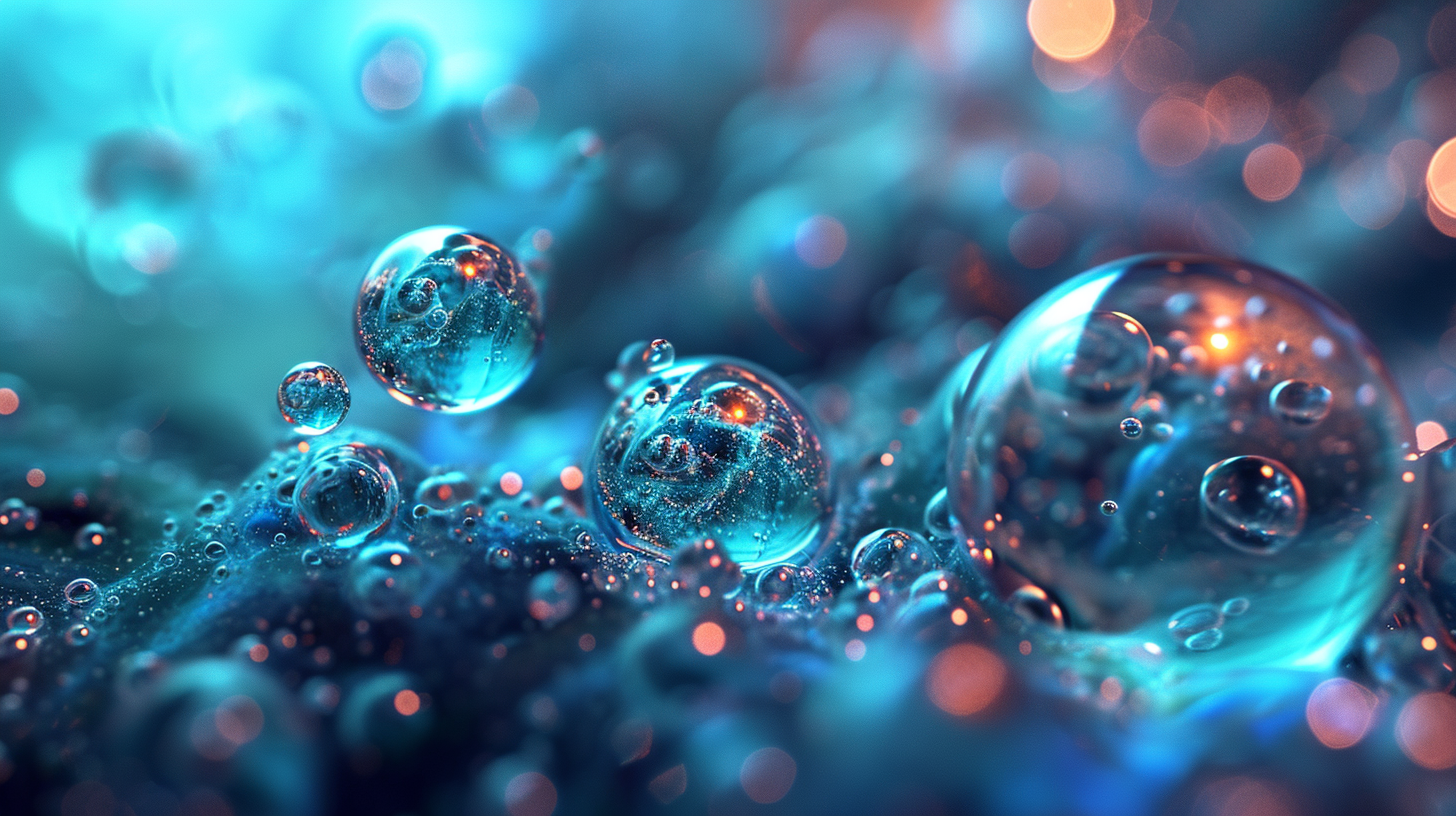
In the world of asyncio, protocols and transports are the fundamental building blocks that facilitate communication between different parts of an application or different applications altogether. Understanding these concepts is key to mastering the more advanced features of asyncio.
Protocols in asyncio are essentially factories for creating protocol instances. These instances define how to handle various events in the lifecycle of a connection. For instance, a protocol might define methods to handle connection establishment, data reception, and connection closure. A simple example of an asyncio protocol could look like this:
import asyncio class EchoProtocol(asyncio.Protocol): def connection_made(self, transport): self.transport = transport print('Connection established') def data_received(self, data): print('Data received:', data.decode()) self.transport.write(data) def connection_lost(self, exc): print('Connection closed') self.transport.close()
Transports, on the other hand, are abstractions over the underlying network or IPC mechanisms. They are responsible for sending and receiving data over the connection. Transports call into the associated protocol to process events like data reception or connection loss. In asyncio, transports can be created by loop methods such as loop.create_connection()
or loop.create_unix_connection()
.
Together, protocols and transports provide a high-level interface for performing I/O operations asynchronously. When a transport is created, it’s assigned a protocol instance which it can use to delegate event handling. This separation of concerns allows developers to focus on the logic for handling connection events in the protocol, without having to worry about the nitty-gritty details of the actual I/O.
For example, to use the EchoProtocol with a TCP server, you would do something like this:
loop = asyncio.get_event_loop() coro = loop.create_server(EchoProtocol, '127.0.0.1', 8888) server = loop.run_until_complete(coro) try: loop.run_forever() except KeyboardInterrupt: pass server.close() loop.run_until_complete(server.wait_closed()) loop.close()
This would start a TCP server on localhost, listening on port 8888, and for every incoming connection, an instance of EchoProtocol will be created to handle events for that connection.
Understanding the relationship between protocols and transports especially important when building complex networked applications with asyncio. With this knowledge, you’re well-equipped to start implementing custom protocols and transports, which we will cover in the following subsections.
Implementing Custom Protocols
Implementing custom protocols in asyncio involves defining a new class that inherits from asyncio.Protocol
. This class will need to implement several methods to handle different stages of a connection’s lifecycle. The main methods that you’ll typically need to implement are connection_made()
, data_received()
, and connection_lost()
.
Let’s create a simple custom protocol that acts as a server and responds with a greeting message to any incoming data:
import asyncio class GreetingProtocol(asyncio.Protocol): def connection_made(self, transport): self.transport = transport print('Server connected to client') def data_received(self, data): message = data.decode() print(f'Data received from client: {message}') response = f'Hello, {message}!' self.transport.write(response.encode()) def connection_lost(self, exc): print('The client closed the connection') self.transport.close()
With our GreetingProtocol
defined, we can now use it with an asyncio server just like we did with the EchoProtocol
. Here’s the code to start the server:
loop = asyncio.get_event_loop() coro = loop.create_server(GreetingProtocol, '127.0.0.1', 8888) server = loop.run_until_complete(coro) try: loop.run_forever() except KeyboardInterrupt: print('Server is shutting down') finally: server.close() loop.run_until_complete(server.wait_closed()) loop.close()
When a client connects to this server and sends a message, the server will respond with a greeting that includes the client’s message. That’s a simple example, but custom protocols can be as complex as needed, handling more sophisticated communication patterns and data processing.
It is also possible to handle errors and exceptions in your protocol. For example, if an exception occurs while receiving data, you might want to log the error and close the connection:
class RobustGreetingProtocol(GreetingProtocol): def data_received(self, data): try: super().data_received(data) except Exception as e: print(f'Error: {e}') self.transport.close()
By extending our original GreetingProtocol
and overriding the data_received
method, we can add a try-except block to handle any exceptions that occur during data processing. That’s a simple way to make your protocol more robust and error-resistant.
Implementing custom protocols gives you complete control over how your asyncio application communicates, so that you can optimize for your specific use case and achieve better performance and reliability.
Creating Custom Transports
Creating custom transports in asyncio involves diving a bit deeper into the library’s internals. Transports are responsible for the actual I/O operations, and by creating a custom transport, you can control how data is sent and received at a lower level than with protocols.
To create a custom transport, you generally need to subclass from asyncio.Transport
. You will need to implement several methods to handle sending and receiving data, as well as managing the transport’s state. Here’s a skeleton of how a custom transport class might look:
class CustomTransport(asyncio.Transport): def __init__(self, loop): self._loop = loop self._closed = False # Initialization code here def write(self, data): # Code to send data pass def close(self): # Code to close the transport self._closed = True def is_closing(self): return self._closed # Other necessary methods...
Let’s say we want to create a transport that logs all data sent and received for debugging purposes. We could create a LoggingTransport class like so:
class LoggingTransport(asyncio.Transport): def __init__(self, original_transport): self._original_transport = original_transport def write(self, data): print(f"Sending data: {data}") self._original_transport.write(data) def close(self): print("Closing transport") self._original_transport.close() # Delegate all other methods to the original transport def __getattr__(self, item): return getattr(self._original_transport, item)
This custom transport wraps an existing transport (like the one provided by loop.create_connection()
) and logs all data that passes through it. You can use it like this:
# Use EchoProtocol from the previous example loop = asyncio.get_event_loop() coro = loop.create_connection(lambda: EchoProtocol(), '127.0.0.1', 8888) transport, protocol = loop.run_until_complete(coro) # Wrap the transport with our custom logging transport logging_transport = LoggingTransport(transport) # Now use logging_transport instead of the original transport logging_transport.write(b'Hello World')
When you run this code, you should see the “Sending data” message logged before the data is sent through the original transport.
Custom transports can be very powerful, allowing you to implement features such as encryption, compression, or custom framing/deframing of your data. However, they also add complexity to your application, so they should be used judiciously and only when necessary.
By understanding and using asyncio’s protocols and transports, you can build highly efficient and scalable networked applications and services. Whether you are implementing a custom protocol, a custom transport, or both, asyncio provides the flexibility needed to meet the demands of state-of-the-art network programming.
Advanced Techniques for Handling Asynchronous I/O
As we dive deeper into advanced asyncio techniques, it’s important to explore how to handle asynchronous I/O operations more effectively. One such technique is the use of streams, which provide a higher-level, more Pythonic way of working with network connections.
Streams in asyncio are abstracted using two classes: StreamReader and StreamWriter. These classes provide a simpler interface for reading from and writing to connections and are built on top of protocols and transports. Here’s an example of how you might use streams:
import asyncio async def echo_client(reader, writer): data = await reader.read(100) # Read up to 100 bytes writer.write(data) await writer.drain() # Ensure data is sent writer.close() async def main(): server = await asyncio.start_server( echo_client, '127.0.0.1', 8888) async with server: await server.serve_forever() asyncio.run(main())
In this example, we’ve defined an echo_client
coroutine that reads data from the client, echoes it back, and then closes the connection. The main
coroutine starts the server and runs it indefinitely. The use of async with
ensures the server is properly cleaned up when the coroutine is done.
Another advanced technique in asyncio is using tasks to schedule the execution of coroutines at the same time. Tasks are used to run coroutines in the event loop and can be created using the asyncio.create_task()
function. Here’s how you might use tasks to handle multiple client connections simultaneously:
async def handle_client(reader, writer): # Handle client connection pass async def main(): server = await asyncio.start_server( handle_client, '127.0.0.1', 8888) async with server: while True: reader, writer = await server.accept() task = asyncio.create_task(handle_client(reader, writer)) # Now the server can handle other clients simultaneously asyncio.run(main())
In this updated example, each client connection is handled by creating a new task, allowing the server to manage multiple connections simultaneously without blocking.
Lastly, asyncio also supports using callbacks for handling I/O events. While the use of coroutines and tasks is generally preferred for their readability and ease of use, callbacks can be useful in certain situations. Here’s an example of how you might use callbacks with asyncio:
def on_data_received(data, future): print(f"Data received: {data}") future.set_result(data) async def main(): loop = asyncio.get_event_loop() reader, writer = await asyncio.open_connection('127.0.0.1', 8888) data = await loop.run_in_executor(None, writer.write, b'Hello World') future = loop.create_future() loop.add_reader(reader.fileno(), on_data_received, data, future) await future # Wait for data to be received asyncio.run(main())
In this callback example, we’re using loop.run_in_executor()
to write data to a connection and loop.add_reader()
to register a callback that’s called when data is received. The future
is used to wait for the callback to complete before continuing.
These advanced techniques demonstrate the flexibility and power of asyncio when it comes to handling asynchronous I/O. By mastering these patterns, you can write more efficient, scalable, and maintainable network applications.
Source: https://www.pythonlore.com/advanced-asyncio-implementing-protocols-and-transports/