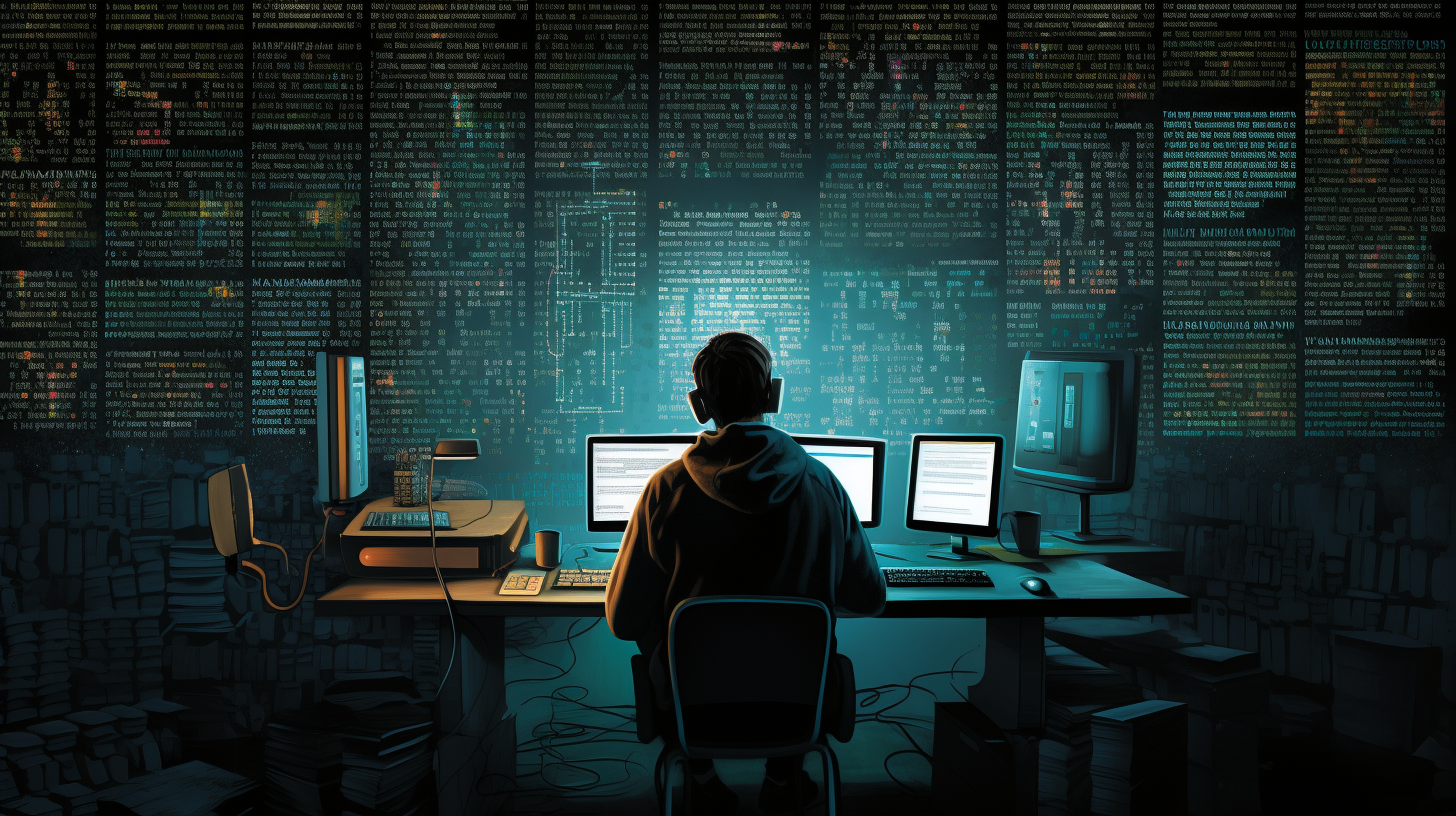
Introduction to Functions in Swift
Functions are one of the fundamental building blocks in Swift programming. They allow you to encapsulate a task or a piece of code that you want to reuse throughout your program. In Swift, functions are defined by the func keyword followed by a name, a set of parentheses, and a block of code.
func sayHello() { print("Hello, World!") }
Once a function is defined, you can call it by using its name followed by parentheses. For example, calling the sayHello
function would look like this:
sayHello() // Prints "Hello, World!"
Functions can also take parameters, which are values you pass into the function to customize its behavior. Parameters are specified within the parentheses after the function name.
func greet(name: String) { print("Hello, (name)!") }
To call a function with parameters, you include the values in the parentheses:
greet(name: "Alice") // Prints "Hello, Alice!"
Functions can also return values. The return type is specified after the arrow (->) following the parentheses.
func add(a: Int, b: Int) -> Int { return a + b } let sum = add(a: 5, b: 3) print(sum) // Prints "8"
Understanding how to define and use functions is essential for organizing and structuring your Swift code effectively. As we delve deeper into the syntax and parameters of functions in the following sections, we’ll explore more advanced concepts and techniques for working with functions in Swift.
Syntax and Parameters of Functions
In Swift, the syntax of a function is important as it defines how the function will be called and what it will do. The basic syntax includes the func keyword, the name of the function, a pair of parentheses, any parameters within those parentheses, and a block of code enclosed in curly braces. Here’s a simple example:
func printMessage() { print("This is a function") }
Parameters allow you to pass data into your function. They are defined within the parentheses of the function declaration, with each parameter consisting of a name and a type. You can have multiple parameters separated by commas. Here’s an example of a function with parameters:
func calculateArea(width: Double, height: Double) -> Double { return width * height }
To call this function, you would provide values for both width and height:
let area = calculateArea(width: 5.0, height: 3.0) print(area) // Prints "15.0"
Swift also supports default parameter values, which are used if no argument is provided for that parameter when the function is called. Default values are specified after the type of the parameter, using the assignment operator (=
). Here’s how you can define default values:
func greet(person: String, greeting: String = "Hello") { print("(greeting), (person)!") } greet(person: "Jane") // Prints "Hello, Jane!" greet(person: "John", greeting: "Hi") // Prints "Hi, John!"
Swift functions can also have variadic parameters, which accept zero or more values of a specified type. You use three periods (...
) after the type to indicate a variadic parameter. Here’s an example:
func sum(numbers: Int...) -> Int { return numbers.reduce(0, +) } let total = sum(numbers: 1, 2, 3, 4, 5) print(total) // Prints "15"
In addition to standard parameters, Swift functions can also have in-out parameters, which allow you to modify the values of variables passed from outside the function. In-out parameters are prefixed with the inout keyword. It’s important to note that when calling a function with in-out parameters, you must pass a variable as an argument rather than a constant or literal value, and you must prefix the argument with an ampersand (&
) to indicate that it can be modified by the function. Here’s an example:
func swapNumbers(a: inout Int, b: inout Int) { let temp = a a = b b = temp } var num1 = 10 var num2 = 20 swapNumbers(a: &num1, b: &num2) print("num1: (num1), num2: (num2)") // Prints "num1: 20, num2: 10"
Understanding the syntax and parameters of functions in Swift allows you to write more flexible and reusable code. In the next sections, we’ll continue to explore advanced concepts and techniques that will further enhance your ability to use functions effectively in your Swift projects.
Advanced Function Concepts and Techniques
As we dive into advanced function concepts and techniques in Swift, we’ll uncover the power of higher-order functions, explore the use of closures, and understand how to leverage function types. These concepts will enable you to write more sophisticated and modular code.
Higher-Order Functions
Higher-order functions are functions that can take other functions as arguments or return a function as a result. This can be particularly useful for creating reusable and flexible code. An example of a higher-order function is map
, which applies a function to each element in a collection.
let numbers = [1, 2, 3, 4, 5] let squaredNumbers = numbers.map { $0 * $0 } print(squaredNumbers) // Prints "[1, 4, 9, 16, 25]"
Closures
Closures are self-contained blocks of functionality that can be passed around in your code. They are similar to functions but have the ability to capture and store references to any constants and variables from the context in which they are defined. This makes them a powerful feature for tasks that require encapsulated functionality, such as completion handlers or callbacks.
let names = ["Chris", "Alex", "Ewa", "Barry", "Daniella"] let sortedNames = names.sorted(by: { (s1: String, s2: String) -> Bool in return s1 < s2 }) print(sortedNames) // Prints "["Alex", "Barry", "Chris", "Daniella", "Ewa"]"
Function Types
Every function in Swift has a type, consisting of the function’s parameter types and return type. You can use these types just like any other type in Swift, which makes it possible to assign functions to variables or pass them as arguments to other functions.
func addTwoInts(_ a: Int, _ b: Int) -> Int { return a + b } func multiplyTwoInts(_ a: Int, _ b: Int) -> Int { return a * b } var mathFunction: (Int, Int) -> Int = addTwoInts print("Result: (mathFunction(2, 3))") // Prints "Result: 5" mathFunction = multiplyTwoInts print("Result: (mathFunction(2, 3))") // Prints "Result: 6"
Using Function Types in Closures
You can also use function types as return types for closures. This allows you to create more dynamic and flexible code by returning different functions based on the context.
func chooseStepFunction(backward: Bool) -> (Int) -> Int { func stepForward(input: Int) -> Int { return input + 1 } func stepBackward(input: Int) -> Int { return input - 1 } return backward ? stepBackward : stepForward } var currentValue = 3 let moveNearerToZero = chooseStepFunction(backward: currentValue > 0) while currentValue != 0 { print("(currentValue)... ") currentValue = moveNearerToZero(currentValue) } print("zero!") // Prints "3... 2... 1... zero!"
By mastering these advanced function concepts and techniques, you’ll be able to write more expressive and powerful Swift code that can handle complex tasks with ease.
Source: https://www.plcourses.com/functions-in-swift/