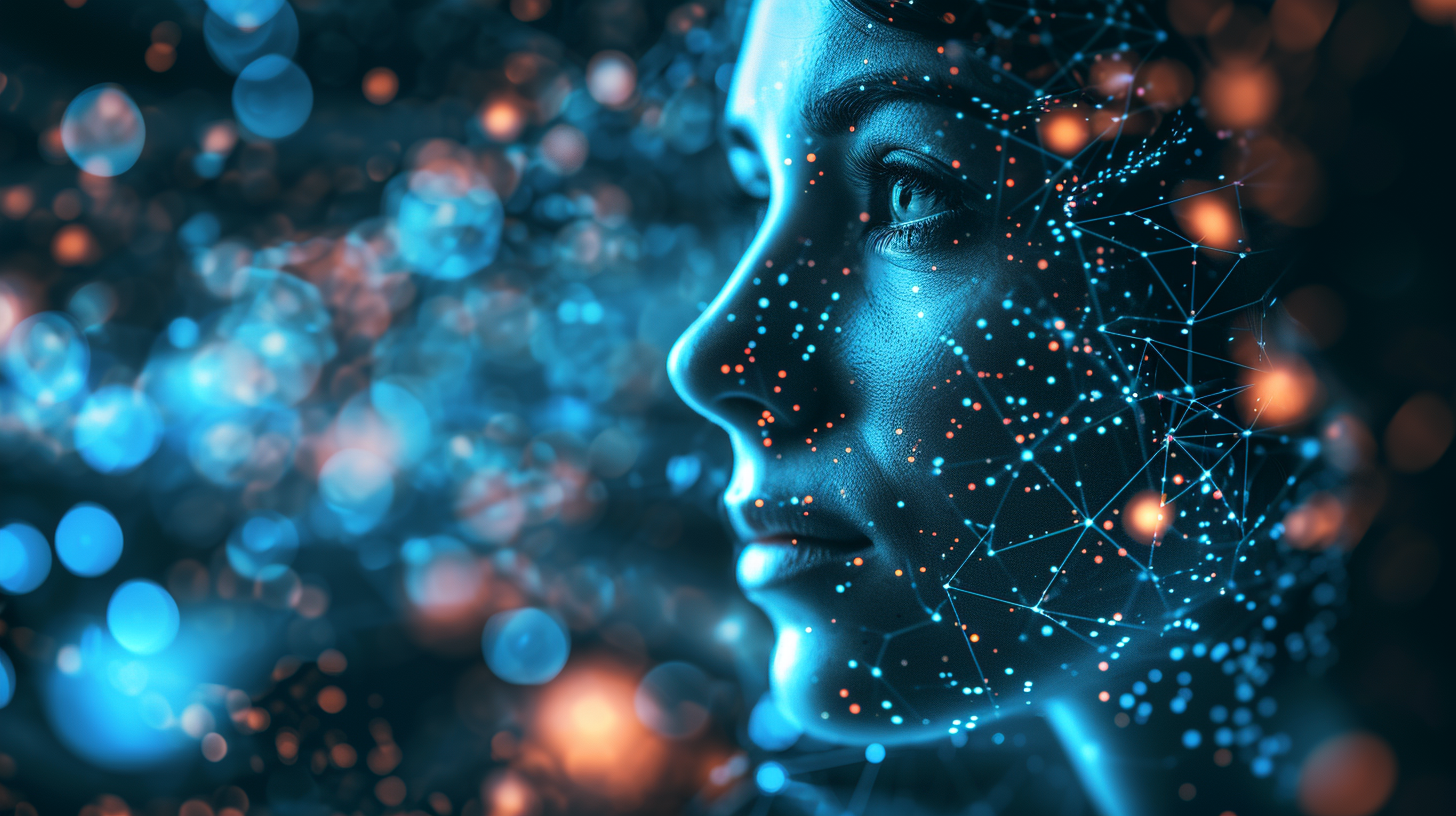
Asynchronous I/O, often referred to as async I/O, is a programming paradigm that enables efficient execution of I/O-bound operations without blocking the execution thread. In traditional synchronous I/O, a program must wait for an I/O operation, such as reading from or writing to a network socket or file, to complete before moving on to the next instruction. This can lead to performance bottlenecks, especially in applications that require handling multiple I/O operations simultaneously.
Async I/O, on the other hand, allows a program to initiate an I/O operation and then continue executing other tasks while the operation is being processed in the background. When the I/O operation is completed, the program is notified to handle the result. This approach helps to improve the overall responsiveness and throughput of the application by using system resources more effectively.
In Python, the asyncio library provides a foundation for writing asynchronous I/O code. It introduces the idea of coroutines, which are special functions that can be paused and resumed during their execution. Coroutines enable the creation of asynchronous code that looks and behaves similarly to synchronous code but allows for non-blocking behavior.
Let’s take a look at a simple example of async I/O using asyncio
in Python:
import asyncio async def main(): print('Hello') await asyncio.sleep(1) print('World') asyncio.run(main())
In this code snippet, the main
function is defined as an async coroutine using the async
keyword. Inside the coroutine, after printing ‘Hello’, the await
keyword is used in conjunction with asyncio.sleep(1)
to create a non-blocking delay of one second. While the program is “sleeping”, the event loop can run other tasks, such as handling I/O operations. After the delay, the program resumes execution and prints ‘World’.
That’s just a simple illustration of async I/O in action. In the following sections, we will delve deeper into asyncio streams and how to implement asynchronous I/O operations using them in Python.
Understanding asyncio Streams
Asyncio streams are high-level, easy-to-use primitives for working with network connections. They provide an abstraction over the low-level details of non-blocking I/O, allowing developers to focus on the logic of their application rather than the intricacies of the underlying network protocols. Streams are one of the key features provided by the asyncio library to facilitate asynchronous I/O.
There are two main types of asyncio streams: StreamReader and StreamWriter. A StreamReader object represents the receiving end of the network connection, which you can use to read data from the network asynchronously. Similarly, a StreamWriter object represents the sending end, which you can use to send data to the network.
To work with asyncio streams, you need to establish a connection. That’s typically done using the asyncio.open_connection
function, which returns a pair of StreamReader and StreamWriter objects. Here is an example:
import asyncio async def tcp_echo_client(message): reader, writer = await asyncio.open_connection( '127.0.0.1', 8888) print(f'Send: {message!r}') writer.write(message.encode()) await writer.drain() data = await reader.read(100) print(f'Received: {data.decode()!r}') print('Close the connection') writer.close() await writer.wait_closed() asyncio.run(tcp_echo_client('Hello World!'))
In the example above, we define an async coroutine tcp_echo_client
that connects to a TCP server at localhost on port 8888. We send a message to the server and then wait for a response. The reader.read
method is used to read up to 100 bytes from the network, and the writer.write
method is used to send bytes to the network. The writer.drain
method is an important step, as it allows the writer to flush the internal buffer, ensuring that all data is sent.
When working with streams, it’s important to properly manage resources by closing the connection when it is no longer needed. In the example, we call writer.close()
followed by await writer.wait_closed()
to close the StreamWriter and wait until the connection is fully closed.
Asyncio streams provide a more intuitive and high-level API compared to the lower-level transport and protocol APIs also available in asyncio. By using streams, you can write code this is both efficient and easy to read, making it an excellent choice for networking applications that require asynchronous I/O.
Implementing Asynchronous I/O with asyncio
Now that we’ve seen how to establish a connection and perform basic read/write operations using asyncio streams, let’s explore how to implement more complex asynchronous I/O tasks. For instance, we might want to create a server that can handle multiple client connections simultaneously. This can be achieved by defining an async coroutine that listens for incoming connections and then processes each one in a separate coroutine.
import asyncio async def handle_client(reader, writer): data = await reader.read(100) message = data.decode() addr = writer.get_extra_info('peername') print(f"Received {message!r} from {addr!r}") print(f"Send: {message!r}") writer.write(data) await writer.drain() print("Close the client socket") writer.close() async def main(): server = await asyncio.start_server( handle_client, '127.0.0.1', 8888) addr = server.sockets[0].getsockname() print(f'Serving on {addr}') async with server: await server.serve_forever() asyncio.run(main())
In the above example, we define a coroutine handle_client
that reads data from the client, processes it, and sends a response. The server is created using the asyncio.start_server
function, which takes the handle_client
coroutine and the host and port to listen on. The async with
statement is used to manage the server’s lifecycle, ensuring it runs indefinitely with server.serve_forever()
until explicitly stopped.
It’s crucial to understand how to handle multiple concurrent connections without blocking. Asyncio’s event loop takes care of scheduling and executing the coroutines, allowing for simultaneous processing of clients. This concurrency is what gives asyncio streams their power and efficiency for networking tasks.
Another common task is implementing timeouts for I/O operations to avoid waiting indefinitely if a peer is unresponsive. You can use the asyncio.wait_for
function to set a timeout for any coroutine:
async def read_with_timeout(reader, timeout): try: return await asyncio.wait_for(reader.read(100), timeout) except asyncio.TimeoutError: print('Timeout! No data received.')
In this example, read_with_timeout
attempts to read data from the reader
within the specified timeout
period. If the timeout is reached, an asyncio.TimeoutError
is raised, and we can handle it as needed.
When implementing asynchronous I/O with asyncio, it is important to ponder in terms of coroutines and the event loop. This mental model allows you to write code that’s non-blocking and can scale to handle many I/O operations at once. With these concepts in mind, you can build robust and efficient networking applications using asyncio streams in Python.
Handling Errors and Exceptions
Handling errors and exceptions in asyncio streams is an important part of writing robust asynchronous applications. Just like with synchronous code, your async code can encounter a variety of exceptions, such as network errors, data parsing errors, and more. However, dealing with them in an asynchronous context requires some special considerations.
One of the most common patterns for error handling in asyncio is using try-except blocks around the code that might raise exceptions. For example, when working with StreamReader and StreamWriter objects, you should be prepared to handle I/O related exceptions:
async def handle_connection(reader, writer): try: data = await reader.read(100) # Process data ... except asyncio.IncompleteReadError as e: print(f'Incomplete read: {e.partial}') except ConnectionResetError: print('Connection reset by peer') except Exception as e: print(f'Unexpected exception: {e}') finally: writer.close() await writer.wait_closed()
In the code above, we handle specific exceptions that might occur during a read operation. asyncio.IncompleteReadError
is raised when the connection is closed before the requested amount of bytes is read. ConnectionResetError
indicates that the connection was closed by the peer. We also catch any other unexpected exceptions to ensure our program can recover gracefully.
Another important aspect of error handling in asyncio is ensuring that all resources are properly released, even in case of an error. That’s why we use the finally block to close the StreamWriter and wait for the closure to complete. This cleanup very important to prevent resource leaks that can degrade the performance of your application over time.
When dealing with timeouts, as mentioned in the previous section, you can use asyncio.wait_for
to wrap your coroutine calls. If a timeout occurs, you need to handle the asyncio.TimeoutError
exception:
async def read_with_timeout(reader, timeout): try: return await asyncio.wait_for(reader.read(100), timeout) except asyncio.TimeoutError: print('Timeout! No data received.') # Handle timeout, e.g., by retrying or closing the connection.
It’s also important to be aware that cancelling a coroutine with asyncio.CancelledError
is a valid and often necessary operation. For example, when shutting down your application, you may want to cancel all pending tasks:
tasks = [asyncio.create_task(coroutine()) for coroutine in coroutines] # At some later point, cancel all tasks for task in tasks: task.cancel() try: await asyncio.gather(*tasks) except asyncio.CancelledError: pass # Tasks were cancelled, so we can ignore this exception
In this scenario, we create a list of tasks and then cancel them all simultaneously. When awaiting the completion of these tasks with asyncio.gather
, we catch the asyncio.CancelledError
that is raised when a task is cancelled. This allows us to clean up our tasks without crashing our application.
By understanding and properly handling errors and exceptions, you can write more resilient asynchronous applications with asyncio streams. Remember to use try-except-finally blocks to catch and handle exceptions, manage timeouts appropriately, and cleanly cancel tasks when necessary. These practices will help ensure your application can handle unexpected events and maintain its stability and performance.
Best Practices for Working with asyncio Streams
Working with asyncio streams can significantly enhance the performance of your I/O-bound applications, but it is essential to follow some best practices to avoid common pitfalls and ensure your code is efficient and maintainable. Here are some tips to get the most out of asyncio streams:
-
Use context managers for resource management: When working with streams, it is crucial to ensure that you properly manage resources such as network connections. Python’s context managers can help with this. For example, you can use the
async with
statement when opening a connection, which will automatically close the stream when the block is exited, even in case of an error.async with asyncio.open_connection('127.0.0.1', 8888) as (reader, writer): # Perform I/O operations ...
-
Be cautious with buffer sizes: When reading from a stream, you can specify the buffer size. However, choosing a buffer size that is too small can lead to inefficient I/O operations, while a buffer size that’s too large can waste memory. It’s important to find a balance based on the expected data sizes you’ll be handling.
data = await reader.read(1024) # Read up to 1024 bytes
-
Handle backpressure: Backpressure occurs when you are sending data faster than it can be processed. To avoid overwhelming your system or the network, use the
await writer.drain()
method after writing to a stream. This will give the system time to process the buffer before more data is added.writer.write(data) await writer.drain()
-
Use tasks for concurrent operations: If you need to perform multiple I/O operations at once, you can use
asyncio.create_task
to run coroutines at the same time. This allows the event loop to manage the execution of these tasks efficiently.async def fetch_urls(urls): tasks = [asyncio.create_task(fetch(url)) for url in urls] await asyncio.gather(*tasks)
- Implement proper error handling: Always anticipate potential exceptions in your I/O operations. Use try-except blocks to catch and handle exceptions gracefully, and clean up resources as needed.
-
Avoid blocking operations: Ensure that all operations within your asyncio code are non-blocking. If you need to run blocking code, such as CPU-bound tasks or synchronous I/O, use
asyncio.to_thread
orasyncio.run_in_executor
to offload those tasks to a separate thread or process. -
Use
asyncio.wait_for
for timeouts: Prevent hanging operations by setting timeouts on your I/O tasks. This will raiseasyncio.TimeoutError
if the operation takes longer than expected, allowing you to handle the situation appropriately.try: await asyncio.wait_for(reader.read(100), timeout=5) except asyncio.TimeoutError: # Handle timeout ...
By following these best practices, you’ll be well on your way to writing high-performance, non-blocking network applications with asyncio streams. Remember to always consider the asynchronous nature of your I/O operations and the implications it has on resource management, error handling, and concurrency.
Source: https://www.pythonlore.com/implementing-asynchronous-i-o-with-asyncio-streams/