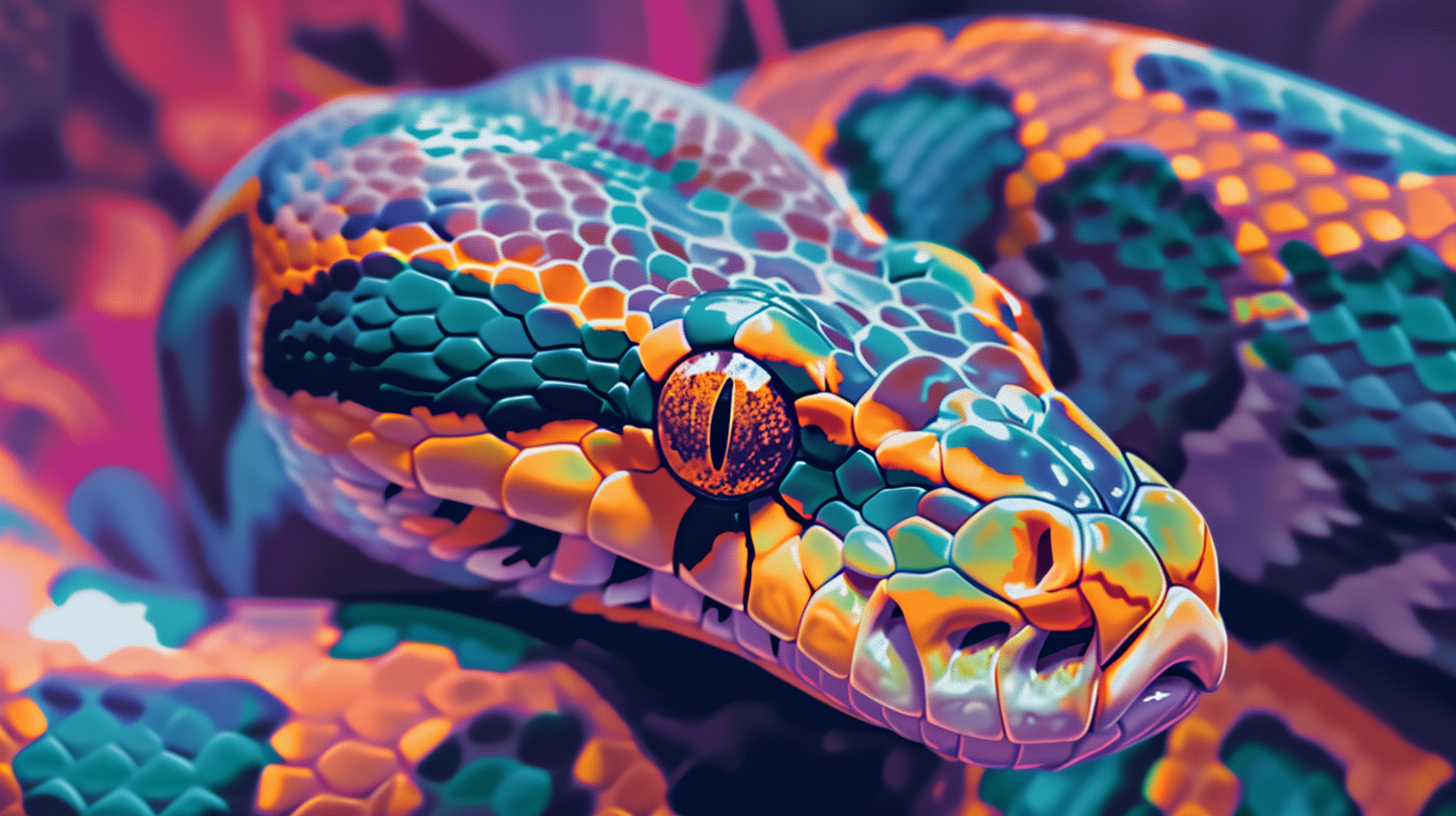
In Python, regular expressions are used for string searching and manipulation. For more efficient and convenient pattern matching, the re
module in Python provides a compile()
method, which compiles a regular expression pattern into a re.Pattern
object. A re.Pattern
object encapsulates the compiled version of a regular expression pattern.
Once you have a compiled re.Pattern
object, you can use it to perform various operations such as searching for matches, splitting strings, and replacing substrings. The re.Pattern
object provides several methods like match()
, search()
, findall()
, and finditer()
that allow you to apply the pattern to strings and work with the matches.
The main advantage of using a re.Pattern
object is performance. When you compile a regular expression, the Python interpreter converts it into a series of bytecodes that can be executed more efficiently. This means that if you’re going to use the same pattern multiple times, compiling it once and reusing the re.Pattern
object can result in significant performance improvements.
Here’s an example of how to create a re.Pattern
object:
import re # Compile a regular expression pattern into a re.Pattern object pattern = re.compile(r'bfoob')
In this example, the regular expression pattern bfoob (which matches the word “foo” as a whole word) is compiled into a re.Pattern
object and stored in the variable pattern
. Now you can use this pattern
to perform pattern matching operations on strings.
This introduction sets the stage for understanding how to create and use re.Pattern
objects for effective pattern matching in Python. In the following sections, we’ll dive deeper into creating compiled regular expressions, using re.Pattern
objects for pattern matching, exploring advanced features and methods, and discussing best practices.
Creating Compiled Regular Expressions
To create a compiled regular expression, you need to use the re.compile()
method from the Python re module. The compile()
method takes a string that represents your regular expression pattern as its argument. Optionally, you can pass various flags to modify the behavior of the pattern matching, such as re.IGNORECASE
for case-insensitive matching, re.MULTILINE
for multiline matching, and re.DOTALL
to make the dot match all characters including the newline.
import re # Compile a case-insensitive pattern pattern_insensitive = re.compile(r'some pattern', re.IGNORECASE) # Compile a multiline pattern pattern_multiline = re.compile(r'^start', re.MULTILINE) # Compile a pattern where dot matches newline pattern_dotall = re.compile(r'.+', re.DOTALL)
These flags can be combined using the bitwise OR operator (|
) if you need to apply more than one modification to the pattern matching.
import re # Combine IGNORECASE and MULTILINE flags combined_pattern = re.compile(r'some pattern', re.IGNORECASE | re.MULTILINE)
When you have special characters like in your pattern that also happen to be escape characters in Python strings, it’s often a good practice to use raw strings by prefixing the string with an
r
. This prevents Python from interpreting the backslashes as escape characters.
import re # Compile a pattern with special characters special_pattern = re.compile(r'd+.d*') # Matches a number with an optional decimal part
The compiled pattern can then be used to match against strings using various methods provided by the re.Pattern object, which we will discuss in the next section. By compiling regular expressions, especially those used frequently throughout your program, you can optimize your code for better performance.
Using re.Pattern Objects for Pattern Matching
Once you have your re.Pattern
object, you can start using it to match patterns within strings. The match()
method checks for a match only at the beginning of the string, while search()
scans through the whole string looking for a match. If you want to find all instances of the pattern, findall()
is the method to use, and finditer()
provides an iterator yielding match objects over all non-overlapping matches.
Here’s an example of using the match()
method:
import re # Compile a pattern pattern = re.compile(r'd+') # Use match() to search for a pattern at the beginning of the string result = pattern.match('123abc') if result: print('Match found:', result.group()) else: print('No match')
In this case, result.group()
will output ‘123’ as it is the first sequence of digits at the start of the string. If we had used pattern.match('abc123')
, it would return None, since there are no digits at the beginning of this string.
To search throughout the entire string, you would use search()
:
# Use search() to find a pattern anywhere in the string result = pattern.search('abc123') if result: print('Match found:', result.group()) else: print('No match')
This time, ‘123’ is found and outputted, as search()
examines the entire string.
Finding all matches is straightforward with findall()
:
# Use findall() to find all matches in the string result = pattern.findall('123 abc 456 def') print('Matches found:', result)
This will output a list: [‘123’, ‘456’].
For more detailed information on each match, including position, you can use finditer()
:
# Use finditer() to find all matches and get an iterator of match objects iterator = pattern.finditer('123 abc 456 def') for match in iterator: print('Match found:', match.group(), 'at position:', match.start())
This will print out each match along with its starting index in the original string.
These methods make re.Pattern
objects powerful tools for pattern matching in Python. By compiling your regular expressions into these objects, you can efficiently and conveniently search, analyze, and manipulate strings based on complex patterns.
Advanced Features and Methods of re.Pattern Objects
While the methods mentioned above cover most of the basic use cases, re.Pattern objects also offer advanced features that can be extremely useful in certain scenarios. One such feature is the groups() method, which is used when dealing with capturing groups in a pattern. Capturing groups are parts of the pattern enclosed in parentheses, which can capture the text of the substring matched by that part of the pattern.
import re # Compile a pattern with capturing groups group_pattern = re.compile(r'(d+)([a-z]+)') # Use search() to find a pattern with groups result = group_pattern.search('123abc') if result: print('Match found:', result.groups()) else: print('No match')
In this example, the result.groups()
will output a tuple: (‘123’, ‘abc’) as it captures the digits and letters separately.
Another advanced method is split(), which splits a string by occurrences of the pattern. If capturing groups are used in the pattern, then the text of all groups in the pattern are also returned as part of the resulting list.
# Use split() to split a string by pattern split_result = group_pattern.split('123abc456def') print('Split result:', split_result)
This will output: [”,’123′, ‘abc’, ‘456’, ‘def’, ”]. The empty strings at the beginning and end are included because there’s no text outside the split groups.
For replacing substrings, re.Pattern objects offer the sub() and subn() methods. The sub()
method replaces all occurrences of the pattern with a replacement string, while subn()
does the same but also returns the number of substitutions made.
# Use sub() to replace all matches with a string sub_result = group_pattern.sub('*', '123abc456def') print('Substitution result:', sub_result) # Use subn() to replace matches and get the number of replacements subn_result, num_subs = group_pattern.subn('*', '123abc456def') print('Substitution result:', subn_result) print('Number of substitutions:', num_subs)
This will output: Substitution result: * * and Number of substitutions: 2.
When working with re.Pattern objects, you may also want to access pattern attributes directly. The attributes pattern, flags, and groupindex hold the original pattern string, flags passed to the compile function, and a dictionary mapping group names to group numbers, respectively.
pattern.pattern
: The regex pattern string used to compile this re.Pattern object.pattern.flags
: The regex flags used for compiling this pattern.pattern.groupindex
: A dictionary mapping any named groups in the pattern to their corresponding group numbers.
These advanced features and methods add more power and flexibility to re.Pattern objects, enabling you to handle complex string processing tasks with ease.
Best Practices for Working with Compiled Regular Expressions
When working with compiled regular expressions, it’s important to follow best practices to ensure your code is efficient, readable, and maintainable. Here are some tips to help you make the most of re.Pattern objects:
- Compile once, use many times: If you’re using the same regular expression pattern multiple times in your code, compile it once and reuse the compiled re.Pattern object. This will save you the overhead of compiling the pattern each time you need it.
- Use raw strings for patterns: Always use raw strings (prefix with
r
) when defining regular expression patterns. This makes it clear that the string is meant to be taken as is, without interpreting backslashes as escape characters. - Choose the right method for matching: Be clear about which method to use for pattern matching. Use
match()
if you want to check only at the beginning of the string,search()
to scan through the entire string, andfindall()
orfinditer()
to retrieve all matches. - Use named groups for clarity: When your pattern includes multiple groups, use named groups (
(?P...)
) to make your code more readable and maintainable. - Avoid excessive backtracking: Some patterns can cause a lot of backtracking, which can slow down matching significantly. Try to write efficient patterns that minimize backtracking.
- Profile if necessary: If you’re working with very large texts or complex patterns and performance is a concern, profile your regular expressions to identify bottlenecks.
Here’s an example of using named groups in a compiled pattern:
import re # Compile a pattern with named groups named_group_pattern = re.compile(r'(?P<digits>d+)(?P<letters>[a-z]+)') # Use search() to find a pattern with named groups result = named_group_pattern.search('123abc') if result: print('Match found:', result.group('digits'), result.group('letters')) else: print('No match')
This will print ‘Match found: 123 abc’, making it clear which part of the match corresponds to digits and which to letters.
By following these best practices, you’ll be able to use re.Pattern objects effectively in your Python programs, making your pattern matching operations faster and your code cleaner and more professional.
Source: https://www.pythonlore.com/understanding-re-pattern-for-compiled-regular-expression-objects/