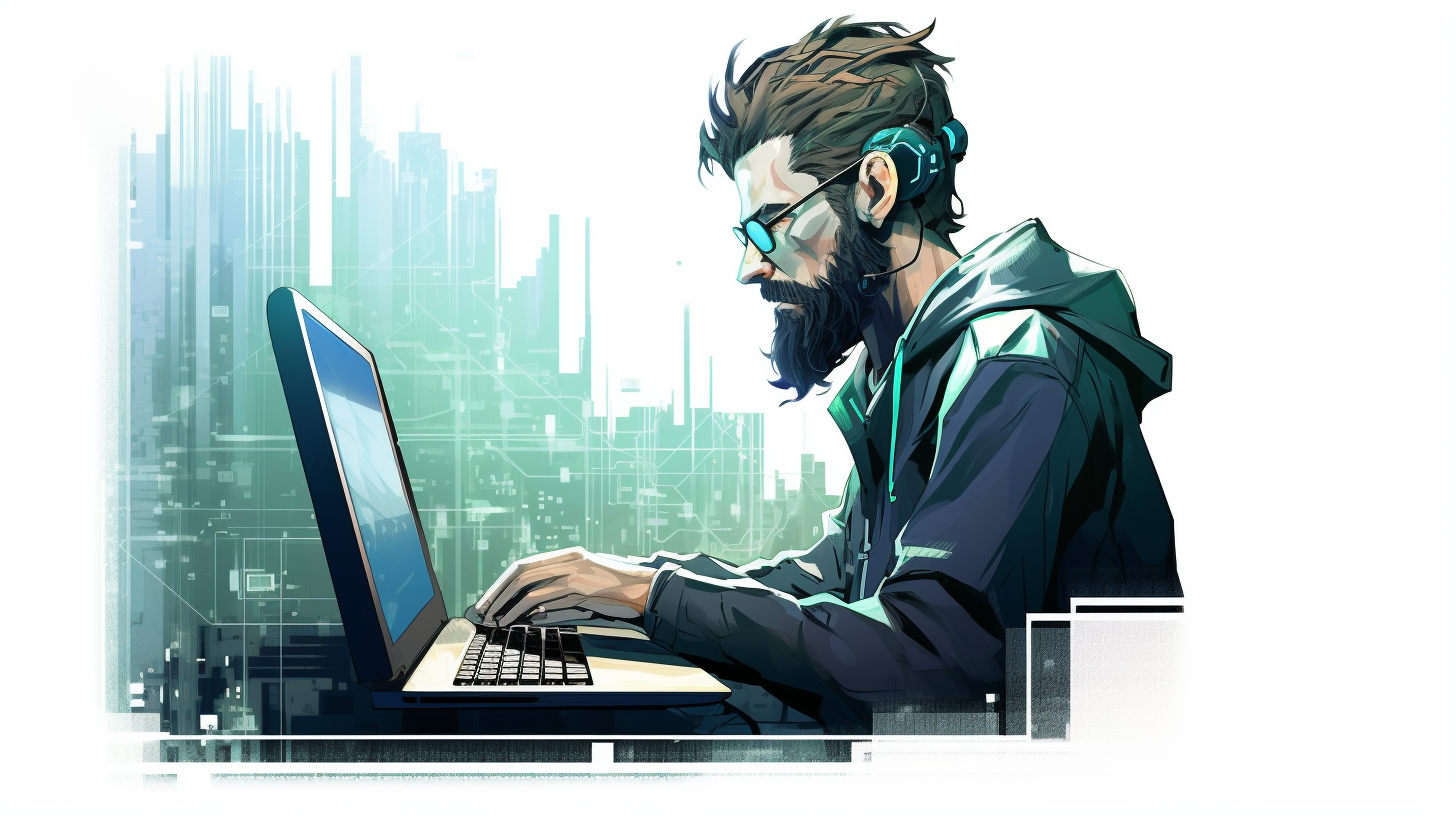
Networking in Pygame is an essential aspect for creating multiplayer games and applications that require online interactions. Pygame does not have built-in networking capabilities; however, Python’s robust libraries such as socket, asyncio, and third-party libraries like podsixnet can be integrated to add networking features. These libraries enable Pygame applications to communicate over the internet or a local network, allowing for real-time data exchange between different instances of a game or application.
To begin with networking in Pygame, one must understand the basics of client-server architecture. In this model, a server is set up to listen for connections from clients. When a client connects, a two-way communication channel is established. The server can handle multiple clients simultaneously, broadcasting messages to all clients or sending specific messages to individual clients. The communication is typically handled using the Transmission Control Protocol (TCP) or the User Datagram Protocol (UDP), depending on the requirements of the application.
Here is a simple example of how to create a TCP server in Python:
import socket # Create a TCP/IP socket server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Bind the socket to the address and port server_address = ('localhost', 10000) server_socket.bind(server_address) # Listen for incoming connections server_socket.listen(1) while True: # Wait for a connection print('waiting for a connection') connection, client_address = server_socket.accept() try: print('connection from', client_address) # Receive and send data back and forth while True: data = connection.recv(16) print('received {!r}'.format(data)) if data: print('sending data back to the client') connection.sendall(data) else: print('no more data from', client_address) break finally: # Clean up the connection connection.close()
Once the server is set up, clients can connect to it using the socket.connect()
method, specifying the server’s address and port. In subsequent sections, we will delve deeper into setting up a server, connecting clients, implementing online features, and handling network errors and troubleshooting in Pygame.
Setting Up a Server in Pygame
When setting up a server in Pygame, it’s important to decide on the protocol to use. TCP is a reliable, connection-oriented protocol that ensures data is received in the order it was sent. UDP, on the other hand, is a connectionless protocol that does not guarantee the order or even the delivery of the data. For most multiplayer games, TCP is the preferred protocol due to its reliability.
Once you have decided on the protocol, the next step is to create a socket object and bind it to an IP address and port number. That’s the address and port that clients will use to connect to the server. Use the socket.bind()
method to associate the socket with the server’s address.
# Import the socket library import socket # Create a TCP/IP socket server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Define the server address and port number server_address = ('localhost', 10000) # Bind the socket to the server address server_socket.bind(server_address)
After binding the socket, the next step is to put the server into listening mode with the socket.listen()
method. The argument passed to listen()
specifies the maximum number of queued connections.
# Put the server into listening mode server_socket.listen(5) print('Server is listening on port', server_address[1])
Now the server is ready to accept connections. Use the socket.accept()
method to accept a connection from a client. This method blocks and waits for an incoming connection. When a client connects, it returns a new socket object representing the connection and a tuple with the client’s address.
# Wait for a client connection connection, client_address = server_socket.accept() print('Got a connection from', client_address)
With the connection established, the server can now exchange messages with the client using connection.recv()
to receive data and connection.send()
or connection.sendall()
to send data. It is important to handle exceptions and ensure that the connection is properly closed when finished.
try: # Receive data from the client data = connection.recv(1024) print('Received {!r}'.format(data)) # Send data to the client if data: connection.sendall(data) else: print('No data from', client_address) finally: # Clean up the connection connection.close()
It’s a good practice to wrap the server code in a loop, so it can handle multiple connections one after another.
Remember that that is just a basic server setup. In a real-world scenario, you would likely need to handle multiple connections concurrently, deal with various types of network errors, and implement security measures such as encryption. However, this basic setup is a good starting point to understand how networking works in Pygame.
Connecting Clients to the Server
Connecting clients to a Pygame server involves creating a socket on the client side and then using the connect()
method to establish a connection to the server’s IP address and port number. Below is an example of how to connect a client to a Pygame server using TCP:
# Import the socket library import socket # Create a TCP/IP socket client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Define the server address and port number server_address = ('localhost', 10000) # Connect the socket to the server's address client_socket.connect(server_address) try: # Send data to the server message = 'This is the message. It will be repeated.' client_socket.sendall(message.encode('utf-8')) # Look for the response amount_received = 0 amount_expected = len(message) while amount_received < amount_expected: data = client_socket.recv(16) amount_received += len(data) print('received {!r}'.format(data.decode('utf-8'))) finally: # Clean up the connection client_socket.close()
When the client calls connect()
, the client initiates a three-way handshake with the server. If the server is listening and accepts the connection, the client and server can start communicating. The client sends data using sendall()
and receives data using recv()
, just like the server.
It’s important to note that the client should handle exceptions to deal with potential network issues, such as the server not being available or the connection being lost. Here’s an example of how to handle exceptions on the client side:
try: # Connect the socket to the server's address client_socket.connect(server_address) # Code to send and receive data as shown above except socket.error as e: print('Socket error:', e) except Exception as e: print('Other exception:', e) finally: client_socket.close()
This simple client setup is sufficient to connect to a Pygame server and exchange messages. For more advanced scenarios, such as handling multiple clients, non-blocking sockets or asynchronous I/O might be required.
Implementing Online Features
Implementing online features in a Pygame application involves more than just setting up a server and connecting clients. It is about creating a dynamic and interactive experience for users playing the game. This can be achieved by implementing various online features such as chat, multiplayer gameplay, and real-time updates.
Let’s take a look at how we can implement a simple chat feature in a Pygame application. To start with, we will need a way for the server to broadcast messages received from one client to all other connected clients. Here’s an example of how this can be done:
# Server-side code to broadcast messages to all clients connected_clients = [] while True: # Wait for a connection connection, client_address = server_socket.accept() connected_clients.append(connection) try: while True: data = connection.recv(16) if data: # Broadcast message to all connected clients for client in connected_clients: if client != connection: client.sendall(data) else: break finally: connected_clients.remove(connection) connection.close()
On the client side, we need to modify the code to handle incoming messages from other clients. We can do this by running a loop that constantly listens for new messages:
# Client-side code to handle incoming messages try: while True: # Wait for incoming messages data = client_socket.recv(16) if data: print('Message from other client: {!r}'.format(data.decode('utf-8'))) except KeyboardInterrupt: pass finally: client_socket.close()
For multiplayer gameplay, the server needs to keep track of the game state and ensure that all actions taken by players are reflected in real-time to all other players. This could include player movements, score updates, or any other game-related data. The server could use a game loop similar to the main loop in Pygame but designed to handle network messages instead of events. For example:
# Server-side code to handle game state updates game_state = {} while True: for client in connected_clients: data = client.recv(1024) if data: game_action = parse_data(data) update_game_state(game_state, game_action) broadcast_game_state(game_state, connected_clients)
Where parse_data
is a function that converts the raw data received from a client into a game action, update_game_state
updates the global game state based on that action, and broadcast_game_state
sends the updated game state to all clients.
As for real-time updates, using a non-blocking approach or asynchronous I/O can be beneficial. With a non-blocking socket, the server can check for incoming data and handle it without pausing the rest of the program. That is achieved by setting the socket to non-blocking mode with the setblocking
method:
# Set the socket to non-blocking mode server_socket.setblocking(False)
Alternatively, Python’s asyncio
library can be used to achieve asynchronous I/O, which allows for handling multiple clients and real-time updates more efficiently.
Finally, when implementing online features, it’s important to think the security and integrity of the data being exchanged. Ensure that sensitive information is encrypted and that data received from clients is validated to prevent malicious activities.
By carefully designing the network communication and implementing these features, a Pygame application can offer an engaging and interactive online experience for its users.
Handling Network Errors and Troubleshooting
When working with networking in Pygame, it is inevitable that you’ll encounter network errors at some point. Handling these errors gracefully is essential to ensure a smooth user experience. Common network errors include connection timeouts, refused connections, and unexpected disconnections. Here’s how you can handle some of these errors:
Connection Timeouts: A connection timeout occurs when a client attempts to connect to a server, but the server does not respond within a specified time frame. To handle this, you can set a timeout value on the socket using the settimeout()
method. If the connection is not established within the given time, a socket.timeout
exception is raised.
# Set a timeout for the connection attempt client_socket.settimeout(5.0) try: client_socket.connect(server_address) except socket.timeout: print('Connection timed out')
Refused Connections: A connection refused error occurs when the server is not listening on the specified port, or the connection is blocked by a firewall. This raises a ConnectionRefusedError
. To handle this, you can use a try-except block to catch the error and respond accordingly.
try: client_socket.connect(server_address) except ConnectionRefusedError: print('Connection refused by the server')
Unexpected Disconnections: An unexpected disconnection can happen for various reasons, such as network issues or the server crashing. When this occurs, a socket.error
is raised. You can catch this error and attempt to reconnect or terminate the connection gracefully.
try: data = client_socket.recv(1024) except socket.error as e: print('Connection lost:', e)
When troubleshooting network issues in Pygame, it’s also important to use logging or print statements to help identify where the problem lies. Additionally, you can use network analysis tools such as Wireshark to monitor network traffic and diagnose issues.
Remember that networking is complex, and there are many factors that can cause errors. Patience and careful debugging are key to resolving these issues. By anticipating and handling common network errors, you can create a more sturdy and durable Pygame application.
Source: https://www.pythonlore.com/networking-and-online-features-in-pygame/