationally expensive and prone to overfitting if not managed properly with hyperparameters tuning. They also require careful preprocessing of data and feature engineering to achieve the best results. Despite these challenges, when properly tuned and trained, Gradient Boosting Machines can provide highly accurate and interpretable models.
One of the most popular implementations of Gradient Boosting Machines is available in the Python library scikit-learn, which provides a simple and efficient tool for data mining and data analysis. Scikit-learn’s GradientBoostingClassifier
and GradientBoostingRegressor
are uncomplicated to manage classes that allow developers to harness the power of GBM with minimal code.
from sklearn.ensemble import GradientBoostingClassifier # Create a Gradient Boosting Classifier gb_clf = GradientBoostingClassifier(n_estimators=100, learning_rate=1.0, max_depth=1, random_state=0) gb_clf.fit(X_train, y_train)
This example shows the creation of a Gradient Boosting Classifier with 100 trees, a learning rate of 1.0, and a maximum depth of 1 for each tree. The model is then trained on a dataset represented by X_train
and y_train
. That’s just a starting point, as the power of GBM comes from its flexibility and the tuning of its parameters, which will be discussed in later sections.
Building a Gradient Boosting Model with scikit-learn
After initializing the GradientBoostingClassifier, the next step is to fit the model to your training data. This step very important as it is where the iterative process of building trees and correcting errors from previous trees occurs. The fit
method takes two main arguments: the features X_train
and the target y_train
.
gb_clf.fit(X_train, y_train)
Once the model has been trained, you can use it to make predictions on new, unseen data. That is done using the predict
method. For classification tasks, this method returns the class label that the model predicts for the input data.
y_pred = gb_clf.predict(X_test)
It is also possible to assess the probability of each class, which can be useful for understanding the model’s confidence in its predictions or for setting a custom threshold for classification.
y_pred_probs = gb_clf.predict_proba(X_test)
Scikit-learn’s GradientBoostingClassifier also provides methods for assessing the performance of the model. One such method is score
, which provides a simple way to get the accuracy of the model on a given test dataset.
accuracy = gb_clf.score(X_test, y_test)
Another important aspect of building a Gradient Boosting Model is handling categorical features. Scikit-learn’s GBM implementation does not handle categorical variables natively, and these need to be preprocessed appropriately before training the model. One common approach is to use one-hot encoding to transform categorical variables into a form that can be provided to the model to learn from.
Building a Gradient Boosting Model with scikit-learn involves initializing the model with desired hyperparameters, fitting the model to the training data, making predictions, and evaluating the model’s performance. Proper preprocessing of data, especially for handling categorical variables, is also a key step in the process of building an effective GBM.
Tuning Hyperparameters for Improved Performance
One critical aspect of getting the most out of Gradient Boosting Machines is the tuning of hyperparameters. Hyperparameters are the configuration settings used to structure the machine learning algorithms. Unlike model parameters, which are learned during training, hyperparameters are set before the training process begins.
The primary hyperparameters in GBM that require tuning for improved performance are:
- The number of boosting stages to be run, which essentially represents the number of trees in the model. A higher number of trees can improve performance but also increases the risk of overfitting.
- This controls the contribution of each tree in the ensemble. A smaller learning rate requires more trees in the ensemble but often leads to a more robust model.
- The maximum depth of the individual regression estimators. This controls the complexity of the trees.
- The minimum number of samples required to split an internal node. This can vary the degree of bias and variance in the model.
- The minimum number of samples required to be at a leaf node. This parameter has a similar effect to min_samples_split.
- The number of features to ponder when looking for the best split. This can help in improving the performance if the feature space is large.
- The fraction of samples to be used for fitting the individual base learners. A value lower than 1.0 leads to a reduction of variance and an increase in bias.
Hyperparameter tuning can be done manually, through a grid search, or using randomized searches. Scikit-learn provides GridSearchCV
and RandomizedSearchCV
for automating this process. Here’s a simple example of using GridSearchCV to tune hyperparameters:
from sklearn.model_selection import GridSearchCV param_grid = { 'n_estimators': [100, 200, 300], 'learning_rate': [0.01, 0.1, 1.0], 'max_depth': [3, 4, 5], 'min_samples_split': [2, 3], 'min_samples_leaf': [1, 2], 'subsample': [0.9, 1.0] } gb_clf = GradientBoostingClassifier(random_state=0) grid_search = GridSearchCV(estimator=gb_clf, param_grid=param_grid, cv=5, n_jobs=-1) grid_search.fit(X_train, y_train) best_parameters = grid_search.best_params_ print(best_parameters)
After identifying the best hyperparameters, the model can then be retrained using these optimized values to hopefully achieve better performance:
gb_clf_optimized = GradientBoostingClassifier( n_estimators=best_parameters['n_estimators'], learning_rate=best_parameters['learning_rate'], max_depth=best_parameters['max_depth'], min_samples_split=best_parameters['min_samples_split'], min_samples_leaf=best_parameters['min_samples_leaf'], subsample=best_parameters['subsample'], random_state=0 ) gb_clf_optimized.fit(X_train, y_train)
Tuning the hyperparameters can significantly improve the performance of a Gradient Boosting Machine model. However, it is important to monitor the model to avoid overfitting, which can happen with too many trees or too complex trees. A well-tuned GBM model can lead to better accuracy and a more generalized model that performs well on unseen data.
Evaluating Model Performance and Interpretability
Evaluating the performance of a Gradient Boosting Machine (GBM) model involves not only looking at its accuracy but also at other key metrics that can provide a more nuanced understanding of the model’s predictions. Commonly used evaluation metrics include precision, recall, F1-score for classification tasks, and mean squared error, mean absolute error, and R-squared for regression tasks.
Scikit-learn provides a handy classification_report
function that can be used to generate a report on several of these metrics for classification problems:
from sklearn.metrics import classification_report y_true = y_test y_pred = gb_clf.predict(X_test) print(classification_report(y_true, y_pred))
For regression problems, scikit-learn also provides functions like mean_squared_error
and r2_score
that can be used to evaluate the model:
from sklearn.metrics import mean_squared_error, r2_score y_true = y_test y_pred = gb_clf.predict(X_test) mse = mean_squared_error(y_true, y_pred) r2 = r2_score(y_true, y_pred) print(f'Mean Squared Error: {mse}') print(f'R-squared: {r2}')
Model interpretability is another crucial aspect of model evaluation, especially for complex models like GBMs. Being able to understand and explain the predictions made by the model is important in many fields, particularly in industries like finance and healthcare where decisions can have significant consequences.
One way to interpret GBM models is through feature importance, which shows the relative importance of each feature in making predictions. Scikit-learn provides a simple way to access feature importances after the model has been trained:
feature_importances = gb_clf.feature_importances_ print(feature_importances)
For a more detailed view, one can visualize the feature importances using libraries like Matplotlib or Seaborn:
import matplotlib.pyplot as plt import seaborn as sns sns.barplot(x=feature_importances, y=X_train.columns) plt.title('Feature Importance') plt.show()
Another approach for model interpretability is to use techniques such as Partial Dependence Plots (PDP) and SHAP (SHapley Additive exPlanations) values, which can provide insights into how the model makes predictions.
Overall, evaluating the performance and interpretability of a GBM requires a combination of metrics and techniques to ensure that the model not only predicts accurately but also provides insights that can inform decision-making processes.
Source: https://www.pythonlore.com/implementing-gradient-boosting-machines-with-scikit-learn/