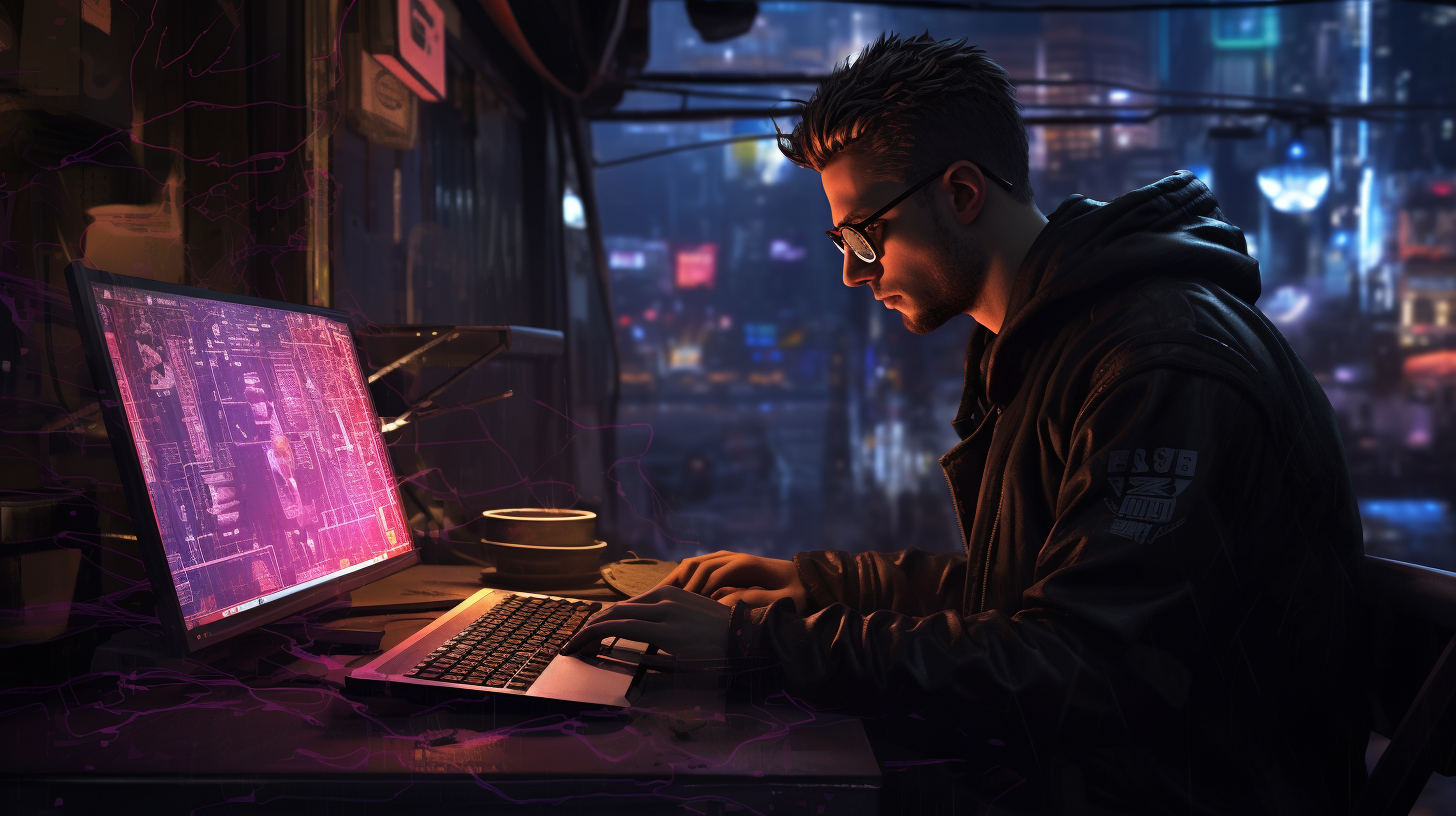
As a Python developer, managing dependencies is a important part of the development process. It can be challenging to keep track of the different packages and their versions required for a project. Python virtual environments provide a solution by allowing you to create isolated environments with their own set of dependencies.
A virtual environment is an isolated Python environment with its own Python interpreter and installed packages. This allows you to have multiple environments for different projects, each with its own set of dependencies. Virtual environments are particularly useful when working on projects that have conflicting package requirements or when you want to test your code with different versions of a package.
In this tutorial, you will learn how to create and manage virtual environments using Python’s built-in venv
module and how to install and manage dependencies using pip
.
Creating a Virtual Environment
The first step in managing dependencies with virtual environments is creating a new environment.
# Creating a virtual environment named 'myenv' python -m venv myenv
This command will create a new directory called myenv
that contains the necessary files for the virtual environment.
Activating the Virtual Environment
To start using the virtual environment, you need to activate it.
# On Windows myenvScriptsactivate # On Unix or Linux source myenv/bin/activate
After activation, your prompt will change, indicating that you are now working within the virtual environment.
Installing Packages with Pip
With the virtual environment activated, you can now install packages using pip
. Pip is a package manager for Python that allows you to easily install, upgrade, and remove packages.
To install a package, simply use the pip install
command followed by the name of the package.
pip install package_name
If you want to install a specific version of a package, you can specify it using the ==
operator.
pip install package_name==version_number
You can also specify a minimum version using the >=
operator.
pip install package_name>=version_number
Managing Dependencies
Pip makes it easy to manage dependencies within a virtual environment. When you install a package, pip will automatically install any required dependencies as well.
You can use the pip freeze
command to generate a list of installed packages and their versions.
pip freeze
This will output a list of packages and their versions in the format package_name==version_number
. You can redirect this output to a file to save the dependencies.
pip freeze > requirements.txt
You can then share this file with others working on the project, allowing them to easily install the same set of dependencies.
To install dependencies from a requirements file, you can use the pip install -r
command followed by the path to the file.
pip install -r requirements.txt
Deactivating the Virtual Environment
To deactivate the virtual environment and return to the global Python environment, you can use the deactivate
command.
deactivate
With this, you now have a solid understanding of virtual environments and how to manage dependencies using Python.
Python virtual environments are an essential tool for managing dependencies in your projects. They allow you to create isolated environments and easily manage package installations. By following the steps outlined in this tutorial, you can take advantage of Python’s venv
module and pip
package manager to create and manage your virtual environments effectively.
Source: https://www.plcourses.com/python-virtual-environments-managing-dependencies/