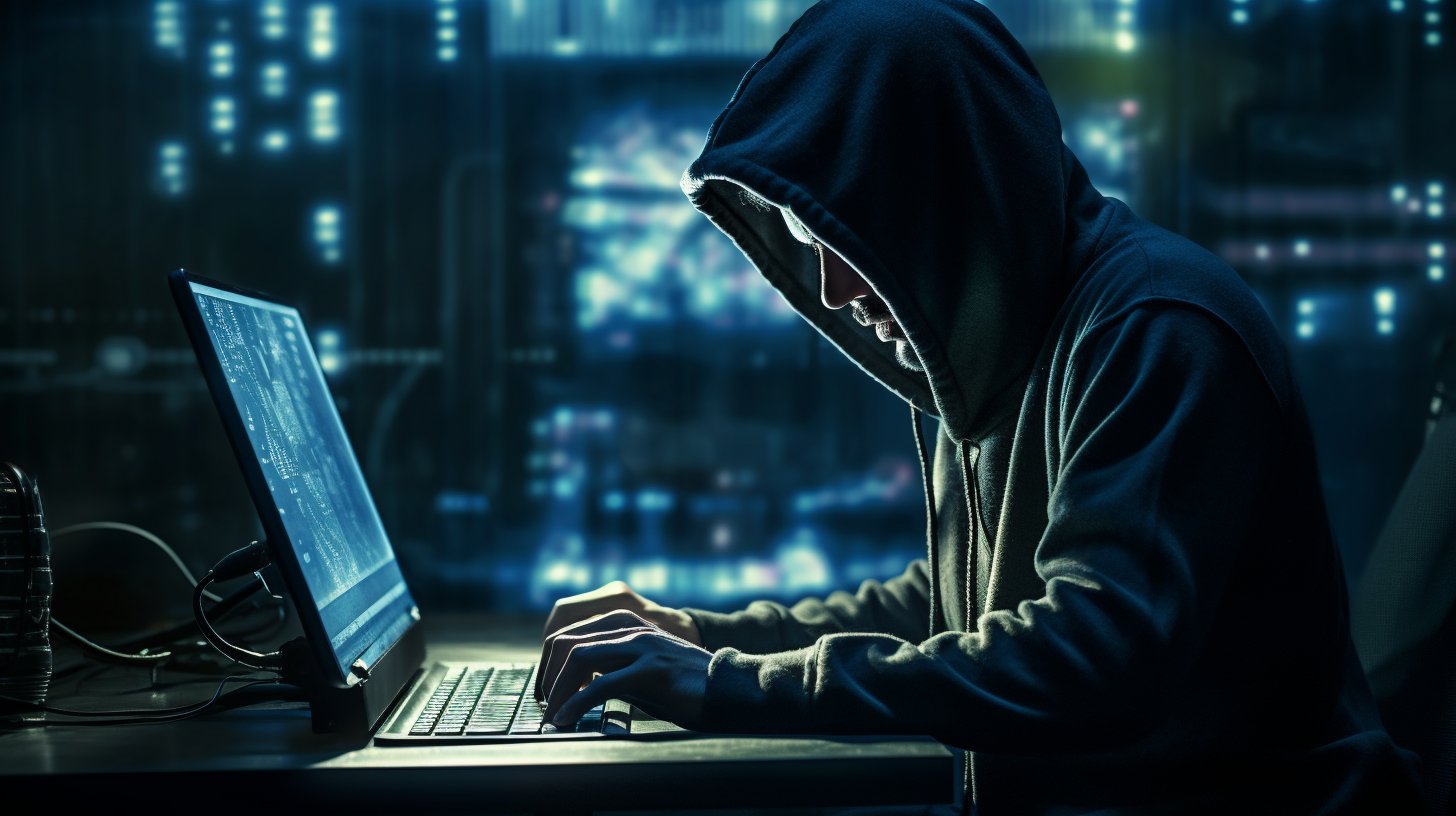
Introduction to Java Inheritance
Inheritance is one of the fundamental concepts of object-oriented programming (OOP) languages, and Java is no exception. It allows a class to inherit properties and methods from another class, enabling code reusability and the creation of a hierarchical relationship between classes. In Java, inheritance is achieved using the extends keyword.
When a class extends another, it inherits all the non-private members (fields and methods) from the parent class. This means that the child class can use the properties and methods of the parent class as if they were its own. However, the child class also has the option to override the methods of the parent class to provide its specific implementation.
Inheritance promotes the idea of code reusability and helps to create a natural hierarchy in the code architecture. It is one of the pillars that support the idea of polymorphism in Java.
Let’s take a look at a simple example of inheritance in Java:
class Vehicle { public void move() { System.out.println("The vehicle is moving"); } } class Car extends Vehicle { @Override public void move() { System.out.println("The car is moving"); } }
In the above example, Car
is a subclass (child class) that extends the Vehicle
superclass (parent class). The Car
class inherits the move
method from Vehicle
but provides its own implementation with the @Override
annotation. When an object of Car
calls the move
method, it will execute the overridden method in the Car
class, not the one in the Vehicle
class.
That is just a basic introduction to inheritance in Java. In the following sections, we will explore the different types of inheritance available in Java and provide more examples to illustrate how inheritance can be used in real-world programming scenarios.
Types of Inheritance in Java
In Java, there are four types of inheritance that are possible:
- That’s the simplest form of inheritance where a subclass inherits from only one superclass. It allows a class to inherit features from one parent class, thus forming a single inheritance hierarchy.
- Java does not support multiple inheritance with classes. This would be a scenario where a class can inherit features from more than one parent class. However, multiple inheritance can be achieved in Java using interfaces.
- This type of inheritance forms a multilevel class hierarchy where a class can be derived from a class which is itself derived from another class. In other words, a subclass inherits from a parent class which is also a subclass of another class.
- In this inheritance type, one class serves as a superclass for more than one subclass. It forms a hierarchy of class inheritance.
Let’s illustrate these concepts with code examples:
Single Inheritance:
class Animal { public void eat() { System.out.println("Animal is eating"); } } class Dog extends Animal { public void bark() { System.out.println("Dog is barking"); } }
Here, the Dog
class inherits from the Animal
class, forming a simple inheritance hierarchy.
Multi-level Inheritance:
class Animal { // Same as above } class Dog extends Animal { // Same as above } class Puppy extends Dog { public void weep() { System.out.println("Puppy is weeping"); } }
In this example, Puppy
is a subclass of Dog
, which is itself a subclass of Animal
. That is a multi-level inheritance scenario.
Hierarchical Inheritance:
class Animal { // Same as above } class Dog extends Animal { // Same as above } class Cat extends Animal { public void meow() { System.out.println("Cat is meowing"); } }
Here, both Dog
and Cat
classes inherit from the Animal
class, forming a hierarchy.
While multiple inheritance is not supported with classes in Java, it can be achieved using interfaces:
interface Animal { void eat(); } interface Mammal { void breathe(); } class Human implements Animal, Mammal { public void eat() { System.out.println("Human is eating"); } public void breathe() { System.out.println("Human is breathing"); } }
In this case, the Human
class implements two interfaces, Animal
and Mammal
, thus achieving multiple inheritance.
Understanding the types of inheritance in Java is important for designing robust and maintainable object-oriented applications.
Examples of Java Inheritance
Now that we’ve discussed the types of inheritance in Java, let’s delve into some real-world examples to demonstrate how inheritance can be effectively used in Java programming.
Example 1: Extending a Base Class
class Shape { public void draw() { System.out.println("Drawing a shape"); } } class Circle extends Shape { @Override public void draw() { System.out.println("Drawing a circle"); } } class Square extends Shape { @Override public void draw() { System.out.println("Drawing a square"); } }
In this example, we have a base class Shape
with a method draw()
. The subclasses Circle
and Square
extend the Shape
class and override the draw()
method to provide their specific implementations. This is a classic example of single inheritance where each subclass inherits from one superclass.
Example 2: Using Abstract Classes
abstract class Employee { protected String name; protected double salary; public Employee(String name, double salary) { this.name = name; this.salary = salary; } public abstract void work(); } class Developer extends Employee { public Developer(String name, double salary) { super(name, salary); } @Override public void work() { System.out.println(name + " is coding"); } } class Manager extends Employee { public Manager(String name, double salary) { super(name, salary); } @Override public void work() { System.out.println(name + " is managing the team"); } }
In this example, we have an abstract class Employee
that serves as a base class for Developer
and Manager
subclasses. The Employee
class has an abstract method work()
which is implemented by the subclasses. This is an example of hierarchical inheritance where multiple subclasses inherit from a single superclass.
Example 3: Implementing Interfaces
interface Flyable { void fly(); } class Bird implements Flyable { @Override public void fly() { System.out.println("Bird is flying"); } } class Airplane implements Flyable { @Override public void fly() { System.out.println("Airplane is flying"); } }
In this example, we have an interface Flyable
with a method fly()
. The classes Bird
and Airplane
implement the Flyable
interface and provide their own implementations of the fly()
method. This demonstrates how multiple inheritance can be achieved through interfaces in Java.
Inheritance in Java allows us to create a structured and reusable codebase. By using the power of inheritance, we can build complex systems with less code and easier maintenance. The examples provided above are just a starting point to understand how inheritance can be applied in Java programming. As you become more familiar with inheritance, you’ll be able to use it to design more efficient and scalable applications.
Source: https://www.plcourses.com/java-inheritance-basics-and-examples/