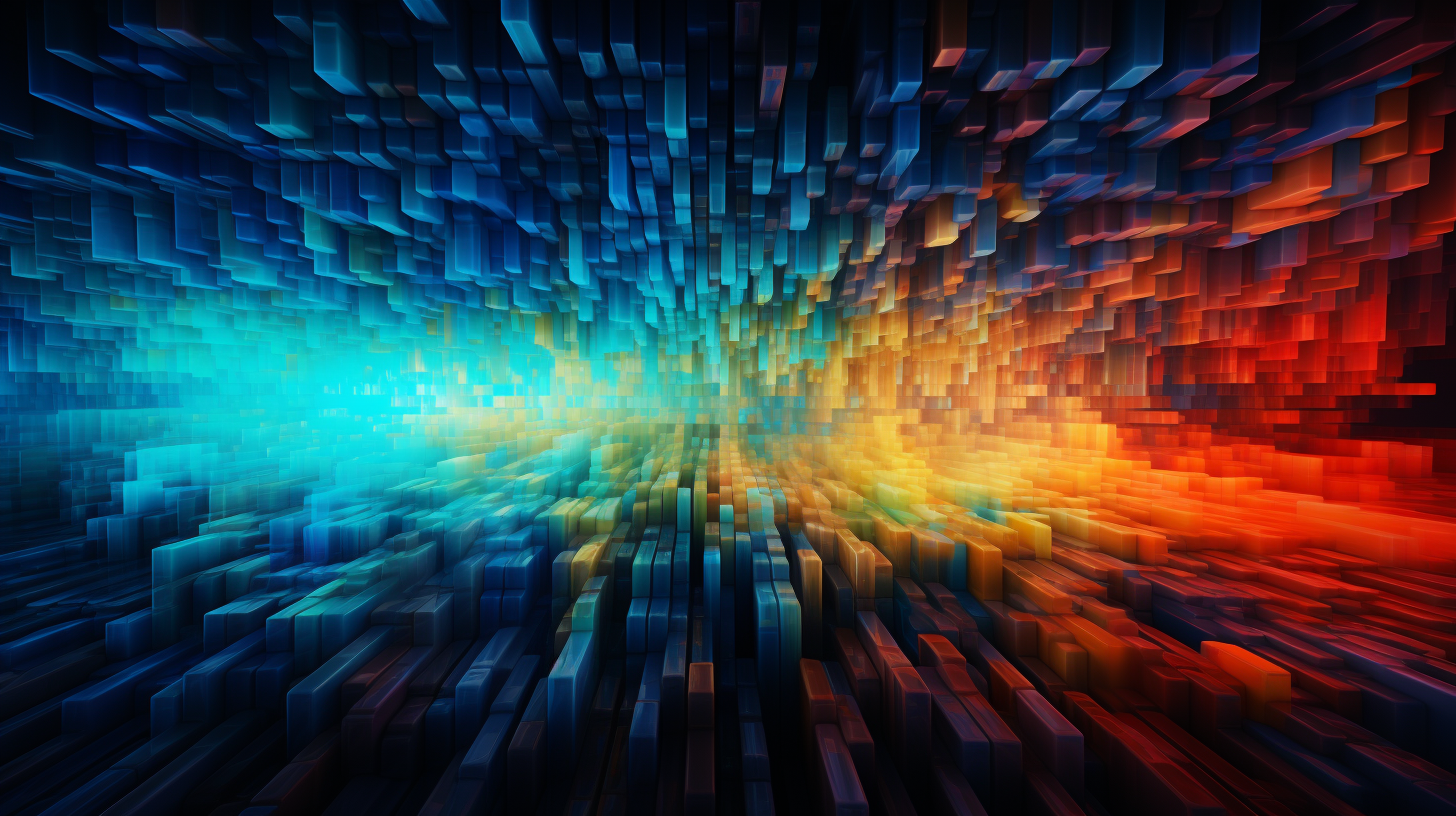
In Flask, the request object is a important component that allows you to access incoming request data such as form data, query parameters, JSON payloads, and file uploads. It acts as a global object that Flask creates automatically for each incoming request, and it contains various attributes and methods that you can use to interact with the request data.
The Flask request object is an instance of the flask.Request class and is part of the flask module. It’s a context-local object, which means that it is specific to the current thread handling the request. Flask handles this internally, so you don’t have to worry about managing context or threading issues when accessing the request object.
To understand how to work with the Flask request object, it’s important to know some of its most commonly used attributes:
- request.method: This attribute returns a string representing the HTTP method used in the request (e.g., ‘GET’, ‘POST’, ‘PUT’, ‘DELETE’).
- request.args: A MultiDict containing parsed query string parameters.
- request.form: A MultiDict containing form data submitted in a POST or PUT request.
- request.values: A CombinedMultiDict that combines the contents of request.args and request.form.
- request.json: Returns the JSON payload of the request, if it exists, or None otherwise. It internally uses json.loads() to parse the request data.
- request.files: A MultiDict containing any files uploaded as part of the request.
- request.headers: A dictionary-like object containing all HTTP headers sent with the request.
- request.cookies: A dictionary-like object containing all cookies sent with the request.
Here’s an example of how you might access some of these attributes in a Flask route:
from flask import Flask, request app = Flask(__name__) @app.route('/submit', methods=['GET', 'POST']) def submit(): if request.method == 'POST': # Access form data username = request.form['username'] password = request.form['password'] # Access JSON data json_data = request.json # Access file uploads uploaded_file = request.files['file'] # Process the data... # Always return a response return 'Form submitted' if __name__ == '__main__': app.run(debug=True)
A key point to remember is that you should never attempt to access the request object outside of a Flask route or view function context. Doing so will result in a RuntimeError, as the request object is only valid while handling a request.
By understanding these fundamental aspects of the Flask request object, you’ll be better equipped to handle incoming request data and build robust web applications with Flask.
Retrieving Data from the Request Object
When working with the Flask request object, it’s essential to understand how to retrieve various types of data sent by the client. Depending on the nature of your web application, you might need to handle different kinds of data, such as query parameters, form data, JSON payloads, or file uploads.
To retrieve query parameters from the URL, we use request.args
. For example, if a client sends a GET request to /search?q=flask
, you can access the value of ‘q’ using:
search_term = request.args.get('q', '')
For form data submitted via POST or PUT requests, you can use request.form
. Here’s an example of retrieving form data:
@app.route('/login', methods=['POST']) def login(): username = request.form['username'] password = request.form['password'] # Perform login logic... return 'Login Successful'
If you’re dealing with JSON data, which is common in API development, you can use request.json
to get the JSON payload. Here’s how:
@app.route('/api/data', methods=['POST']) def process_json(): json_data = request.json # Work with JSON data... return 'JSON Received'
For handling file uploads, Flask provides request.files
. You can retrieve uploaded files like this:
@app.route('/upload', methods=['POST']) def upload_file(): if 'file' in request.files: file = request.files['file'] # Save or process the file... return 'File Uploaded' else: return 'No file uploaded'
It’s important to note that for the request.files
attribute to work, your HTML form must have enctype="multipart/form-data"
set.
Finally, you might also need to access request headers or cookies. This can be done using request.headers
and request.cookies
, respectively. Here’s an example of accessing a specific header and a cookie:
user_agent = request.headers.get('User-Agent') session_id = request.cookies.get('session_id')
By learning how to retrieve different types of data from the Flask request object, you can handle virtually any kind of client input in your Flask applications.
Handling Different Types of Incoming Request Data
When dealing with incoming request data in Flask, it’s important to be able to handle different types of data that a client might send. The Flask request object provides various methods and attributes to access this data, whether it’s query parameters, form data, JSON payloads, or files. Here are some detailed examples of how to handle these different types of data.
Handling Query Parameters
To access query parameters, we use the request.args
attribute. That is particularly useful when you have a search functionality in your application. For instance:
@app.route('/search') def search(): query = request.args.get('query') # Use the 'query' parameter to perform search... return 'Results for {}'.format(query)
Handling Form Data
When a client submits form data via POST or PUT requests, you can retrieve this data using request.form
. Here’s an example of a login function that accesses form data:
@app.route('/login', methods=['POST']) def login(): username = request.form.get('username') password = request.form.get('password') # Authenticate user... return 'Welcome, {}'.format(username)
Handling JSON Payloads
If your application is an API or handles AJAX requests, you might receive JSON payloads. To access this data, you use request.json
, which parses the JSON into a Python dictionary:
@app.route('/api/users', methods=['POST']) def create_user(): user_data = request.json # Create new user with JSON data... return 'User created', 201
Handling File Uploads
File uploads are accessed through request.files
. This attribute holds a MultiDict where each key is the name of a file field in your form and each value is a Werkzeug FileStorage object:
@app.route('/upload', methods=['POST']) def upload_file(): uploaded_file = request.files['file'] if uploaded_file: filename = secure_filename(uploaded_file.filename) uploaded_file.save(os.path.join('/path/to/upload/folder', filename)) return 'File uploaded successfully' return 'No file uploaded'
In the above example, we also use the secure_filename()
function from Werkzeug utility to sanitize the filename before saving it.
Handling Headers and Cookies
Sometimes you may need to access request headers or cookies. This can be done using request.headers
and request.cookies
. Here is how you can access a specific header and cookie:
@app.route('/user') def get_user(): token = request.headers.get('Authorization') user_id = request.cookies.get('user_id') # Retrieve user with 'user_id' and verify 'token'... return 'User profile'
By understanding how to retrieve different types of incoming request data using the Flask request object, you’ll be able to build more dynamic and flexible web applications that can respond to various client-side actions.
Advanced Techniques for Working with the Flask Request Object
As you become more comfortable working with the Flask request object, you’ll want to explore some advanced techniques for managing incoming request data. These techniques can help you write cleaner, more efficient code and provide better error handling and validation for your web applications.
One such technique is using Flask request hooks. These are decorators that allow you to run code before or after each request, regardless of which route is being executed. The most commonly used hooks are before_request
, after_request
, and teardown_request
.
@app.before_request def before_request_func(): # Code to run before each request @app.after_request def after_request_func(response): # Code to run after each request return response @app.teardown_request def teardown_request_func(error=None): # Code to run when tearing down the request, even if an exception occurred
Another advanced technique involves custom request processing. Sometimes, you may want to pre-process request data before it reaches your route handler. For this, you can create a custom subclass of flask.Request
and override its methods to add custom behavior.
from flask import Flask, Request class CustomRequest(Request): def on_json_loading_failed(self, e): # Custom behavior for handling JSON loading errors super(CustomRequest, self).on_json_loading_failed(e) app = Flask(__name__) app.request_class = CustomRequest
In the example above, we’ve overridden the on_json_loading_failed
method to provide custom error handling when Flask fails to load JSON data from the request.
Request data validation is another critical area where advanced techniques come into play. One way to validate incoming data is by using Flask extensions like Flask-WTF or marshmallow. These libraries provide powerful validation and serialization tools for Flask applications.
from flask_wtf import FlaskForm from wtforms import StringField, validators class LoginForm(FlaskForm): username = StringField('Username', [validators.Length(min=4, max=25)]) password = StringField('Password', [validators.Length(min=6)]) @app.route('/login', methods=['POST']) def login(): form = LoginForm() if form.validate_on_submit(): # Form data is valid return 'Login Successful' else: # Form data is invalid return 'Invalid Credentials', 400
By implementing these advanced techniques, you’ll have more control over how incoming request data is handled and be able to build more robust and secure Flask applications.
Source: https://www.pythonlore.com/working-with-flask-request-object-for-incoming-request-data/