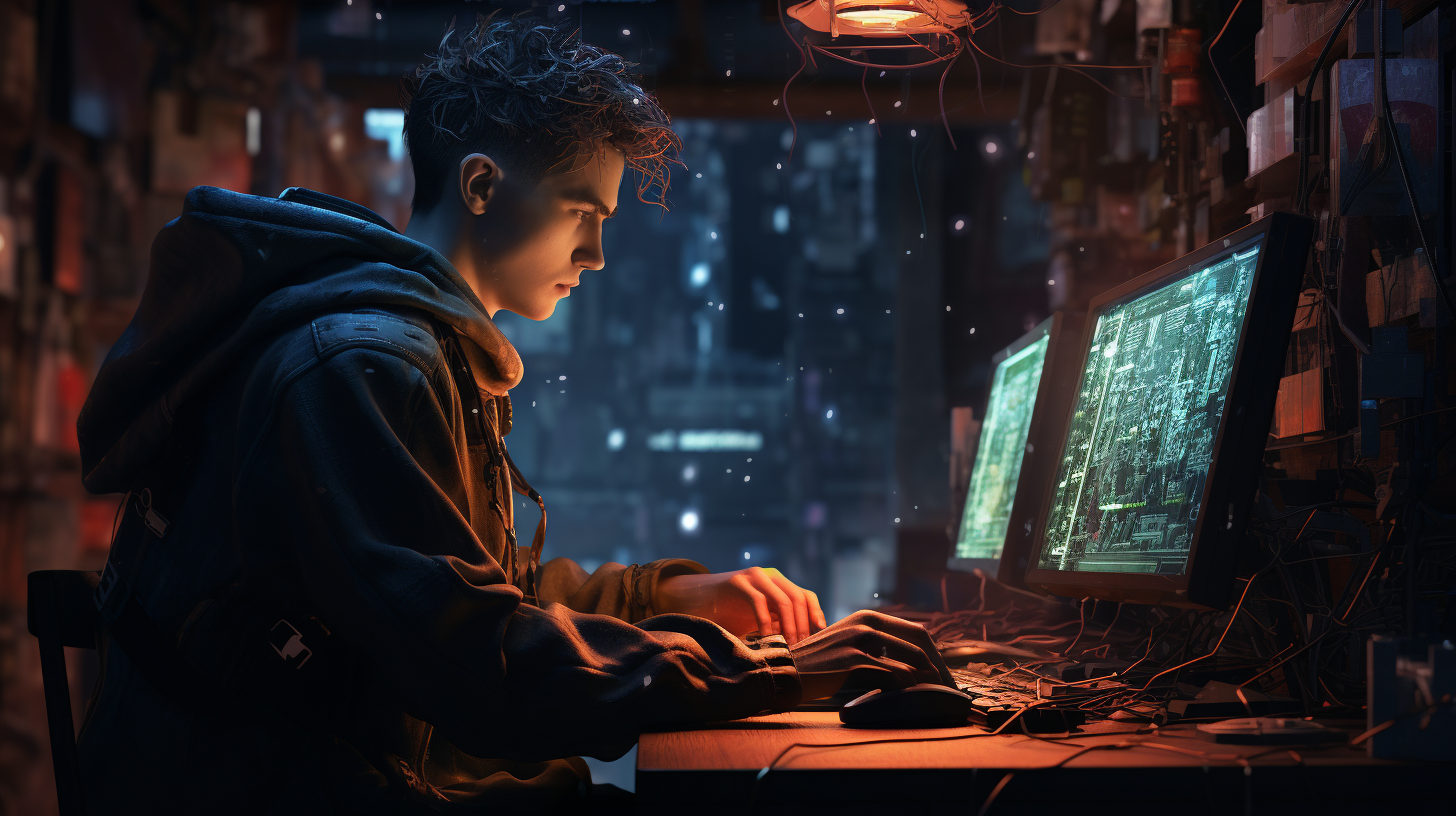
Overview of JavaScript Modules
JavaScript modules are a way to split up your code into separate, reusable pieces. They allow you to encapsulate functionality and expose it to other parts of your application as needed. Before the introduction of ES6, developers had to rely on third-party libraries or their own solutions for modularizing their code. However, with ES6, JavaScript now has a native module system that provides a standardized way to organize and share code between files.
Modules in JavaScript are defined using the export statement to make parts of the module available to other files, and the import statement to bring in functionality from other modules. This system is based on the ES6 module syntax, which is designed to be statically analyzable. This means that tools like bundlers and minifiers can optimize your code before it’s deployed.
Each module in JavaScript has its own scope, meaning that variables, functions, classes, etc., defined in a module are not visible outside the module unless they’re explicitly exported. This helps prevent naming conflicts and keeps your code organized.
- Modules can contain both code and declarations of variables, functions, and classes.
- Modules can be imported into other modules using the
import
statement. - Modules can export multiple values or a single default value.
Here’s a simple example of a module that exports a function:
// math.js export function sum(x, y) { return x + y; }
And here’s how you would import and use that function in another file:
// app.js import { sum } from './math.js'; console.log(sum(2, 3)); // Output: 5
It’s important to note that you can only use import and export statements inside modules, not regular scripts. To tell the browser that a script should be treated as a module, you need to include type="module"
in the script tag:
<script type="module" src="app.js"></script>
Modules are a powerful feature in JavaScript that help keep your code organized, maintainable, and easy to understand. By using the native module system provided by ES6, developers can create more structured and scalable applications.
Creating and Exporting Modules
To create a JavaScript module, you start by creating a new file which will contain the code you want to modularize. This file can contain any valid JavaScript code, including variable declarations, functions, and classes. Once you have your code written, you can export parts of it using the export
keyword.
There are two types of exports: named exports and default exports. Named exports allow you to export multiple values, while default exports let you export a single value. Let’s look at how to create and export modules using both methods.
Named Exports:
To create a named export, you simply prefix the declaration with the export
keyword:
// utils.js export const PI = 3.14159; export function calculateArea(radius) { return PI * radius * radius; } export class Circle { constructor(radius) { this.radius = radius; } getArea() { return calculateArea(this.radius); } }
In the above example, we export a constant, a function, and a class. Each of these can be imported individually in other modules using their named exports.
Default Exports:
If you want to export a single value or to have a “fallback” export, you can use a default export:
// greeting.js function greet(name) { return `Hello, ${name}!`; } export default greet;
Here, we have a function greet
that we export as the default export of the module. Unlike named exports, you can only have one default export per module.
It is also possible to combine named and default exports in the same module, but it is generally a good practice to use them separately to keep your module’s interface clean and clear.
Exporting an Existing Declaration:
If you have already declared a variable, function, or class, you can export it after the declaration using the following syntax:
// calculator.js function add(x, y) { return x + y; } function subtract(x, y) { return x - y; } export { add, subtract };
In this case, we export the add
and subtract
functions after they have been declared. That’s useful if you want to group your exports together at the end of the module.
Creating and exporting modules is a straightforward process in JavaScript with ES6. By using the export
keyword, you can share your code across different parts of your application, making it more maintainable and reusable.
Importing Modules
When you want to use functionality from another module, you need to import it into the current file. The import statement is used to read in the exports from a module and make them available for use. The basic syntax for importing a named export is:
import { name } from 'module-name';
Where name
is the named export you want to import, and module-name
is the file path to the module you are importing from. You can import as many named exports as you need by separating them with commas:
import { name1, name2 } from 'module-name';
If the module you are importing from has a default export, you can import it using the following syntax:
import defaultExport from 'module-name';
It is also possible to import both named and default exports at the same time:
import defaultExport, { name } from 'module-name';
If you want to import an entire module for side effects only, without importing any specific exports, you can use the following syntax:
import 'module-name';
Renaming imports can also be useful in cases where you might have naming conflicts or when you want to give an import a more descriptive name:
import { originalName as newName } from 'module-name';
Here’s an example of importing a named export and a default export from different modules:
// main.js import greet, { PI, calculateArea } from './greeting.js'; import { sum } from './math.js'; console.log(greet('World')); // Output: Hello, World! console.log('The value of PI is:', PI); console.log('The area of a circle with radius 5 is:', calculateArea(5)); console.log('The sum of 2 and 3 is:', sum(2, 3));
It’s important to remember that import statements are hoisted to the top of the module, so they’re processed before any code is executed. Additionally, imports are read-only views into the exported values, meaning that if the exported value changes in the module it was imported from, the imported value will also reflect that change.
Importing modules is a key aspect of working with JavaScript modules, as it allows you to break your code into smaller, more manageable pieces while still being able to access all the functionality you need.
Default Exports and Named Exports
In JavaScript modules, exports come in two flavors: default exports and named exports. Understanding the difference between these two and when to use each can help you write more readable and maintainable code.
Default Exports: As the name suggests, default exports are used to export a single value from a module. This could be a function, a class, an object, or any other valid JavaScript expression. Default exports are particularly useful when a module is designed to export a single main functionality.
// message.js function showMessage(msg) { console.log(msg); } export default showMessage;
To import a default export, you don’t need to use curly braces and you can name it whatever you want in the importing module:
// app.js import displayMessage from './message.js'; displayMessage('Hello, Default Export!');
Named Exports: Named exports are useful when a module exports multiple things. Each export has its own name and can be imported separately. You can have as many named exports as you like in a module.
// mathUtils.js export const add = (a, b) => a + b; export const subtract = (a, b) => a - b; export const multiply = (a, b) => a * b; export const divide = (a, b) => a / b;
To import named exports, you use curly braces to specify which exports you want to bring into your module:
// calculator.js import { add, subtract } from './mathUtils.js'; console.log(add(10, 5)); // Output: 15 console.log(subtract(10, 5)); // Output: 5
It’s also possible to import all named exports at once using the asterisk (*) as follows:
// calculator.js import * as MathUtils from './mathUtils.js'; console.log(MathUtils.add(10, 5)); // Output: 15 console.log(MathUtils.subtract(10, 5)); // Output: 5
One thing to remember is that you can mix default and named exports in a single module, but it’s generally considered best practice to stick to using one or the other for the sake of clarity and consistency in your codebase.
Choosing between default and named exports often depends on the structure and intended usage of your module. If a module represents a single entity or functionality, default exports make sense. On the other hand, if a module includes a collection of utilities or components, named exports provide the flexibility to import only what’s needed, potentially optimizing the final bundle size.
Ultimately, the decision on when to use default or named exports in JavaScript modules lies with the developer and the specific requirements of the project.
Advanced Module Techniques
Now that we’ve covered the basics of JavaScript modules and how to use the import and export syntax, let’s dive into some advanced module techniques that can help you write even more efficient and organized code.
Re-exporting
Sometimes, you might want to aggregate several modules and re-export their functionality from a single module. This can be particularly useful when creating a “barrel” file that simplifies the import paths for consumers of your modules. Here’s how you can re-export named exports from another module:
// utils/index.js export { add, subtract } from './mathUtils.js'; export { default as showMessage } from './message.js';
With this setup, other modules can import utilities from the utils
module without needing to know the internal structure of the utils
directory:
// app.js import { add, showMessage } from './utils/index.js'; console.log(add(2, 3)); // Output: 5 showMessage('Re-exporting is convenient!');
Dynamic Imports
Dynamic imports allow you to load modules on demand, which can be a great way to improve the performance of your application by splitting the code and only loading what’s needed at runtime. This is done using the import()
function, which returns a promise. Here’s an example:
// app.js button.addEventListener('click', async () => { const module = await import('./dynamicModule.js'); module.dynamicFunction(); });
Conditional Imports
With dynamic imports, you can also conditionally load modules based on certain criteria. This can be useful for feature toggles, polyfills, or loading different modules for different platforms:
// app.js if (someCondition) { import('./moduleA.js').then(module => { module.doSomething(); }); } else { import('./moduleB.js').then(module => { module.doSomethingElse(); }); }
Circular Dependencies
Circular dependencies occur when two or more modules import each other. This can sometimes lead to unexpected behaviors or errors. It’s best to avoid circular dependencies if possible, but if you must deal with them, make sure to design your modules so that the imported values are used after the modules have been initialized. For example:
// moduleA.js import { b } from './moduleB.js'; export function a() { console.log('Function a called'); b(); } // moduleB.js import { a } from './moduleA.js'; export function b() { console.log('Function b called'); // a(); // This would cause an error if called immediately } setTimeout(() => a(), 1000); // Delaying the call to avoid error
By using these advanced module techniques, you can create more dynamic, efficient, and well-organized JavaScript applications. Always remember to structure your modules thoughtfully and be mindful of potential pitfalls like circular dependencies.
Source: https://www.plcourses.com/javascript-modules-and-import-export/