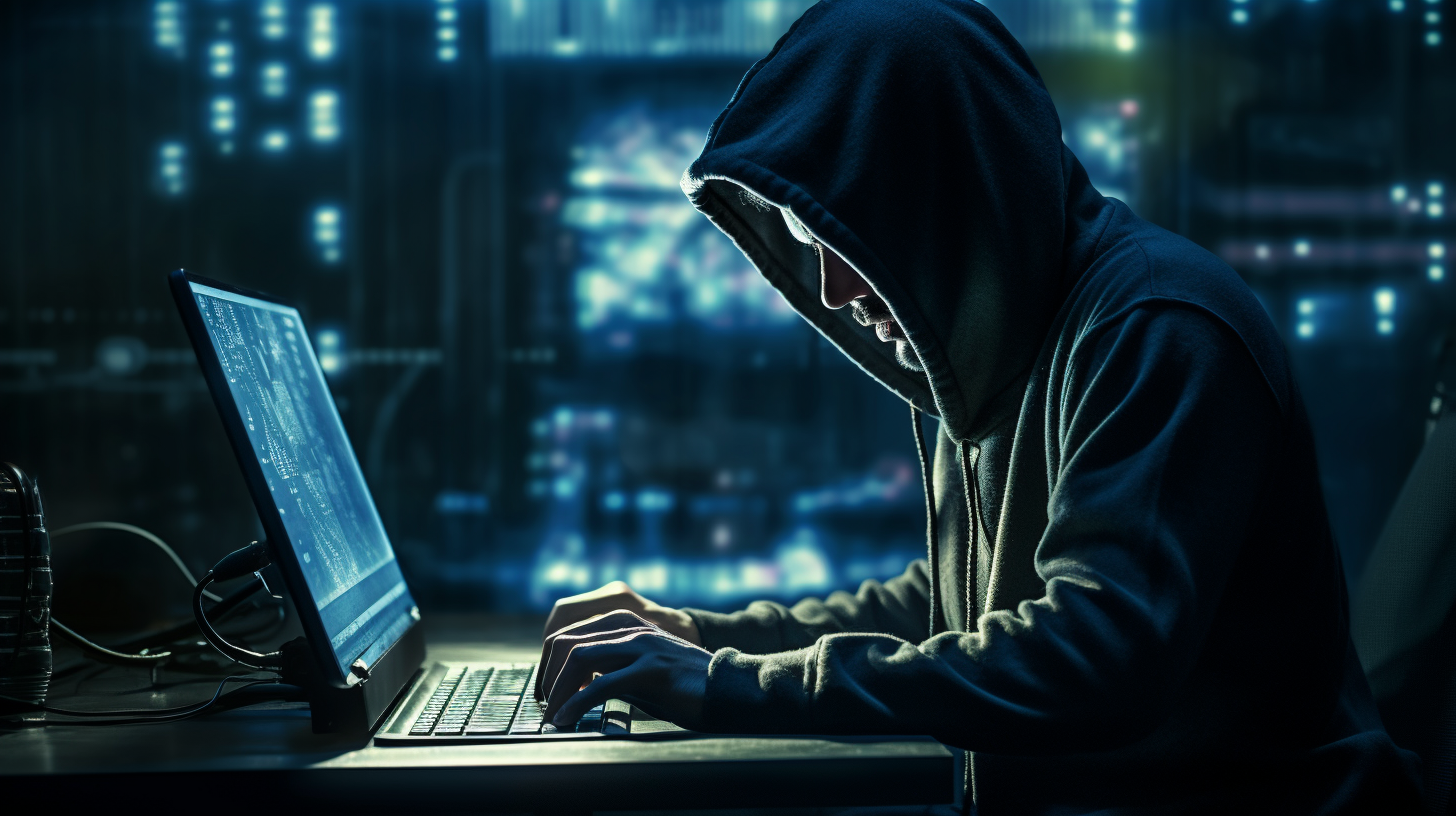
Introduction to JavaScript Callbacks
Understanding Promises in JavaScript
As we delve into the world of asynchronous JavaScript, it’s important to understand the role of Promises. A Promise in JavaScript is an object that represents the eventual completion (or failure) of an asynchronous operation, and its resulting value. Unlike callbacks, which require you to pass functions into other functions, Promises allow you to attach callbacks to the returned promise object.
In essence, a Promise is in one of these states:
- Pending: The initial state of a Promise. The operation has not completed yet.
- Fulfilled: The operation has completed successfully, and the Promise now has a resolved value.
- Rejected: The operation has failed, and the Promise has a reason for the failure. This reason is usually an Error of some kind.
To create a Promise, you use the new Promise()
constructor which takes a function, known as the “executor function”, as an argument. This function takes two functions as parameters, commonly referred to as resolve
and reject
. The resolve
function is called when the operation completes successfully, and reject
is called when the operation fails.
const myPromise = new Promise((resolve, reject) => { // Asynchronous operation here if (/* operation is successful */) { resolve('Success!'); } else { reject('Failure!'); } });
Once a Promise is created, you can use its then
method to handle fulfilled promises, and the catch
method to handle rejections. The then
method takes two arguments: a callback for success (fulfillment), and an optional callback for failure (rejection). The catch
method only takes one argument: a rejection callback.
myPromise .then((value) => { // This will be executed if the promise is successfully resolved console.log(value); // 'Success!' }) .catch((error) => { // This will be executed if the promise is rejected console.log(error); // 'Failure!' });
It’s also worth noting that Promises are chainable. This means you can append multiple then
methods, and each one will receive the result of the previous one’s success callback. If any link in the chain rejects, control will be passed to the next catch
handler.
Note: Always remember to add a
catch
at the end of your promise chains to handle any uncaught errors.
Promises are a powerful tool in state-of-the-art JavaScript and are widely used in place of callbacks for better handling of asynchronous operations. They provide a cleaner, more manageable code structure, especially when dealing with multiple asynchronous calls that depend on each other.
In the next section, we’ll compare callbacks and promises side-by-side to better understand their differences and when to use each.
Comparing Callbacks and Promises
When working with asynchronous operations in JavaScript, developers have the choice between using callbacks or Promises. Both serve the same purpose of handling the result of an asynchronous operation, but they do so in different ways. Let’s compare the two to understand their differences and when one might be preferable over the other.
Callbacks are functions that are passed into another function as an argument and are invoked to handle the result of an asynchronous operation. They have been around in JavaScript for a long time and are the traditional way to handle asynchronous operations. However, callbacks can lead to what is known as “callback hell” or the “pyramid of doom”, where multiple nested callbacks create a complex and hard-to-maintain code structure.
function fetchData(callback) { // Asynchronous operation setTimeout(() => { callback('Data fetched'); }, 1000); } fetchData((data) => { console.log(data); // 'Data fetched' // Nested callback fetchData((moreData) => { console.log(moreData); // 'Data fetched' // Another nested callback fetchData((evenMoreData) => { console.log(evenMoreData); // 'Data fetched' // ... and so on }); }); });
Promises provide a more elegant solution to handle asynchronous operations by avoiding the need for multiple nested callbacks. They represent a value that may be available now, later, or never, and allow developers to attach handlers for an operation’s eventual success value or reason for failure. This leads to cleaner and more manageable code, especially when chaining multiple asynchronous operations together.
function fetchData() { // Return a new Promise return new Promise((resolve, reject) => { // Asynchronous operation setTimeout(() => { resolve('Data fetched'); }, 1000); }); } fetchData() .then((data) => { console.log(data); // 'Data fetched' return fetchData(); // Chaining another promise }) .then((moreData) => { console.log(moreData); // 'Data fetched' return fetchData(); // Chaining yet another promise }) .then((evenMoreData) => { console.log(evenMoreData); // 'Data fetched' // ... and so on }) .catch((error) => { console.error(error); // Handle any error that occurs during any of the above steps });
There are several key differences between callbacks and Promises:
- Composition: Promises allow for better composition of asynchronous operations through chaining.
- Error Handling: Promises have better error handling with the catch method, which can catch errors from any step in the chain of promises.
- State: Promises have a state (pending, fulfilled, or rejected), which provides more control over the flow of asynchronous operations.
- Value: Once a Promise is fulfilled or rejected, it holds onto its value or reason for rejection, which can be accessed anytime after.
While callbacks can be simpler for handling single asynchronous operations, Promises are generally more suitable for handling multiple, complex asynchronous operations due to their chainability and better error handling. As such, Promises have become an integral part of writing clean and efficient JavaScript code in modern web development.
Source: https://www.plcourses.com/javascript-callbacks-and-promises/