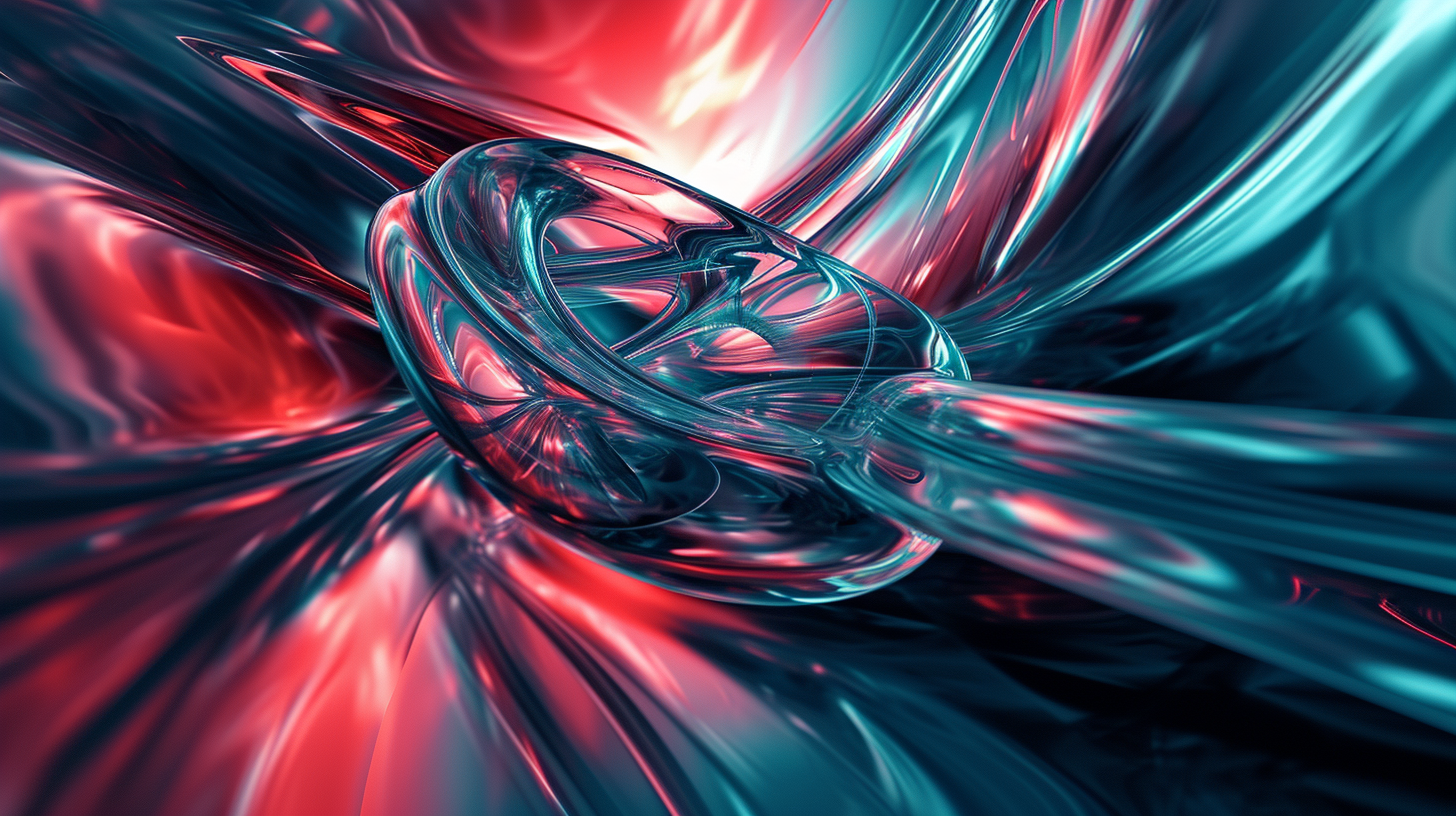
When working with data in Python, it’s common to encounter datasets that have missing or NaN (Not a Number) values. This can happen for a variety of reasons, such as data entry errors, data corruption, or simply because the information is not applicable or was not collected. Missing data can pose significant challenges when it comes to analyzing and manipulating the dataset.
To handle missing data effectively, the pandas library offers a powerful tool: DataFrame.dropna(). This method allows us to remove missing data from our DataFrame in a flexible and simpler manner. Whether you want to drop rows or columns with missing values, or set a threshold for the amount of missing data you are willing to tolerate, dropna()
provides a range of options to clean your dataset.
Before we dive into how to use dropna()
, it is important to understand the impact of missing data on your analysis. Depending on the nature of your data and the goals of your project, you may need to carefully ponder the trade-offs between dropping data and imputing missing values. Dropping data can lead to a loss of valuable information, especially if the missing values are not randomly distributed. On the other hand, keeping too much missing data can skew your results and lead to unreliable conclusions.
With pandas, you have the flexibility to handle missing data in a way that best suits your needs. In the following sections, we’ll explore how to identify missing data in your DataFrame, and how to use dropna()
to clean your data effectively.
Let’s start by looking at some example code that creates a simple DataFrame with missing values:
import pandas as pd import numpy as np # Create a DataFrame with missing values data = {'Name': ['John', 'Anna', 'Peter', 'Linda', None], 'Age': [28, None, 34, 29, 32], 'Salary': [50000, 62000, None, 54000, 58000]} df = pd.DataFrame(data) print(df)
This code block generates a DataFrame with some intentionally missing values. In the next section, we’ll learn how to identify these missing values within our DataFrame.
Identifying Missing Data in a DataFrame
Identifying missing data in a pandas DataFrame is an essential first step before deciding how to handle it. pandas provides several methods to detect missing values, which makes it easy to find and address them. The isnull() and notnull() functions are commonly used to identify missing values. Let’s see these functions in action using our example DataFrame:
# Identify missing values using isnull() missing_values = df.isnull() print(missing_values)
The isnull()
function will return a DataFrame of the same size as the original, but with boolean values indicating the presence of missing data. A True value indicates a missing value, while a False value indicates a non-missing value. Similarly, the notnull()
function works in the opposite way, returning True for non-missing values and False for missing values.
# Identify non-missing values using notnull() non_missing_values = df.notnull() print(non_missing_values)
Another way to get a quick overview of missing data in the DataFrame is by using the info() method. This method provides a concise summary of the DataFrame, including the number of non-null entries for each column:
# Get summary information including non-null counts df.info()
For a more direct approach, we can use the sum() method in combination with isnull()
to count the number of missing values per column:
# Count the number of missing values per column missing_count = df.isnull().sum() print(missing_count)
Lastly, if we want to check if there are any missing values at all in the DataFrame, we can use the any() method:
# Check if there are any missing values in the DataFrame any_missing = df.isnull().any() print(any_missing)
Once we have identified the missing data, we can make more informed decisions about how to handle it. In the next sections, we’ll discuss how to drop rows and columns with missing data using the dropna()
method.
Dropping Rows with Missing Data
Now that we’ve identified the missing data in our DataFrame, let’s move on to the process of dropping rows with missing data using the dropna()
method. This method is highly customizable and allows us to specify how we want to handle missing values. By default, dropna()
will remove any row that contains at least one missing value.
Here’s an example of how to use dropna()
to drop rows with missing data:
# Drop rows with any missing values df_dropped_rows = df.dropna() print(df_dropped_rows)
The resulting DataFrame, df_dropped_rows
, will only contain rows that have no missing values at all. However, this approach might not always be suitable, as it can result in a significant loss of data, especially if your dataset has a lot of missing values.
To have more control over which rows to drop, we can use the axis
and how
parameters. The axis
parameter determines whether to drop labels from the index (0 or ‘index’) or columns (1 or ‘columns’). The how
parameter can take on values ‘any’ or ‘all’, where ‘any’ drops the row if any NA value is present, and ‘all’ drops the row only if all values are NA.
For example, if we only want to drop rows where all values are missing, we can do the following:
# Drop rows where all values are missing df_dropped_all_na = df.dropna(how='all') print(df_dropped_all_na)
Sometimes, we might have columns that are more important than others, and we only want to drop rows that have missing values in those specific columns. We can achieve this by using the subset
parameter. The subset
parameter takes a list of column names to think when dropping rows.
For instance, if we ponder the ‘Age’ column to be critical and want to drop rows that have missing values in that column, we can do the following:
# Drop rows with missing values in the 'Age' column df_dropped_age = df.dropna(subset=['Age']) print(df_dropped_age)
In summary, the dropna()
method provides a convenient way to remove rows with missing data from a DataFrame. By understanding and using the available parameters, we can tailor the method to fit the specific needs of our analysis and ensure that we’re working with a clean and reliable dataset.
Dropping Columns with Missing Data
Now let’s focus on how to drop columns with missing data using the dropna()
method. Similar to dropping rows, we can also remove entire columns that contain missing values. This can be particularly useful when a column has a high percentage of missing data, making it less reliable or useful for analysis.
To drop columns with any missing values, we simply set the axis
parameter to 1 or ‘columns’. Here’s how we do it:
# Drop columns with any missing values df_dropped_columns = df.dropna(axis='columns') print(df_dropped_columns)
The resulting DataFrame, df_dropped_columns
, will only contain columns that have no missing values at all. However, just like with rows, dropping too many columns can lead to a loss of potentially valuable data.
If we want to be more selective and only drop columns where all values are missing, we can combine the axis
parameter with the how
parameter, setting it to ‘all’:
# Drop columns where all values are missing df_dropped_columns_all_na = df.dropna(axis='columns', how='all') print(df_dropped_columns_all_na)
In some cases, we might decide that we only want to drop a column if it has more than a certain number of missing values. This is where the thresh
parameter comes into play. The thresh
parameter allows us to specify a minimum number of non-NA values that a column must have in order to not be dropped.
For example, if we want to keep only the columns that have at least 4 non-missing values, we can use the following code:
# Drop columns with less than 4 non-missing values df_dropped_thresh = df.dropna(axis='columns', thresh=4) print(df_dropped_thresh)
It is important to carefully think the implications of dropping columns from your DataFrame. While it can simplify the dataset and make it easier to work with, it can also result in the loss of important information. By understanding the available options and thinking critically about your dataset and analysis goals, you can use the dropna()
method to manage missing data effectively and ensure the integrity of your results.
Handling Missing Data with Thresholds
Handling Missing Data with Thresholds
When working with large datasets, it may not be practical to drop every row or column that contains missing data. In some cases, you might want to keep rows or columns that have a certain level of completeness. This is where thresholds come into play. The thresh parameter in the dropna()
method allows you to specify a minimum number of non-missing values required to keep a row or column.
Let’s say we have a DataFrame where we’re willing to tolerate some missing data, but we want to ensure that each row has at least 2 non-missing values. We can use the following code:
# Drop rows with less than 2 non-missing values df_dropped_row_thresh = df.dropna(thresh=2) print(df_dropped_row_thresh)
The thresh
parameter can also be combined with the subset
parameter to apply thresholds to specific columns. For example, if we have a dataset where the ‘Salary’ column is important and we want to keep rows that have at least 1 non-missing value in the ‘Salary’ column, we can do the following:
# Drop rows with missing 'Salary' but require at least 1 non-missing value in 'Salary' df_dropped_salary_thresh = df.dropna(subset=['Salary'], thresh=1) print(df_dropped_salary_thresh)
Setting thresholds is a powerful way to balance the need for complete data with the reality of missing values. It allows you to retain as much data as possible while still ensuring a certain level of data quality. When deciding on the appropriate threshold for your analysis, consider the importance of each variable and the nature of your data. It is a judgment call that can have a significant impact on your results.
The use of thresholds is not limited to dropping rows. You can apply the same principle to columns as well. If you want to keep only columns that have a minimum number of non-missing values, you can set the axis
parameter to ‘columns’ and use the thresh
parameter accordingly:
# Drop columns with less than 3 non-missing values df_dropped_column_thresh = df.dropna(axis='columns', thresh=3) print(df_dropped_column_thresh)
In summary, the thresh
parameter in the dropna()
method provides a flexible way to handle missing data according to the level of completeness you require for your analysis. By setting thresholds, you can make informed decisions about which data to keep and which to discard, ensuring that you work with a dataset that meets your specific needs.
Source: https://www.pythonlore.com/handling-missing-data-with-pandas-dataframe-dropna/