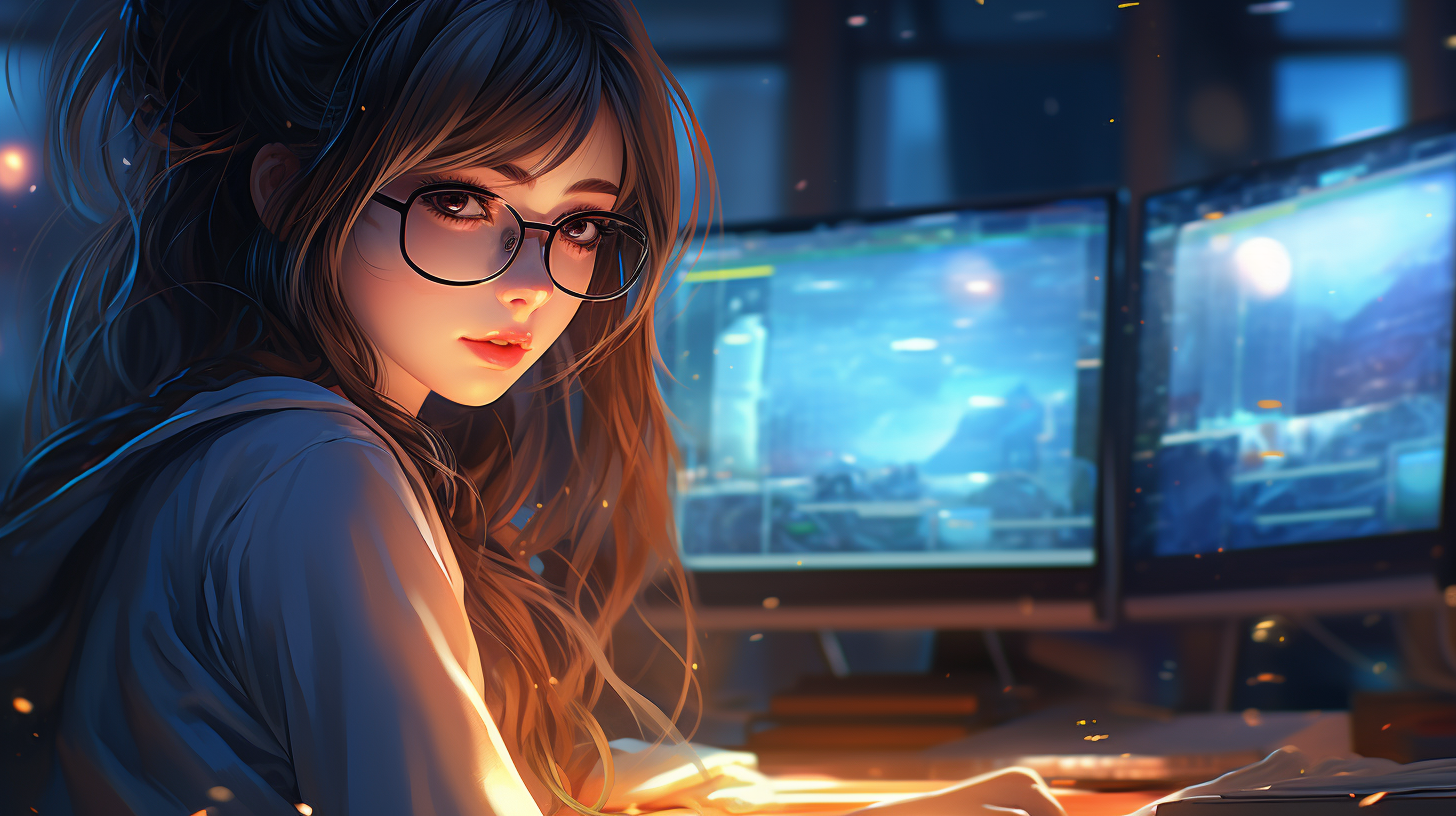
Introduction to User Interface Customization in Swift
User Interface (UI) customization in Swift allows developers to create unique and engaging experiences for users. Swift provides a robust set of tools and frameworks that enable developers to design and build custom interfaces that stand out from the crowd. Whether it’s customizing the look and feel of buttons, labels, or entire screens, Swift gives you the power to personalize your app’s UI to match your vision.
At the heart of UI customization in Swift is the UIKit framework. UIKit provides a wide array of classes and methods for managing and displaying content on the screen. By using UIKit, developers can modify existing UI elements or create entirely new ones from scratch. Moreover, with the introduction of SwiftUI, Apple’s declarative UI framework, customizing user interfaces has become more intuitive and efficient.
Why Customize Your UI?
- Custom UI elements can help reinforce your brand identity and make your app instantly recognizable.
- Tailoring the UI to your users’ needs can greatly enhance their experience and satisfaction with your app.
- A unique UI can set your app apart from competitors and capture the attention of potential users.
Getting Started with UI Customization
To begin customizing your UI in Swift, you’ll need to have a basic understanding of the following:
- UIKit components and how they interact with each other
- Auto Layout for responsive design across different screen sizes
- Asset management for images, icons, and other media
- SwiftUI for building state-of-the-art, declarative UIs (optional but recommended)
Here’s a simple example of how you can customize a UIButton in Swift using UIKit:
let customButton = UIButton(type: .system) customButton.setTitle("Click Me!", for: .normal) customButton.backgroundColor = .blue customButton.setTitleColor(.white, for: .normal) customButton.layer.cornerRadius = 10 customButton.layer.borderWidth = 2 customButton.layer.borderColor = UIColor.white.cgColor
And here’s an example using SwiftUI:
Button(action: { // Action to perform }) { Text("Click Me!") .padding() .background(Color.blue) .foregroundColor(.white) .cornerRadius(10) .overlay( RoundedRectangle(cornerRadius: 10) .stroke(Color.white, lineWidth: 2) ) }
Both examples create a blue button with white text, rounded corners, and a white border. However, the SwiftUI example showcases the framework’s declarative nature, making it easier to read and understand the UI code.
As you dive deeper into UI customization, you’ll discover that Swift provides a flexible and powerful platform for creating truly unique and engaging user interfaces. The possibilities are endless, and with the right tools and techniques, you can bring your creative vision to life in your iOS apps.
Customizing UI Elements in Swift
When it comes to customizing UI elements in Swift, developers have a plethora of options at their disposal. Let’s look at a few ways you can tailor UI components to fit your app’s design requirements.
Custom Fonts and Text Attributes:
Using custom fonts can significantly enhance your app’s visual allure. Here’s how you can set a custom font for a UILabel:
let label = UILabel() label.text = "Welcome to Swift!" label.font = UIFont(name: "YourCustomFontName", size: 18) label.textColor = .red
Styling Navigation Bars:
Navigation bars are crucial for navigation in many apps. Customizing them can improve user experience and maintain consistency with your branding. Here’s an example of customizing a navigation bar:
if let navigationBar = navigationController?.navigationBar { navigationBar.barTintColor = .purple navigationBar.tintColor = .white navigationBar.titleTextAttributes = [.foregroundColor: UIColor.white] }
Customizing Table Views:
Table views are common in iOS apps, and customizing them can make your app stand out. Here’s how you can customize the cells in a UITableView:
func tableView(_ tableView: UITableView, willDisplay cell: UITableViewCell, forRowAt indexPath: IndexPath) { cell.backgroundColor = .lightGray cell.textLabel?.textColor = .blue cell.selectionStyle = .none }
Additionally, you can use UIAppearance
to customize UI elements globally. For example, to set a global tint color for all instances of UIButton:
UIButton.appearance().tintColor = .green
Creating Custom Controls:
Sometimes, the standard UI components may not suffice, and you might need to create custom controls. Subclassing UI elements allows you to override their default behavior and appearance. Here’s a simple example of a custom slider:
class CustomSlider: UISlider { override func trackRect(forBounds bounds: CGRect) -> CGRect { var newBounds = super.trackRect(forBounds: bounds) newBounds.size.height = 10 // Custom track height return newBounds } }
With these examples, you can see how Swift and its frameworks provide a flexible environment for UI customization. Whether you are adjusting existing components or creating new ones from scratch, Swift has the tools you need to implement your creative designs.
Advanced Techniques for User Interface Customization
As we delve into the advanced techniques for user interface customization in Swift, it’s essential to understand that the more complex your UI needs, the more you’ll need to dip into custom rendering and layout. Let’s explore some of these advanced techniques that can take your UI customization to the next level.
Core Graphics and Custom Drawing:
Core Graphics is a powerful tool for custom drawing in Swift. It allows you to create complex shapes, gradients, and other graphical elements. You can use Core Graphics within the draw(_ rect: CGRect)
method of a UIView to render custom content. Here’s an example of drawing a custom circular progress bar:
class CircularProgressView: UIView { var progress: CGFloat = 0.0 { didSet { setNeedsDisplay() } } override func draw(_ rect: CGRect) { let center = CGPoint(x: rect.width / 2, y: rect.height / 2) let radius = min(rect.width, rect.height) / 2 let startAngle = -CGFloat.pi / 2 let endAngle = startAngle + 2 * CGFloat.pi * progress let progressPath = UIBezierPath(arcCenter: center, radius: radius, startAngle: startAngle, endAngle: endAngle, clockwise: true) progressPath.lineWidth = 10 UIColor.blue.setStroke() progressPath.stroke() } }
Custom Animations:
Animations can significantly enhance the user experience. Beyond the basic UIView animations, Core Animation provides a more granular control over the animation process. Here’s an example of a custom fade animation for a UIView:
func fadeInView(_ view: UIView, duration: TimeInterval = 1.0) { view.alpha = 0 UIView.animate(withDuration: duration, animations: { view.alpha = 1 }) }
Custom Gesture Recognizers:
Gesture recognizers are a great way to add interactivity to your app. By creating custom gesture recognizers, you can detect more complex user gestures. Here’s how you might implement a custom long-press gesture recognizer:
class CustomLongPressGestureRecognizer: UILongPressGestureRecognizer { override func touchesBegan(_ touches: Set, with event: UIEvent) { super.touchesBegan(touches, with: event) // Custom logic for long press } }
Custom Layouts with UICollectionView:
UICollectionView is a highly customizable component for presenting data in a grid or any other custom layout. By subclassing UICollectionViewLayout
, you can create entirely custom collection view layouts. Here’s a snippet for a custom layout:
class CustomCollectionViewLayout: UICollectionViewLayout { // Implement custom layout logic override func layoutAttributesForElements(in rect: CGRect) -> [UICollectionViewLayoutAttributes]? { // Return layout attributes for elements within the specified rect } }
Using Layer Masks for Complex UI Shapes:
CALayer masks can be used to create UI elements with complex shapes. By applying a mask to a view’s layer, you can control which parts of the view are visible. Here’s an example of masking a UIView to be circular:
func makeCircular(_ view: UIView) { let maskLayer = CAShapeLayer() maskLayer.path = UIBezierPath(ovalIn: view.bounds).cgPath view.layer.mask = maskLayer }
These advanced techniques for user interface customization in Swift provide a deeper level of control over how your app looks and feels. By mastering these methods, you can create an app that not only functions well but also has a stunning, memorable interface that truly reflects your creative vision.
Source: https://www.plcourses.com/swift-and-user-interface-customization/