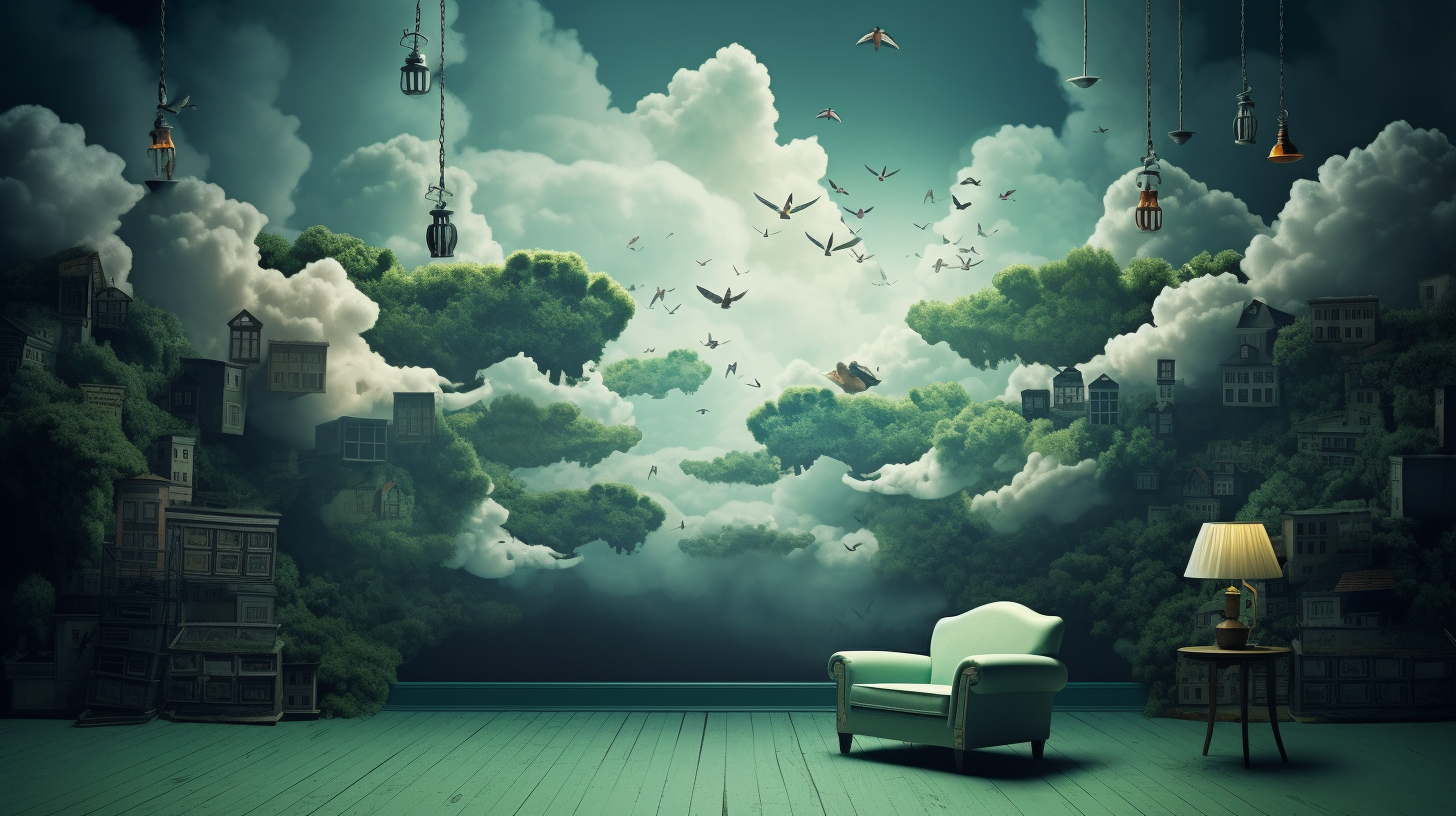
Introduction to Build Automation
Build automation is the process of automating the creation of a software build and the associated processes including compiling computer source code into binary code, packaging binary code, and running automated tests. In the context of Java, build automation tools are essential for any serious development effort.
Historically, developers compiled Java applications manually by executing the javac command. This approach is not only time-consuming but also prone to human error. Build automation tools help to eliminate these issues by providing a standardized way to build and test Java applications.
At its core, build automation involves the use of scripts or tools to perform tasks such as:
- Compiling source code into bytecode
- Managing project dependencies
- Executing unit tests and integration tests
- Packaging compiled code into JAR or WAR files
- Deploying applications to servers or repositories
One of the main advantages of build automation is that it enables continuous integration and continuous delivery practices. By automating the build and deployment process, teams can detect integration issues early, deliver updates faster, and reduce the risk of deployment failures.
Here’s a simple example of how a Java build automation script might look like:
// A simple build script using Apache Ant
<project name="HelloWorld" default="compile">
<target name="compile">
<javac srcdir="src" destdir="build/classes"/>
</target>
</project>
This script uses Apache Ant, one of the earlier build automation tools for Java, to compile Java source code from the src directory into the build/classes directory.
However, more state-of-the-art and sophisticated tools such as Maven and Gradle have since emerged. These tools offer more features and a more streamlined approach to Java build automation, which we will explore in the following sections.
Understanding Java Build Tools
When it comes to Java build tools, there are a few key players that have become industry standards due to their efficiency and feature-rich capabilities. Understanding these tools is important for any Java developer looking to implement build automation in their workflow.
Apache Ant is one of the oldest and most straightforward build tools. It uses XML to describe the build process and its dependencies. Ant scripts are procedural and require the developer to describe every step of the build process.
<target name="jar" depends="compile"> <jar destfile="dist/MyApp.jar" basedir="build/classes"> <manifest> <attribute name="Main-Class" value="com.mycompany.myapp.Main"/> </manifest> </jar> </target>
Apache Maven is a more advanced build tool that uses conventions for the build procedure and a project object model (POM) to manage project dependencies. Maven’s approach simplifies the build process by reducing the need for explicit configuration compared to Ant.
<dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> <scope>test</scope> </dependency>
Gradle is another popular build tool that combines the features of Ant and Maven. It introduces a Groovy-based domain-specific language (DSL) for describing builds, providing a balance between Ant’s flexibility and Maven’s convention-over-configuration.
apply plugin: 'java' repositories { mavenCentral() } dependencies { testCompile 'junit:junit:4.12' }
Each of these tools has its own strengths and weaknesses. Ant is highly customizable but can become unwieldy for larger projects. Maven is great for standard Java projects but can be restrictive due to its conventions. Gradle offers flexibility and a modern approach with its DSL, making it a powerful choice for many developers.
Understanding these tools and their differences is essential for choosing the right one for your project’s needs. As we will see in the next section, Maven, in particular, offers a set of features that make it a compelling choice for Java build automation.
Maven: Features and Benefits
Maven is a powerful build automation tool that is widely used in the Java development community. It offers a range of features and benefits that make it an attractive choice for developers looking to streamline their build process. Here are some of the key features and benefits of using Maven:
- Maven uses a standard directory layout and default build lifecycle which means developers spend less time writing and maintaining build scripts. The convention-over-configuration approach also makes it easier for new team members to understand the build process.
- Maven’s Project Object Model (POM) file describes the project’s dependencies and how they should be resolved and managed. Maven automatically downloads the required dependencies and includes them in the classpath.
- Maven provides archetypes which are project templates that can be used to create a new project structure within seconds. This not only speeds up project setup but also ensures consistency across projects in an organization.
- Maven has a rich set of plugins available that can extend its functionality and integrate with other tools and systems. This allows for a high level of customization and automation of various aspects of the build process.
- Maven’s build lifecycle comprises several phases such as compile, test, package, and install which are executed in a predefined sequence. This structured lifecycle makes the build process more predictable and easier to manage.
Let’s look at some examples of how these features are used in a typical Maven project:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.mycompany.app</groupId> <artifactId>my-app</artifactId> <version>1.0-SNAPSHOT</version> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> <scope>test</scope> </dependency> </dependencies> </project>
Here, the POM file declares a dependency on JUnit, which Maven will automatically download and include in the project’s build path. Additionally, by following Maven’s standard directory layout, the project’s source code and resources are organized in a way that Maven can automatically recognize and build without additional configuration.
The benefits of using Maven are clear: it simplifies the build process, reduces the potential for errors, ensures consistency across projects, and allows developers to focus more on writing code rather than managing builds. For these reasons, Maven has become a staple in many Java development workflows.
Implementing Maven in Java Projects
Implementing Maven in your Java projects can significantly improve your build process. This section will guide you through setting up Maven and using it to manage your Java project’s lifecycle. Let’s get started with the basic steps required to implement Maven.
Step 1: Installing Maven
Before you can start using Maven, you need to install it on your machine. You can download Maven from the official Apache Maven website. After downloading, simply extract the archive to a directory on your local machine and add the bin
directory to your PATH
environment variable.
Step 2: Creating a Maven Project
To create a new Maven project, you can use the mvn archetype:generate
command which will prompt you to choose an archetype. For a simple Java project, you might select the maven-archetype-quickstart
, which sets up a project with a standard directory layout and a sample pom.xml
file.
mvn archetype:generate -DgroupId=com.mycompany.app -DartifactId=my-app -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
Step 3: Understanding the POM File
The Project Object Model (POM) file, pom.xml
, is the core of a Maven project. It contains information about the project and configuration details used by Maven to build the project. Here’s a simple example:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.mycompany.app</groupId> <artifactId>my-app</artifactId> <version>1.0-SNAPSHOT</version> <dependencies> <dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-lang3</artifactId> <version>3.10</version> </dependency> </dependencies> </project>
Step 4: Building the Project
With Maven installed and a project created, you can build your project by running the mvn package
command from the root directory of your project. This will compile your code, run any tests, and package the compiled code into a JAR file.
mvn package
Step 5: Running Tests
Maven also simplifies the process of running tests. By convention, Maven expects your test classes to be in the src/test/java
directory. Tests can be run using the mvn test
command:
mvn test
Step 6: Managing Dependencies
One of Maven’s most powerful features is its ability to manage project dependencies. You can add a dependency to your pom.xml
file, and Maven will handle the downloading and inclusion of the dependency in your project’s classpath. For example, to add JUnit to your project:
<dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> <scope>test</scope> </dependency>
Step 7: Customizing the Build Lifecycle
Maven’s build lifecycle can be customized by adding plugins to the pom.xml
file. For example, if you want to create an executable JAR with dependencies, you can use the maven-assembly-plugin
:
<build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-assembly-plugin</artifactId> <configuration> <archive> <manifest> <mainClass>com.mycompany.app.App</mainClass> </manifest> </archive> <descriptorRefs> <descriptorRef>jar-with-dependencies</descriptorRef> </descriptorRefs> </configuration> <executions> <execution> <id>make-assembly</id> <phase>package</phase> <goals> <goal>single</goal> </goals> </execution> </executions> </plugin> </plugins> </build>
By following these steps and using the features of Maven, you can greatly improve the efficiency and reliability of your Java project’s build process. Maven’s convention-over-configuration approach, combined with its powerful dependency management and customizable build lifecycle, makes it an indispensable tool for modern Java development.
Source: https://www.plcourses.com/java-and-maven-build-automation/