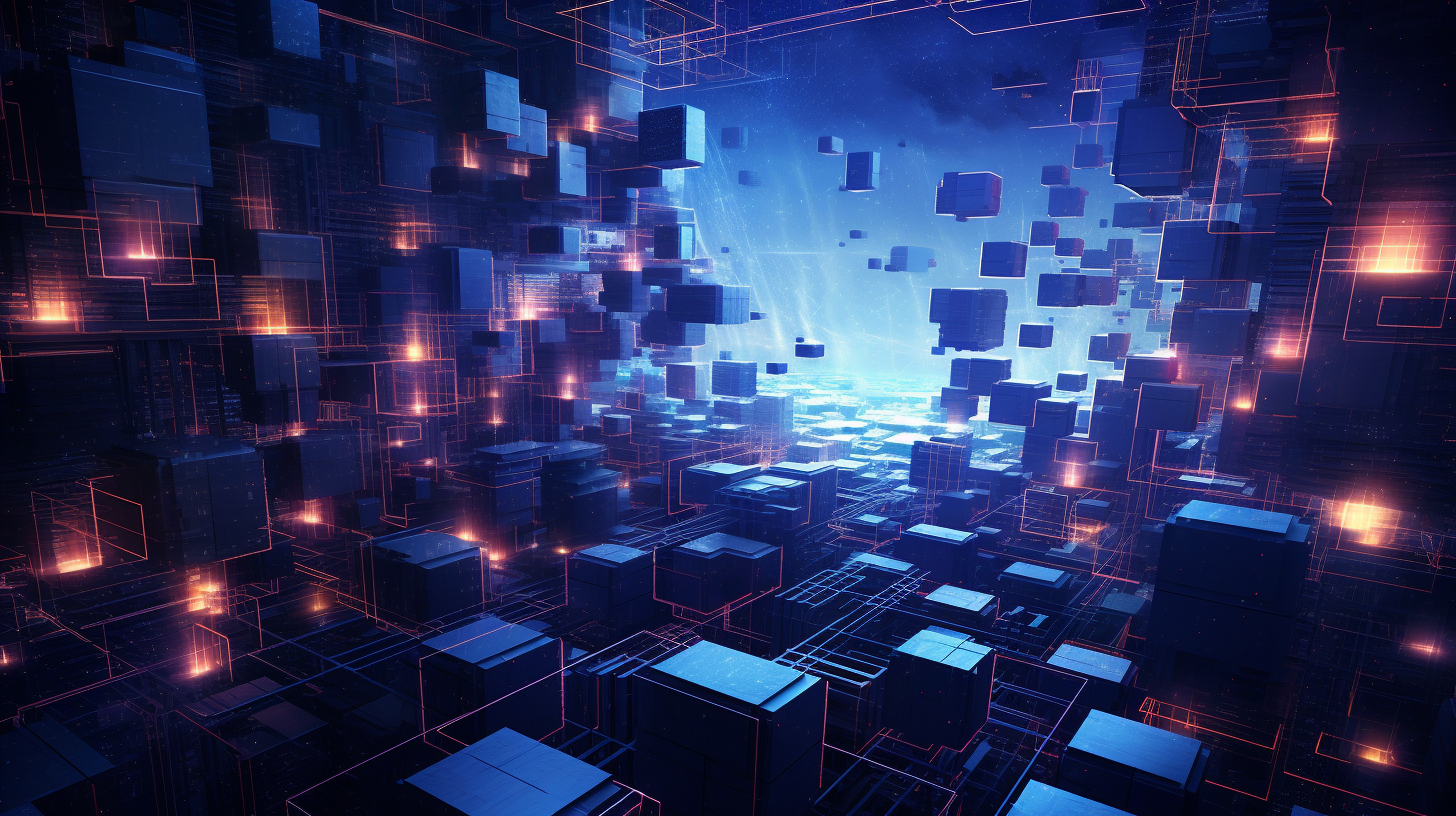
Introduction to Continuous Integration
Continuous Integration (CI) is a software development practice where members of a team integrate their work frequently, usually each person integrates at least daily – leading to multiple integrations per day. Each integration is verified by an automated build (including test) to detect integration errors as quickly as possible. This approach leads to significantly reduced integration problems and allows a team to develop cohesive software more rapidly.
One of the key benefits of continuous integration is that it allows developers to detect errors quickly, and locate them more easily. With the traditional approach, developers might work in isolation for an extended period and only merge their changes with the main branch once their work is complete. This can result in integration conflicts, hard-to-find bugs, and a delay in the release of the software.
With CI, developers integrate their changes into a shared repository using a version control system such as Git. After the code is pushed, an automated build system, such as Jenkins, runs a series of tests and checks to ensure that the new code does not break the build or introduce any new bugs.
Here is a simple Java code example that could be part of a CI process:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
This code could be committed to a shared repository, triggering a Jenkins build that compiles the code, runs any unit tests, and reports the results back to the team.
Continuous Integration is a development practice that requires developers to integrate code into a shared repository several times a day. Each check-in is then verified by an automated build, allowing teams to detect problems early. By integrating regularly, you can detect errors quickly, and locate them more easily.
Setting Up Jenkins for Java Projects
Setting up Jenkins for your Java projects is a straightforward process that will automate your build and testing processes, ensuring that your codebase remains stable and your team productive. The following steps will guide you through the installation and configuration of Jenkins, specifically tailored for Java projects.
Step 1: Install Jenkins
- Download Jenkins from the official website or use a package manager for your system.
- Run the Jenkins war file using the command
java -jar jenkins.war
. - //localhost:8080 in your browser and complete the installation wizard.
Step 2: Configure System Settings
- In the Jenkins dashboard, go to Manage Jenkins > Configure System.
- Set the JDK installations by providing the JAVA_HOME path.
- Configure your build tools such as Maven or Gradle if needed.
Step 3: Create a New Jenkins Job
- Click on New Item, enter a name for your project, and select Freestyle project.
- In the Source Code Management section, add your repository URL and credentials if necessary.
- Under Build Triggers, select the appropriate triggers for your project, like polling SCM or webhooks.
Step 4: Configure the Build
- In the Build section, add build steps such as Invoke top-level Maven targets for Maven projects or Execute shell for custom scripts.
- Set the goals to
clean install
for a full rebuild of the project.
Step 5: Add Post-build Actions
- Configure post-build actions like archiving artifacts, publishing JUnit test results, or sending notifications.
Example Build Script
mvn clean install
Once you have completed these steps, your Jenkins server will be ready to build and test your Java projects automatically. That’s a basic setup, and you can extend it by integrating with other tools such as code quality analyzers, artifact repositories, and more.
By setting up Jenkins for your Java projects, you’ll be able to automate your build and testing processes, which is an important aspect of Continuous Integration. Jenkins provides a robust and flexible platform that can be tailored to the unique needs of your Java project, ensuring that your team can focus on writing quality code and delivering features faster.
Integrating Additional Tools for Continuous Integration in Java
Integrating additional tools into your Continuous Integration pipeline can greatly enhance the effectiveness of your development process. These tools can range from code quality analyzers, artifact repositories, to deployment automation systems. In this section, we will discuss how to integrate some of these tools into your CI pipeline using Jenkins.
Code Quality Analyzers
-
One of the popular tools for Java is SonarQube, which provides detailed reports on code quality, code smells, and technical debt. To integrate SonarQube with Jenkins, you must first install the SonarQube Scanner plugin in Jenkins.
-
After installing the plugin, configure the SonarQube servers in Jenkins by navigating to Manage Jenkins > Configure System and adding your SonarQube server details.
-
In your Jenkins job configuration, add a new build step to execute SonarQube scanner, specifying your project key, name, and version.
Artifact Repositories
-
Artifact repositories like Nexus or Artifactory can be integrated to store the build outputs. For Maven projects, this can be done by configuring the
pom.xml
to include the repository URL and credentials.
<distributionManagement> <repository> <id>nexus-releases</id> <url>http://your-nexus-server/nexus/content/repositories/releases/</url> </repository> </distributionManagement>
Deployment Automation
-
Tools like Ansible, Chef, or Puppet can be used to automate the deployment process. These tools can be invoked from Jenkins jobs to deploy the build artifacts to various environments.
-
In Jenkins, use the “Execute shell” build step to run the deployment scripts or commands.
ansible-playbook -i inventory/production deploy.yml
By integrating these additional tools into your Jenkins pipeline, you can ensure that the code quality is maintained, artifacts are securely stored, and deployments are automated, resulting in a more efficient and reliable development process.
Source: https://www.plcourses.com/java-and-continuous-integration-jenkins-and-more/