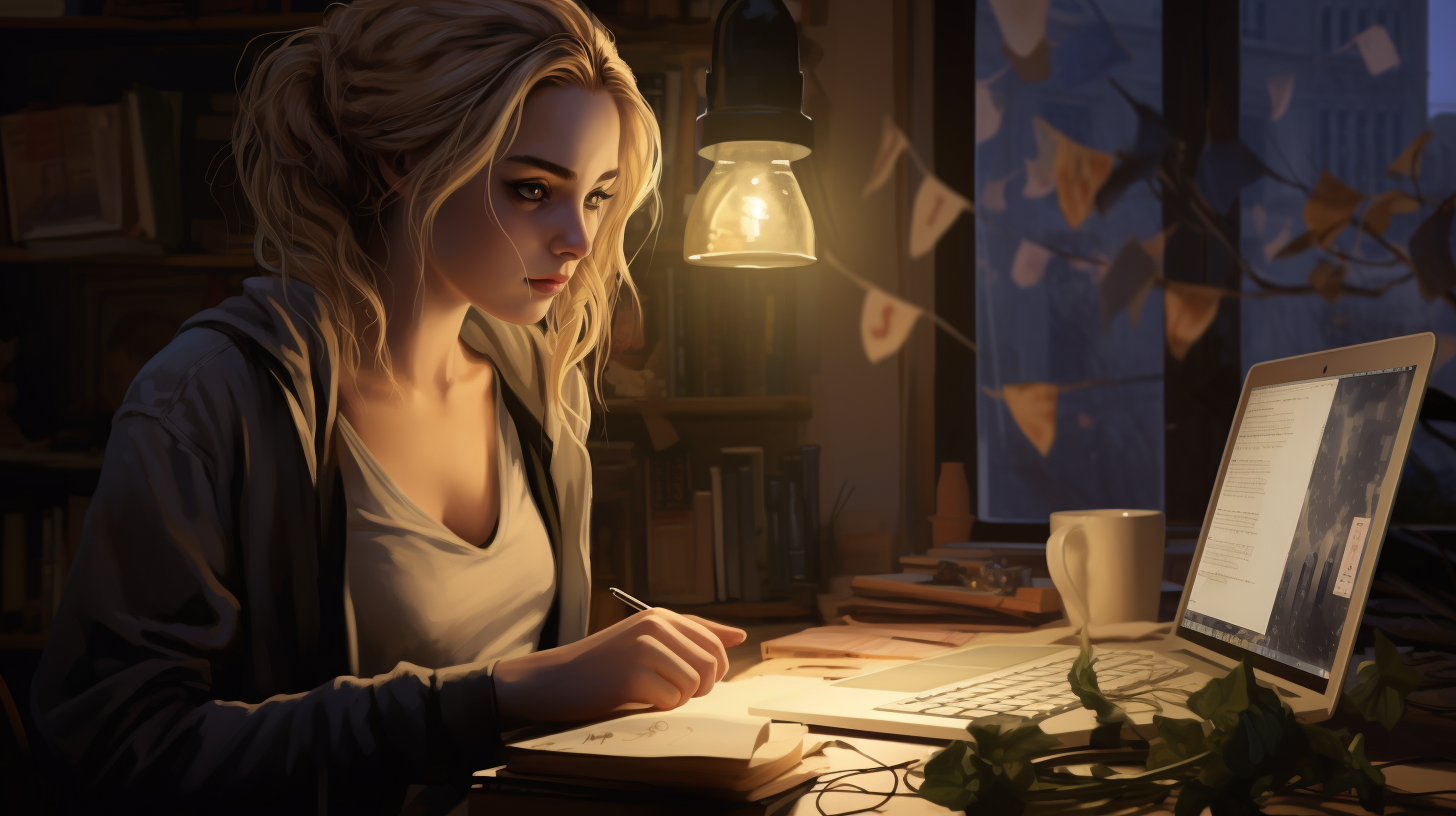
Loops are an essential part of any programming language. They allow us to repetitively execute a block of code until a specific condition is met. In Python, there are two main types of loops: the for loop and the while loop. In this tutorial, we will explore both of these loops in detail and understand their usage and differences.
The For Loop
The for loop is used to iterate over a sequence such as a list, string, or tuple. It executes a block of code for each item in the sequence.
Here is the basic syntax of a for loop:
for item in sequence: # code block to be executed
Let’s take a look at a simple example. Suppose we have a list of fruits and we want to print each fruit:
fruits = ['apple', 'banana', 'orange'] for fruit in fruits: print(fruit)
This will output:
apple banana orange
The loop begins by assigning the first item of the list to the variable fruit
. Then it executes the code block, which in this case is simply printing the value of fruit
. After that, it moves on to the next item in the list and repeats the process until there are no more items left.
We can also use the range() function with the for loop to iterate over a sequence of numbers:
for num in range(5): print(num)
This will output:
0 1 2 3 4
In this example, the range() function creates a sequence of numbers from 0 to 4. The for loop then iterates over this sequence, assigning each number to the variable num
and printing it.
The While Loop
The while loop is used to repeatedly execute a block of code as long as a certain condition is true. Unlike the for loop, it doesn’t require a sequence and instead relies on a condition.
Here is the basic syntax of a while loop:
while condition: # code block to be executed
Let’s take a look at an example. Suppose we want to count from 1 to 5 using a while loop:
count = 1 while count <= 5: print(count) count += 1
This will output:
1 2 3 4 5
The loop begins by checking if the condition count <= 5
is true. Since the initial value of count
is 1, the condition is true and the code block is executed. After printing the value of count
, we increment count
by 1 using the shorthand +=
operator. This process repeats until count
becomes 6, which breaks the loop since the condition is no longer true.
We can also use the break and continue statements to modify the behavior of a while loop. The break statement terminates the loop completely, while the continue statement skips the rest of the code block and moves to the next iteration.
Nesting Loops
It’s also possible to nest loops, where one loop is placed inside another loop. This allows us to perform more complex iterations. Let’s see an example where we nest a for loop inside a while loop:
i = 1 j = 1 while i <= 3: for j in range(3): print(i, j) j += 1 i += 1
This will output:
1 0 1 1 1 2 2 0 2 1 2 2 3 0 3 1 3 2
In this example, the while loop runs until i
becomes greater than 3. Inside the while loop, the for loop iterates through the numbers 0 to 2. So, for each iteration of the while loop, the for loop is executed three times, resulting in the output shown above.
The ability to nest loops provides great flexibility and allows us to solve a wide range of problems efficiently.
In this tutorial, we explored the two main types of loops in Python: the for loop and the while loop. We learned how to use them to iterate over sequences and perform repetitive tasks. We also saw how to nest loops to solve more complex problems. With this knowledge, you should now be able to effectively use loops in your Python programs.
Source: https://www.plcourses.com/looping-in-python-for-and-while-loops/